Introduction
Audio on the web has been fairly primitive up to this point and until
very recently has had to be delivered through plugins such as Flash and
QuickTime. The introduction of the audio
element in HTML5
is very important, allowing for basic streaming audio playback. But, it
is not powerful enough to handle more complex audio applications. For
sophisticated web-based games or interactive applications, another
solution is required. It is a goal of this specification to include the
capabilities found in modern game audio engines as well as some of the
mixing, processing, and filtering tasks that are found in modern
desktop audio production applications.
The APIs have been designed with a wide variety of use cases [webaudio-usecases] in mind. Ideally, it should be able to support any use case which could reasonably be implemented with an optimized C++ engine controlled via script and run in a browser. That said, modern desktop audio software can have very advanced capabilities, some of which would be difficult or impossible to build with this system. Apple’s Logic Audio is one such application which has support for external MIDI controllers, arbitrary plugin audio effects and synthesizers, highly optimized direct-to-disk audio file reading/writing, tightly integrated time-stretching, and so on. Nevertheless, the proposed system will be quite capable of supporting a large range of reasonably complex games and interactive applications, including musical ones. And it can be a very good complement to the more advanced graphics features offered by WebGL. The API has been designed so that more advanced capabilities can be added at a later time.
Features
The API supports these primary features:
-
Modular routing for simple or complex mixing/effect architectures.
-
High dynamic range, using 32-bit floats for internal processing.
-
Sample-accurate scheduled sound playback with low latency for musical applications requiring a very high degree of rhythmic precision such as drum machines and sequencers. This also includes the possibility of dynamic creation of effects.
-
Automation of audio parameters for envelopes, fade-ins / fade-outs, granular effects, filter sweeps, LFOs etc.
-
Flexible handling of channels in an audio stream, allowing them to be split and merged.
-
Processing of audio sources from an
audio
orvideo
media element
. -
Processing live audio input using a
MediaStream
fromgetUserMedia()
. -
Integration with WebRTC
-
Processing audio received from a remote peer using a
MediaStreamTrackAudioSourceNode
and [webrtc]. -
Sending a generated or processed audio stream to a remote peer using a
MediaStreamAudioDestinationNode
and [webrtc].
-
-
Audio stream synthesis and processing directly using scripts.
-
Spatialized audio supporting a wide range of 3D games and immersive environments:
-
Panning models: equalpower, HRTF, pass-through
-
Distance Attenuation
-
Sound Cones
-
Obstruction / Occlusion
-
Source / Listener based
-
-
A convolution engine for a wide range of linear effects, especially very high-quality room effects. Here are some examples of possible effects:
-
Small / large room
-
Cathedral
-
Concert hall
-
Cave
-
Tunnel
-
Hallway
-
Forest
-
Amphitheater
-
Sound of a distant room through a doorway
-
Extreme filters
-
Strange backwards effects
-
Extreme comb filter effects
-
-
Dynamics compression for overall control and sweetening of the mix
-
Efficient real-time time-domain and frequency-domain analysis / music visualizer support.
-
Efficient biquad filters for lowpass, highpass, and other common filters.
-
A Waveshaping effect for distortion and other non-linear effects
-
Oscillators
Modular Routing
Modular routing allows arbitrary connections between different AudioNode
objects. Each node can have inputs and/or outputs.
A source node has no inputs and a single output.
A destination node has one input and no outputs. Other nodes such as
filters can be placed between the source and destination nodes. The
developer doesn’t have to worry about low-level stream format
details when two objects are connected together; the right thing just happens.
For example, if a mono audio stream is connected to a
stereo input it should just mix to left and right channels appropriately.
In the simplest case, a single source can be routed directly to the output.
All routing occurs within an AudioContext
containing a single AudioDestinationNode
:
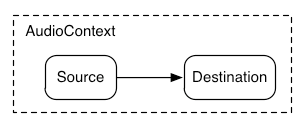
Illustrating this simple routing, here’s a simple example playing a single sound:
const context= new AudioContext(); function playSound() { const source= context. createBufferSource(); source. buffer= dogBarkingBuffer; source. connect( context. destination); source. start( 0 ); }
Here’s a more complex example with three sources and a convolution reverb send with a dynamics compressor at the final output stage:
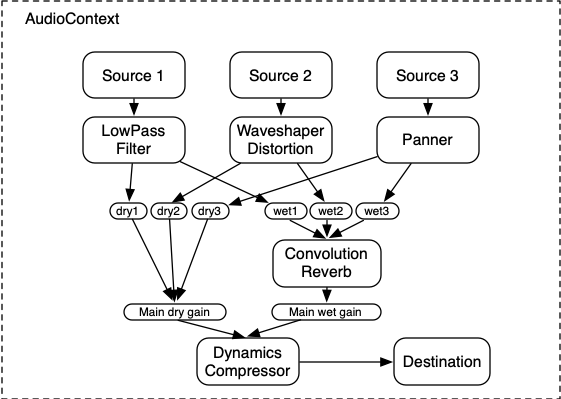
let context; let compressor; let reverb; let source1, source2, source3; let lowpassFilter; let waveShaper; let panner; let dry1, dry2, dry3; let wet1, wet2, wet3; let mainDry; let mainWet; function setupRoutingGraph() { context= new AudioContext(); // Create the effects nodes. lowpassFilter= context. createBiquadFilter(); waveShaper= context. createWaveShaper(); panner= context. createPanner(); compressor= context. createDynamicsCompressor(); reverb= context. createConvolver(); // Create main wet and dry. mainDry= context. createGain(); mainWet= context. createGain(); // Connect final compressor to final destination. compressor. connect( context. destination); // Connect main dry and wet to compressor. mainDry. connect( compressor); mainWet. connect( compressor); // Connect reverb to main wet. reverb. connect( mainWet); // Create a few sources. source1= context. createBufferSource(); source2= context. createBufferSource(); source3= context. createOscillator(); source1. buffer= manTalkingBuffer; source2. buffer= footstepsBuffer; source3. frequency. value= 440 ; // Connect source1 dry1= context. createGain(); wet1= context. createGain(); source1. connect( lowpassFilter); lowpassFilter. connect( dry1); lowpassFilter. connect( wet1); dry1. connect( mainDry); wet1. connect( reverb); // Connect source2 dry2= context. createGain(); wet2= context. createGain(); source2. connect( waveShaper); waveShaper. connect( dry2); waveShaper. connect( wet2); dry2. connect( mainDry); wet2. connect( reverb); // Connect source3 dry3= context. createGain(); wet3= context. createGain(); source3. connect( panner); panner. connect( dry3); panner. connect( wet3); dry3. connect( mainDry); wet3. connect( reverb); // Start the sources now. source1. start( 0 ); source2. start( 0 ); source3. start( 0 ); }
Modular routing also permits the output of AudioNode
s to be routed to an AudioParam
parameter that controls the behavior
of a different AudioNode
. In this scenario, the
output of a node can act as a modulation signal rather than an
input signal.
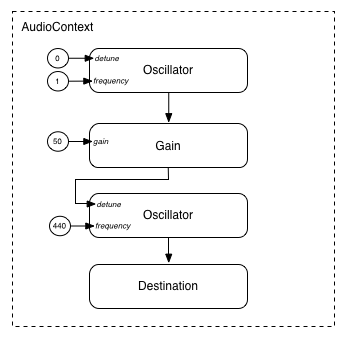
function setupRoutingGraph() { const context= new AudioContext(); // Create the low frequency oscillator that supplies the modulation signal const lfo= context. createOscillator(); lfo. frequency. value= 1.0 ; // Create the high frequency oscillator to be modulated const hfo= context. createOscillator(); hfo. frequency. value= 440.0 ; // Create a gain node whose gain determines the amplitude of the modulation signal const modulationGain= context. createGain(); modulationGain. gain. value= 50 ; // Configure the graph and start the oscillators lfo. connect( modulationGain); modulationGain. connect( hfo. detune); hfo. connect( context. destination); hfo. start( 0 ); lfo. start( 0 ); }
API Overview
The interfaces defined are:
-
An AudioContext interface, which contains an audio signal graph representing connections between
AudioNode
s. -
An
AudioNode
interface, which represents audio sources, audio outputs, and intermediate processing modules.AudioNode
s can be dynamically connected together in a modular fashion.AudioNode
s exist in the context of anAudioContext
. -
An
AnalyserNode
interface, anAudioNode
for use with music visualizers, or other visualization applications. -
An
AudioBuffer
interface, for working with memory-resident audio assets. These can represent one-shot sounds, or longer audio clips. -
An
AudioBufferSourceNode
interface, anAudioNode
which generates audio from an AudioBuffer. -
An
AudioDestinationNode
interface, anAudioNode
subclass representing the final destination for all rendered audio. -
An
AudioParam
interface, for controlling an individual aspect of anAudioNode
's functioning, such as volume. -
An
AudioListener
interface, which works with aPannerNode
for spatialization. -
An
AudioWorklet
interface representing a factory for creating custom nodes that can process audio directly using scripts. -
An
AudioWorkletGlobalScope
interface, the context in which AudioWorkletProcessor processing scripts run. -
An
AudioWorkletNode
interface, anAudioNode
representing a node processed in an AudioWorkletProcessor. -
An
AudioWorkletProcessor
interface, representing a single node instance inside an audio worker. -
A
BiquadFilterNode
interface, anAudioNode
for common low-order filters such as:-
Low Pass
-
High Pass
-
Band Pass
-
Low Shelf
-
High Shelf
-
Peaking
-
Notch
-
Allpass
-
-
A
ChannelMergerNode
interface, anAudioNode
for combining channels from multiple audio streams into a single audio stream. -
A
ChannelSplitterNode
interface, anAudioNode
for accessing the individual channels of an audio stream in the routing graph. -
A
ConstantSourceNode
interface, anAudioNode
for generating a nominally constant output value with anAudioParam
to allow automation of the value. -
A
ConvolverNode
interface, anAudioNode
for applying a real-time linear effect (such as the sound of a concert hall). -
A
DelayNode
interface, anAudioNode
which applies a dynamically adjustable variable delay. -
A
DynamicsCompressorNode
interface, anAudioNode
for dynamics compression. -
A
GainNode
interface, anAudioNode
for explicit gain control. -
An
IIRFilterNode
interface, anAudioNode
for a general IIR filter. -
A
MediaElementAudioSourceNode
interface, anAudioNode
which is the audio source from anaudio
,video
, or other media element. -
A
MediaStreamAudioSourceNode
interface, anAudioNode
which is the audio source from aMediaStream
such as live audio input, or from a remote peer. -
A
MediaStreamTrackAudioSourceNode
interface, anAudioNode
which is the audio source from aMediaStreamTrack
. -
A
MediaStreamAudioDestinationNode
interface, anAudioNode
which is the audio destination to aMediaStream
sent to a remote peer. -
A
PannerNode
interface, anAudioNode
for spatializing / positioning audio in 3D space. -
A
PeriodicWave
interface for specifying custom periodic waveforms for use by theOscillatorNode
. -
An
OscillatorNode
interface, anAudioNode
for generating a periodic waveform. -
A
StereoPannerNode
interface, anAudioNode
for equal-power positioning of audio input in a stereo stream. -
A
WaveShaperNode
interface, anAudioNode
which applies a non-linear waveshaping effect for distortion and other more subtle warming effects.
There are also several features that have been deprecated from the Web Audio API but not yet removed, pending implementation experience of their replacements:
-
A
ScriptProcessorNode
interface, anAudioNode
for generating or processing audio directly using scripts. -
An
AudioProcessingEvent
interface, which is an event type used withScriptProcessorNode
objects.
1. The Audio API
1.1. The BaseAudioContext
Interface
This interface represents a set of AudioNode
objects and their connections. It allows for arbitrary routing of
signals to an AudioDestinationNode
. Nodes are
created from the context and are then connected together.
BaseAudioContext
is not instantiated directly,
but is instead extended by the concrete interfaces AudioContext
(for real-time rendering) and OfflineAudioContext
(for offline rendering).
BaseAudioContext
are created with an internal slot [[pending promises]]
that is an
initially empty ordered list of promises.
Each BaseAudioContext
has a unique media element event task source.
Additionally, a BaseAudioContext
has several private slots [[rendering thread state]]
and [[control thread state]]
that take values from AudioContextState
, and that are both
"suspended"
. "suspended"
, and a private slot [[render quantum size]]
that is an unsigned integer.
enum {
AudioContextState "suspended" ,"running" ,"closed" };
Enum value | Description |
---|---|
"suspended "
| This context is currently suspended (context time is not proceeding, audio hardware may be powered down/released). |
"running "
| Audio is being processed. |
"closed "
| This context has been released, and can no longer be used to process audio. All system audio resources have been released. |
enum {
AudioContextRenderSizeCategory "default" ,"hardware" };
Enumeration description | |
---|---|
"default "
| The AudioContext’s render quantum size is the default value of 128 frames. |
"hardware "
|
The User-Agent picks a render quantum size that is best for the
current configuration.
Note: This exposes information about the host and can be used for fingerprinting. |
callback DecodeErrorCallback =undefined (DOMException error );callback DecodeSuccessCallback =undefined (AudioBuffer decodedData ); [Exposed =Window ]interface BaseAudioContext :EventTarget {readonly attribute AudioDestinationNode destination ;readonly attribute float sampleRate ;readonly attribute double currentTime ;readonly attribute AudioListener listener ;readonly attribute AudioContextState state ; [SameObject ,SecureContext ]readonly attribute AudioWorklet audioWorklet ;attribute EventHandler onstatechange ;AnalyserNode createAnalyser ();BiquadFilterNode createBiquadFilter ();AudioBuffer createBuffer (unsigned long numberOfChannels ,unsigned long length ,float sampleRate );AudioBufferSourceNode createBufferSource ();ChannelMergerNode createChannelMerger (optional unsigned long numberOfInputs = 6);ChannelSplitterNode createChannelSplitter (optional unsigned long numberOfOutputs = 6);ConstantSourceNode createConstantSource ();ConvolverNode createConvolver ();DelayNode createDelay (optional double maxDelayTime = 1.0);DynamicsCompressorNode createDynamicsCompressor ();GainNode createGain ();IIRFilterNode createIIRFilter (sequence <double >feedforward ,sequence <double >feedback );OscillatorNode createOscillator ();PannerNode createPanner ();PeriodicWave createPeriodicWave (sequence <float >real ,sequence <float >imag ,optional PeriodicWaveConstraints constraints = {});ScriptProcessorNode createScriptProcessor (optional unsigned long bufferSize = 0,optional unsigned long numberOfInputChannels = 2,optional unsigned long numberOfOutputChannels = 2);StereoPannerNode createStereoPanner ();WaveShaperNode createWaveShaper ();Promise <AudioBuffer >decodeAudioData (ArrayBuffer audioData ,optional DecodeSuccessCallback ?successCallback ,optional DecodeErrorCallback ?errorCallback ); };
callback DecodeErrorCallback =undefined (DOMException );
error callback DecodeSuccessCallback =undefined (AudioBuffer ); [
decodedData Exposed =Window ]interface BaseAudioContext :EventTarget {readonly attribute AudioDestinationNode destination ;readonly attribute float sampleRate ;readonly attribute double currentTime ;readonly attribute AudioListener listener ;readonly attribute AudioContextState state ;readonly attribute unsigned long renderQuantumSize ; [SameObject ,SecureContext ]readonly attribute AudioWorklet audioWorklet ;attribute EventHandler onstatechange ;AnalyserNode createAnalyser ();BiquadFilterNode createBiquadFilter ();AudioBuffer createBuffer (unsigned long ,
numberOfChannels unsigned long ,
length float );
sampleRate AudioBufferSourceNode createBufferSource ();ChannelMergerNode createChannelMerger (optional unsigned long numberOfInputs = 6);ChannelSplitterNode createChannelSplitter (optional unsigned long numberOfOutputs = 6);ConstantSourceNode createConstantSource ();ConvolverNode createConvolver ();DelayNode createDelay (optional double maxDelayTime = 1.0);DynamicsCompressorNode createDynamicsCompressor ();GainNode createGain ();IIRFilterNode createIIRFilter (sequence <double >,
feedforward sequence <double >);
feedback OscillatorNode createOscillator ();PannerNode createPanner ();PeriodicWave createPeriodicWave (sequence <float >,
real sequence <float >,
imag optional PeriodicWaveConstraints = {});
constraints ScriptProcessorNode createScriptProcessor (optional unsigned long bufferSize = 0,optional unsigned long numberOfInputChannels = 2,optional unsigned long numberOfOutputChannels = 2);StereoPannerNode createStereoPanner ();WaveShaperNode createWaveShaper ();Promise <AudioBuffer >decodeAudioData (ArrayBuffer ,
audioData optional DecodeSuccessCallback ?,
successCallback optional DecodeErrorCallback ?); };
errorCallback
1.1.1. Attributes
audioWorklet
, of type AudioWorklet, readonly-
Allows access to the
Worklet
object that can import a script containingAudioWorkletProcessor
class definitions via the algorithms defined by [HTML] andAudioWorklet
. currentTime
, of type double, readonly-
This is the time in seconds of the sample frame immediately following the last sample-frame in the block of audio most recently processed by the context’s rendering graph. If the context’s rendering graph has not yet processed a block of audio, then
currentTime
has a value of zero.In the time coordinate system of
currentTime
, the value of zero corresponds to the first sample-frame in the first block processed by the graph. Elapsed time in this system corresponds to elapsed time in the audio stream generated by theBaseAudioContext
, which may not be synchronized with other clocks in the system. (For anOfflineAudioContext
, since the stream is not being actively played by any device, there is not even an approximation to real time.)All scheduled times in the Web Audio API are relative to the value of
currentTime
.When the
BaseAudioContext
is in the "running
" state, the value of this attribute is monotonically increasing and is updated by the rendering thread in uniform increments, corresponding to one render quantum. Thus, for a running context,currentTime
increases steadily as the system processes audio blocks, and always represents the time of the start of the next audio block to be processed. It is also the earliest possible time when any change scheduled in the current state might take effect.currentTime
MUST be read atomically on the control thread before being returned. destination
, of type AudioDestinationNode, readonly-
An
AudioDestinationNode
with a single input representing the final destination for all audio. Usually this will represent the actual audio hardware. AllAudioNode
s actively rendering audio will directly or indirectly connect todestination
. listener
, of type AudioListener, readonly-
An
AudioListener
which is used for 3D spatialization. onstatechange
, of type EventHandler-
A property used to set an event handler for an event that is dispatched to
BaseAudioContext
when the state of the AudioContext has changed (i.e. when the corresponding promise would have resolved). The event type of this event handler isstatechange
. An event that uses theEvent
interface will be dispatched to the event handler, which can query the AudioContext’s state directly. A newly-created AudioContext will always begin in thesuspended
state, and a state change event will be fired whenever the state changes to a different state. This event is fired before thecomplete
event is fired. sampleRate
, of type float, readonly-
The sample rate (in sample-frames per second) at which the
BaseAudioContext
handles audio. It is assumed that allAudioNode
s in the context run at this rate. In making this assumption, sample-rate converters or "varispeed" processors are not supported in real-time processing. The Nyquist frequency is half this sample-rate value. state
, of type AudioContextState, readonly-
Describes the current state of the
BaseAudioContext
. Getting this attribute returns the contents of the[[control thread state]]
slot. renderQuantumSize
, of type unsigned long, readonly-
Getting this attribute returns the value of
[[render quantum size]]
slot.
1.1.2. Methods
createAnalyser()
-
Factory method for an
AnalyserNode
.No parameters.Return type:AnalyserNode
createBiquadFilter()
-
Factory method for a
BiquadFilterNode
representing a second order filter which can be configured as one of several common filter types.No parameters.Return type:BiquadFilterNode
createBuffer(numberOfChannels, length, sampleRate)
-
Creates an AudioBuffer of the given size. The audio data in the buffer will be zero-initialized (silent). A
NotSupportedError
exception MUST be thrown if any of the arguments is negative, zero, or outside its nominal range.Arguments for the BaseAudioContext.createBuffer() method. Parameter Type Nullable Optional Description numberOfChannels
unsigned long
✘ ✘ Determines how many channels the buffer will have. An implementation MUST support at least 32 channels. length
unsigned long
✘ ✘ Determines the size of the buffer in sample-frames. This MUST be at least 1. sampleRate
float
✘ ✘ Describes the sample-rate of the linear PCM audio data in the buffer in sample-frames per second. An implementation MUST support sample rates in at least the range 8000 to 96000. Return type:AudioBuffer
createBufferSource()
-
Factory method for a
AudioBufferSourceNode
.No parameters.Return type:AudioBufferSourceNode
createChannelMerger(numberOfInputs)
-
Factory method for a
ChannelMergerNode
representing a channel merger. AnIndexSizeError
exception MUST be thrown ifnumberOfInputs
is less than 1 or is greater than the number of supported channels.Arguments for the BaseAudioContext.createChannelMerger(numberOfInputs) method. Parameter Type Nullable Optional Description numberOfInputs
unsigned long
✘ ✔ Determines the number of inputs. Values of up to 32 MUST be supported. If not specified, then 6
will be used.Return type:ChannelMergerNode
createChannelSplitter(numberOfOutputs)
-
Factory method for a
ChannelSplitterNode
representing a channel splitter. AnIndexSizeError
exception MUST be thrown ifnumberOfOutputs
is less than 1 or is greater than the number of supported channels.Arguments for the BaseAudioContext.createChannelSplitter(numberOfOutputs) method. Parameter Type Nullable Optional Description numberOfOutputs
unsigned long
✘ ✔ The number of outputs. Values of up to 32 MUST be supported. If not specified, then 6
will be used.Return type:ChannelSplitterNode
createConstantSource()
-
Factory method for a
ConstantSourceNode
.No parameters.Return type:ConstantSourceNode
createConvolver()
-
Factory method for a
ConvolverNode
.No parameters.Return type:ConvolverNode
createDelay(maxDelayTime)
-
Factory method for a
DelayNode
. The initial default delay time will be 0 seconds.Arguments for the BaseAudioContext.createDelay(maxDelayTime) method. Parameter Type Nullable Optional Description maxDelayTime
double
✘ ✔ Specifies the maximum delay time in seconds allowed for the delay line. If specified, this value MUST be greater than zero and less than three minutes or a NotSupportedError
exception MUST be thrown. If not specified, then1
will be used.Return type:DelayNode
createDynamicsCompressor()
-
Factory method for a
DynamicsCompressorNode
.No parameters.Return type:DynamicsCompressorNode
createGain()
-
Factory method for
GainNode
.No parameters.Return type:GainNode
createIIRFilter(feedforward, feedback)
-
Arguments for the BaseAudioContext.createIIRFilter() method. Parameter Type Nullable Optional Description feedforward
sequence<double>
✘ ✘ An array of the feedforward (numerator) coefficients for the transfer function of the IIR filter. The maximum length of this array is 20. If all of the values are zero, an InvalidStateError
MUST be thrown. ANotSupportedError
MUST be thrown if the array length is 0 or greater than 20.feedback
sequence<double>
✘ ✘ An array of the feedback (denominator) coefficients for the transfer function of the IIR filter. The maximum length of this array is 20. If the first element of the array is 0, an InvalidStateError
MUST be thrown. ANotSupportedError
MUST be thrown if the array length is 0 or greater than 20.Return type:IIRFilterNode
createOscillator()
-
Factory method for an
OscillatorNode
.No parameters.Return type:OscillatorNode
createPanner()
-
Factory method for a
PannerNode
.No parameters.Return type:PannerNode
createPeriodicWave(real, imag, constraints)
-
Factory method to create a
PeriodicWave
.When calling this method, execute these steps:-
If
real
andimag
are not of the same length, anIndexSizeError
MUST be thrown. -
Let o be a new object of type
PeriodicWaveOptions
. -
Respectively set the
real
andimag
parameters passed to this factory method to the attributes of the same name on o. -
Set the
disableNormalization
attribute on o to the value of thedisableNormalization
attribute of theconstraints
attribute passed to the factory method. -
Construct a new
PeriodicWave
p, passing theBaseAudioContext
this factory method has been called on as a first argument, and o. -
Return p.
Arguments for the BaseAudioContext.createPeriodicWave() method. Parameter Type Nullable Optional Description real
sequence<float>
✘ ✘ A sequence of cosine parameters. See its real
constructor argument for a more detailed description.imag
sequence<float>
✘ ✘ A sequence of sine parameters. See its imag
constructor argument for a more detailed description.constraints
PeriodicWaveConstraints
✘ ✔ If not given, the waveform is normalized. Otherwise, the waveform is normalized according the value given by constraints
.Return type:PeriodicWave
-
createScriptProcessor(bufferSize, numberOfInputChannels, numberOfOutputChannels)
-
Factory method for a
ScriptProcessorNode
. This method is DEPRECATED, as it is intended to be replaced byAudioWorkletNode
. Creates aScriptProcessorNode
for direct audio processing using scripts. AnIndexSizeError
exception MUST be thrown ifbufferSize
ornumberOfInputChannels
ornumberOfOutputChannels
are outside the valid range.It is invalid for both
numberOfInputChannels
andnumberOfOutputChannels
to be zero. In this case anIndexSizeError
MUST be thrown.Arguments for the BaseAudioContext.createScriptProcessor(bufferSize, numberOfInputChannels, numberOfOutputChannels) method. Parameter Type Nullable Optional Description bufferSize
unsigned long
✘ ✔ The bufferSize
parameter determines the buffer size in units of sample-frames. If it’s not passed in, or if the value is 0, then the implementation will choose the best buffer size for the given environment, which will be constant power of 2 throughout the lifetime of the node. Otherwise if the author explicitly specifies the bufferSize, it MUST be one of the following values: 256, 512, 1024, 2048, 4096, 8192, 16384. This value controls how frequently theaudioprocess
event is dispatched and how many sample-frames need to be processed each call. Lower values forbufferSize
will result in a lower (better) latency. Higher values will be necessary to avoid audio breakup and glitches. It is recommended for authors to not specify this buffer size and allow the implementation to pick a good buffer size to balance between latency and audio quality. If the value of this parameter is not one of the allowed power-of-2 values listed above, anIndexSizeError
MUST be thrown.numberOfInputChannels
unsigned long
✘ ✔ This parameter determines the number of channels for this node’s input. The default value is 2. Values of up to 32 must be supported. A NotSupportedError
must be thrown if the number of channels is not supported.numberOfOutputChannels
unsigned long
✘ ✔ This parameter determines the number of channels for this node’s output. The default value is 2. Values of up to 32 must be supported. A NotSupportedError
must be thrown if the number of channels is not supported.Return type:ScriptProcessorNode
createStereoPanner()
-
Factory method for a
StereoPannerNode
.No parameters.Return type:StereoPannerNode
createWaveShaper()
-
Factory method for a
WaveShaperNode
representing a non-linear distortion.No parameters.Return type:WaveShaperNode
decodeAudioData(audioData, successCallback, errorCallback)
-
Asynchronously decodes the audio file data contained in the
ArrayBuffer
. TheArrayBuffer
can, for example, be loaded from anXMLHttpRequest
’sresponse
attribute after setting theresponseType
to"arraybuffer"
. Audio file data can be in any of the formats supported by theaudio
element. The buffer passed todecodeAudioData()
has its content-type determined by sniffing, as described in [mimesniff].Although the primary method of interfacing with this function is via its promise return value, the callback parameters are provided for legacy reasons.
Candidate Correction Issue 2321: Encourage implementation to warn authors in case of a corrupted file. It isn’t possible to throw because this would be a breaking change.Note: If the compressed audio data byte-stream is corrupted but the decoding can otherwise proceed, implementations are encouraged to warn authors for example via the developer tools.WhendecodeAudioData
is called, the following steps MUST be performed on the control thread:-
If this's relevant global object's associated Document is not fully active then return a promise rejected with "
InvalidStateError
"DOMException
. -
Let promise be a new Promise.
-
Proposed Correction Issue 2361-1. Use new Web IDL buffer primitives If
the operationIsDetachedBuffer
(described in [ECMASCRIPT]) onaudioData
isfalse
,audioData
is detached, execute the following steps:-
Append promise to
[[pending promises]]
. -
Proposed Correction Issue 2361-2. Use new Web IDL buffer primitives
DetachDetach theaudioData
ArrayBuffer
.This operation is described in [ECMASCRIPT].If this operations throws, jump to the step 3. -
Queue a decoding operation to be performed on another thread.
-
-
Else, execute the following error steps:
-
Let error be a
DataCloneError
. -
Reject promise with error, and remove it from
[[pending promises]]
. -
Queue a media element task to invoke
errorCallback
with error.
-
-
Return promise.
When queuing a decoding operation to be performed on another thread, the following steps MUST happen on a thread that is not the control thread nor the rendering thread, called thedecoding thread
.Note: Multiple
decoding thread
s can run in parallel to service multiple calls todecodeAudioData
.-
Let can decode be a boolean flag, initially set to true.
-
Attempt to determine the MIME type of
audioData
, using MIME Sniffing § 6.2 Matching an audio or video type pattern. If the audio or video type pattern matching algorithm returnsundefined
, set can decode to false. -
If can decode is true, attempt to decode the encoded
audioData
into linear PCM. In case of failure, set can decode to false.Proposed Correction Issue 2375. Only decode the first audio track of a multi-track media file. If the media byte-stream contains multiple audio tracks, only decode the first track to linear pcm.Note: Authors who need more control over the decoding process can use [WEBCODECS].
-
If can decode is
false
, queue a media element task to execute the following steps:-
Let error be a
DOMException
whose name isEncodingError
.-
Reject promise with error, and remove it from
[[pending promises]]
.
-
-
If
errorCallback
is not missing, invokeerrorCallback
with error.
-
-
Otherwise:
-
Take the result, representing the decoded linear PCM audio data, and resample it to the sample-rate of the
BaseAudioContext
if it is different from the sample-rate ofaudioData
. -
queue a media element task to execute the following steps:
-
Let buffer be an
AudioBuffer
containing the final result (after possibly performing sample-rate conversion). -
Resolve promise with buffer.
-
If
successCallback
is not missing, invokesuccessCallback
with buffer.
-
-
Arguments for the BaseAudioContext.decodeAudioData() method. Parameter Type Nullable Optional Description audioData
ArrayBuffer
✘ ✘ An ArrayBuffer containing compressed audio data. successCallback
DecodeSuccessCallback?
✔ ✔ A callback function which will be invoked when the decoding is finished. The single argument to this callback is an AudioBuffer representing the decoded PCM audio data. errorCallback
DecodeErrorCallback?
✔ ✔ A callback function which will be invoked if there is an error decoding the audio file. Return type:Promise
<AudioBuffer
> -
1.1.3. Callback DecodeSuccessCallback()
Parameters
decodedData
, of typeAudioBuffer
-
The AudioBuffer containing the decoded audio data.
1.1.4. Callback DecodeErrorCallback()
Parameters
error
, of typeDOMException
-
The error that occurred while decoding.
1.1.5. Lifetime
Once created, an AudioContext
will continue to play
sound until it has no more sound to play, or the page goes away.
1.1.6. Lack of Introspection or Serialization Primitives
The Web Audio API takes a fire-and-forget approach to
audio source scheduling. That is, source nodes are created
for each note during the lifetime of the AudioContext
, and
never explicitly removed from the graph. This is incompatible with
a serialization API, since there is no stable set of nodes that
could be serialized.
Moreover, having an introspection API would allow content script to be able to observe garbage collections.
1.1.7. System Resources Associated with BaseAudioContext
Subclasses
The subclasses AudioContext
and OfflineAudioContext
should be considered expensive objects. Creating these objects may
involve creating a high-priority thread, or using a low-latency
system audio stream, both having an impact on energy consumption.
It is usually not necessary to create more than one AudioContext
in a document.
Constructing or resuming a BaseAudioContext
subclass
involves acquiring system resources for
that context. For AudioContext
, this also requires creation
of a system audio stream. These operations return when the context
begins generating output from its associated audio graph.
Additionally, a user-agent can have an implementation-defined
maximum number of AudioContext
s, after which any attempt to
create a new AudioContext
will fail, throwing NotSupportedError
.
suspend
and close
allow authors to release system resources, including threads,
processes and audio streams. Suspending a BaseAudioContext
permits implementations to release some of its resources, and
allows it to continue to operate later by invoking resume
. Closing an AudioContext
permits implementations to release all of its
resources, after which it cannot be used or resumed again.
Note: For example, this can involve waiting for the audio callbacks to fire regularly, or to wait for the hardware to be ready for processing.
1.2. The AudioContext
Interface
This interface represents an audio graph whose AudioDestinationNode
is routed to a real-time
output device that produces a signal directed at the user. In most
use cases, only a single AudioContext
is used per
document.
enum {
AudioContextLatencyCategory "balanced" ,"interactive" ,"playback" };
Enum value | Description |
---|---|
"balanced "
| Balance audio output latency and power consumption. |
"interactive "
| Provide the lowest audio output latency possible without glitching. This is the default. |
"playback "
| Prioritize sustained playback without interruption over audio output latency. Lowest power consumption. |
Issue 2400 Access to a different output device
enum {
AudioSinkType "none" };
Enum Value | Description |
---|---|
"none "
| The audio graph will be processed without being played through an audio output device. |
Issue 2444 Add AudioRenderCapacity Interface
Issue 2400 Access to a different output device
[Exposed =Window ]interface AudioContext :BaseAudioContext {constructor (optional AudioContextOptions contextOptions = {});readonly attribute double baseLatency ;readonly attribute double outputLatency ;AudioTimestamp getOutputTimestamp ();Promise <undefined >resume ();Promise <undefined >suspend ();Promise <undefined >close ();MediaElementAudioSourceNode createMediaElementSource (HTMLMediaElement mediaElement );MediaStreamAudioSourceNode createMediaStreamSource (MediaStream mediaStream );MediaStreamTrackAudioSourceNode createMediaStreamTrackSource (MediaStreamTrack mediaStreamTrack );MediaStreamAudioDestinationNode createMediaStreamDestination (); };
[Exposed =Window ]interface AudioContext :BaseAudioContext {constructor (optional AudioContextOptions contextOptions = {});readonly attribute double baseLatency ;readonly attribute double outputLatency ; [SecureContext ]readonly attribute (DOMString or AudioSinkInfo )sinkId ; [SecureContext ]readonly attribute AudioRenderCapacity renderCapacity ;attribute EventHandler onsinkchange ;AudioTimestamp getOutputTimestamp ();Promise <undefined >resume ();Promise <undefined >suspend ();Promise <undefined >close (); [SecureContext ]Promise <undefined >((
setSinkId DOMString or AudioSinkOptions ));
sinkId MediaElementAudioSourceNode createMediaElementSource (HTMLMediaElement );
mediaElement MediaStreamAudioSourceNode createMediaStreamSource (MediaStream );
mediaStream MediaStreamTrackAudioSourceNode createMediaStreamTrackSource (MediaStreamTrack );
mediaStreamTrack MediaStreamAudioDestinationNode createMediaStreamDestination (); };
An AudioContext
is said to be allowed to start if the user agent
allows the context state to transition from "suspended
" to
"running
". A user agent may disallow this initial transition,
and to allow it only when the AudioContext
's relevant global object has sticky activation.
AudioContext
has following internal slots:
[[suspended by user]]
-
A boolean flag representing whether the context is suspended by user code. The initial value is
false
. [[sink ID]]
-
A
DOMString
or anAudioSinkInfo
representing the identifier or the information of the current audio output device respectively. The initial value is""
, which means the default audio output device. [[pending resume promises]]
-
An ordered list to store pending
Promise
s created byresume()
. It is initially empty.
Issue 2400 Access to a different output device
1.2.1. Constructors
AudioContext(contextOptions)
-
If the current settings object's relevant global object's associated Document is NOT fully active, throw an "
When creating anInvalidStateError
" and abort these steps.AudioContext
, execute these steps:Proposed Addition
Issue 2456 Add a MessagePort to the AudioWorkletGlobalScope
Issue 2400 Access to a different output device
Issue 2450 Allow specifying a different render-quantum-size for an AudioContext or OfflineAudioContext-
Set a
[[control thread state]]
tosuspended
on theAudioContext
. -
Set a
[[rendering thread state]]
tosuspended
on theAudioContext
. -
Set a private slot called
[[render quantum size]]
to128
on theAudioContext
. -
Let
[[pending resume promises]]
be a slot on thisAudioContext
, that is an initially empty ordered list of promises. -
If
contextOptions
is given, apply the options: -
Set the internal latency of this
AudioContext
according tocontextOptions.
, as described inlatencyHint
latencyHint
. -
If
contextOptions.
is specified, execute the following steps:renderSizeHint
-
If equal to
"hardware"
, the User-Agent can set[[render quantum size]]
to a value that’s best for the current setup. -
If an integer has been passed, the User-Agent can set
[[render quantum size]]
to this integer.
-
-
If
contextOptions.
is specified, set thesampleRate
sampleRate
of thisAudioContext
to this value. Otherwise, use the sample rate of the default output device. If the selected sample rate differs from the sample rate of the output device, thisAudioContext
MUST resample the audio output to match the sample rate of the output device.Note: If resampling is required, the latency of the AudioContext may be affected, possibly by a large amount.
-
If the context is allowed to start, send a control message to start processing.
-
Return this
AudioContext
object.
-
Let context be a new
AudioContext
object. -
Set a
[[control thread state]]
tosuspended
on context. -
Set a
[[rendering thread state]]
tosuspended
on context. -
Let messageChannel be a new
MessageChannel
. -
Let controlSidePort be the value of messageChannel’s
port1
attribute. -
Let renderingSidePort be the value of messageChannel’s
port2
attribute. -
Let serializedRenderingSidePort be the result of StructuredSerializeWithTransfer(renderingSidePort, « renderingSidePort »).
-
Set this
audioWorklet
'sport
to controlSidePort. -
Queue a control message to set the MessagePort on the AudioContextGlobalScope, with serializedRenderingSidePort.
-
If
contextOptions
is given, perform the following substeps:-
If
sinkId
is specified, let sinkId be the value ofcontextOptions.
and run the following substeps:sinkId
-
If both sinkId and
[[sink ID]]
are a type ofDOMString
, and they are equal to each other, abort these substeps. -
If sinkId is a type of
AudioSinkOptions
and[[sink ID]]
is a type ofAudioSinkInfo
, andtype
in sinkId andtype
in[[sink ID]]
are equal, abort these substeps. -
Let validationResult be the return value of sink identifier validation of sinkId.
-
If validationResult is a type of
DOMException
, throw an exception with validationResult and abort these substeps. -
If sinkId is a type of
DOMString
, set[[sink ID]]
to sinkId and abort these substeps. -
If sinkId is a type of
AudioSinkOptions
, set[[sink ID]]
to a new instance ofAudioSinkInfo
created with the value oftype
of sinkId.
-
-
Set the internal latency of context according to
contextOptions.
, as described inlatencyHint
latencyHint
. -
If
contextOptions.
is specified, set thesampleRate
sampleRate
of context to this value. Otherwise, follow these substeps:-
If sinkId is the empty string or a type of
AudioSinkOptions
, use the sample rate of the default output device. Abort these substeps. -
If sinkId is a
DOMString
, use the sample rate of the output device identified by sinkId. Abort these substeps.
If
contextOptions.
differs from the sample rate of the output device, the user agent MUST resample the audio output to match the sample rate of the output device.sampleRate
Note: If resampling is required, the latency of context may be affected, possibly by a large amount.
-
-
-
If context is allowed to start, send a control message to start processing.
-
Return context.
-
-
Attempt to acquire system resources. In case of failure, abort the following steps.
-
Set the
[[rendering thread state]]
torunning
on theAudioContext
. -
queue a media element task to execute the following steps:
-
Set the
state
attribute of theAudioContext
to "running
". -
queue a media element task to fire an event named
statechange
at theAudioContext
.
-
-
Attempt to acquire system resources to use a following audio output device based on
[[sink ID]]
for rendering:-
The default audio output device for the empty string.
-
A audio output device identified by
[[sink ID]]
.
In case of failure, abort the following steps.
-
-
Set the
[[rendering thread state]]
torunning
on theAudioContext
. -
Queue a media element task to execute the following steps:
-
Set the
state
attribute of theAudioContext
to "running
". -
Fire an event named
statechange
at theAudioContext
.
-
-
Let deserializedPort be the result of StructuredDeserialize(serializedRenderingSidePort, the current Realm).
-
Set
port
to deserializedPort.
Issue 2400 Access to a different output device
AudioContext
failed. User-Agents are
encouraged to log an informative message if they have access to a logging
mechanism, such as a developer tools console.
Sending a control message to set the MessagePort
on the AudioWorkletGlobalScope
means executing the following steps, on
the rendering thread, with serializedRenderingSidePort, that has been transfered to
the AudioWorkletGlobalScope
:
Parameter | Type | Nullable | Optional | Description |
---|---|---|---|---|
contextOptions
| AudioContextOptions
| ✘ | ✔ | User-specified options controlling how the AudioContext should be constructed.
|
1.2.2. Attributes
baseLatency
, of type double, readonly-
This represents the number of seconds of processing latency incurred by the
AudioContext
passing the audio from theAudioDestinationNode
to the audio subsystem. It does not include any additional latency that might be caused by any other processing between the output of theAudioDestinationNode
and the audio hardware and specifically does not include any latency incurred the audio graph itself. outputLatency
, of type double, readonly-
The estimation in seconds of audio output latency, i.e., the interval between the time the UA requests the host system to play a buffer and the time at which the first sample in the buffer is actually processed by the audio output device. For devices such as speakers or headphones that produce an acoustic signal, this latter time refers to the time when a sample’s sound is produced.
An
outputLatency
attribute value depends on the platform and the connected audio output device hardware. TheoutputLatency
attribute value may change while the context is running or the associated audio output device changes. It is useful to query this value frequently when accurate synchronization is required. renderCapacity
, of type AudioRenderCapacity, readonly-
Returns an
AudioRenderCapacity
instance associated with anAudioContext
. sinkId
, of type(DOMString or AudioSinkInfo)
, readonly-
Returns the value of
[[sink ID]]
internal slot. This attribute is cached upon update, and it returns the same object after caching. onsinkchange
, of type EventHandler-
An event handler for
setSinkId()
. The event type of this event handler issinkchange
. This event will be dispatched when changing the output device is completed.NOTE: This is not dispatched for the initial device selection in the construction of
AudioContext
. Thestatechange
event is available to check the readiness of the initial output device.
AudioDestinationNode
implements double buffering
internally and can process and output audio each render
quantum, then the processing latency is \((2\cdot128)/44100
= 5.805 \mathrm{ ms}\), approximately. AudioDestinationNode
implements double buffering
internally and can process and output audio each render quantum, then
the processing latency is \((2\cdot128)/44100 = 5.805 \mathrm{ ms}\),
approximately.
Issue 2400 Access to a different output device
1.2.3. Methods
close()
-
Closes the
AudioContext
, releasing the system resources being used. This will not automatically release allAudioContext
-created objects, but will suspend the progression of theAudioContext
'scurrentTime
, and stop processing audio data.When close is called, execute these steps:-
If this's relevant global object's associated Document is not fully active then return a promise rejected with "
InvalidStateError
"DOMException
. -
Let promise be a new Promise.
-
If the
[[control thread state]]
flag on theAudioContext
isclosed
reject the promise withInvalidStateError
, abort these steps, returning promise. -
Set the
[[control thread state]]
flag on theAudioContext
toclosed
. -
Queue a control message to close the
AudioContext
. -
Return promise.
Running a control message to close anAudioContext
means running these steps on the rendering thread:-
Attempt to release system resources.
-
Set the
[[rendering thread state]]
tosuspended
.This will stop rendering. -
If this control message is being run in a reaction to the document being unloaded, abort this algorithm.
There is no need to notify the control thread in this case. -
queue a media element task to execute the following steps:
-
Resolve promise.
-
If the
state
attribute of theAudioContext
is not already "closed
":-
Set the
state
attribute of theAudioContext
to "closed
". -
queue a media element task to fire an event named
statechange
at theAudioContext
.
-
-
When an
AudioContext
is closed, anyMediaStream
s andHTMLMediaElement
s that were connected to anAudioContext
will have their output ignored. That is, these will no longer cause any output to speakers or other output devices. For more flexibility in behavior, consider usingHTMLMediaElement.captureStream()
.Note: When an
AudioContext
has been closed, implementation can choose to aggressively release more resources than when suspending.No parameters. -
createMediaElementSource(mediaElement)
-
Creates a
MediaElementAudioSourceNode
given anHTMLMediaElement
. As a consequence of calling this method, audio playback from theHTMLMediaElement
will be re-routed into the processing graph of theAudioContext
.Arguments for the AudioContext.createMediaElementSource() method. Parameter Type Nullable Optional Description mediaElement
HTMLMediaElement
✘ ✘ The media element that will be re-routed. Return type:MediaElementAudioSourceNode
createMediaStreamDestination()
-
Creates a
MediaStreamAudioDestinationNode
No parameters.Return type:MediaStreamAudioDestinationNode
createMediaStreamSource(mediaStream)
-
Creates a
MediaStreamAudioSourceNode
.Arguments for the AudioContext.createMediaStreamSource() method. Parameter Type Nullable Optional Description mediaStream
MediaStream
✘ ✘ The media stream that will act as source. Return type:MediaStreamAudioSourceNode
createMediaStreamTrackSource(mediaStreamTrack)
-
Creates a
MediaStreamTrackAudioSourceNode
.Arguments for the AudioContext.createMediaStreamTrackSource() method. Parameter Type Nullable Optional Description mediaStreamTrack
MediaStreamTrack
✘ ✘ The MediaStreamTrack
that will act as source. The value of itskind
attribute must be equal to"audio"
, or anInvalidStateError
exception MUST be thrown.Return type:MediaStreamTrackAudioSourceNode
getOutputTimestamp()
-
Returns a new
AudioTimestamp
instance containing two related audio stream position values for the context: thecontextTime
member contains the time of the sample frame which is currently being rendered by the audio output device (i.e., output audio stream position), in the same units and origin as context’scurrentTime
; theperformanceTime
member contains the time estimating the moment when the sample frame corresponding to the storedcontextTime
value was rendered by the audio output device, in the same units and origin asperformance.now()
(described in [hr-time-3]).If the context’s rendering graph has not yet processed a block of audio, then
getOutputTimestamp
call returns anAudioTimestamp
instance with both members containing zero.After the context’s rendering graph has started processing of blocks of audio, its
currentTime
attribute value always exceeds thecontextTime
value obtained fromgetOutputTimestamp
method call.The value returned fromgetOutputTimestamp
method can be used to get performance time estimation for the slightly later context’s time value:function outputPerformanceTime( contextTime) { const timestamp= context. getOutputTimestamp(); const elapsedTime= contextTime- timestamp. contextTime; return timestamp. performanceTime+ elapsedTime* 1000 ; } In the above example the accuracy of the estimation depends on how close the argument value is to the current output audio stream position: the closer the given
contextTime
is totimestamp.contextTime
, the better the accuracy of the obtained estimation.Note: The difference between the values of the context’s
currentTime
and thecontextTime
obtained fromgetOutputTimestamp
method call cannot be considered as a reliable output latency estimation becausecurrentTime
may be incremented at non-uniform time intervals, sooutputLatency
attribute should be used instead.No parameters.Return type:AudioTimestamp
resume()
-
Resumes the progression of the
AudioContext
'scurrentTime
when it has been suspended.When resume is called, execute these steps:-
If this's relevant global object's associated Document is not fully active then return a promise rejected with "
InvalidStateError
"DOMException
. -
Let promise be a new Promise.
-
If the
[[control thread state]]
on theAudioContext
isclosed
reject the promise withInvalidStateError
, abort these steps, returning promise. -
Set
[[suspended by user]]
tofalse
. -
If the context is not allowed to start, append promise to
[[pending promises]]
and[[pending resume promises]]
and abort these steps, returning promise. -
Set the
[[control thread state]]
on theAudioContext
torunning
. -
Queue a control message to resume the
AudioContext
. -
Return promise.
Running a control message to resume anAudioContext
means running these steps on the rendering thread:-
Attempt to acquire system resources.
-
Set the
[[rendering thread state]]
on theAudioContext
torunning
. -
Start rendering the audio graph.
-
In case of failure, queue a media element task to execute the following steps:
-
Reject all promises from
[[pending resume promises]]
in order, then clear[[pending resume promises]]
. -
Additionally, remove those promises from
[[pending promises]]
.
-
-
queue a media element task to execute the following steps:
-
Resolve all promises from
[[pending resume promises]]
in order. -
Clear
[[pending resume promises]]
. Additionally, remove those promises from[[pending promises]]
. -
Resolve promise.
-
If the
state
attribute of theAudioContext
is not already "running
":-
Set the
state
attribute of theAudioContext
to "running
". -
queue a media element task to fire an event named
statechange
at theAudioContext
.
-
-
No parameters. -
suspend()
-
Suspends the progression of
AudioContext
'scurrentTime
, allows any current context processing blocks that are already processed to be played to the destination, and then allows the system to release its claim on audio hardware. This is generally useful when the application knows it will not need theAudioContext
for some time, and wishes to temporarily release system resource associated with theAudioContext
. The promise resolves when the frame buffer is empty (has been handed off to the hardware), or immediately (with no other effect) if the context is alreadysuspended
. The promise is rejected if the context has been closed.When suspend is called, execute these steps:-
If this's relevant global object's associated Document is not fully active then return a promise rejected with "
InvalidStateError
"DOMException
. -
Let promise be a new Promise.
-
If the
[[control thread state]]
on theAudioContext
isclosed
reject the promise withInvalidStateError
, abort these steps, returning promise. -
Append promise to
[[pending promises]]
. -
Set
[[suspended by user]]
totrue
. -
Set the
[[control thread state]]
on theAudioContext
tosuspended
. -
Queue a control message to suspend the
AudioContext
. -
Return promise.
Running a control message to suspend anAudioContext
means running these steps on the rendering thread:-
Attempt to release system resources.
-
Set the
[[rendering thread state]]
on theAudioContext
tosuspended
. -
queue a media element task to execute the following steps:
-
Resolve promise.
-
If the
state
attribute of theAudioContext
is not already "suspended
":-
Set the
state
attribute of theAudioContext
to "suspended
". -
queue a media element task to fire an event named
statechange
at theAudioContext
.
-
-
While an
AudioContext
is suspended,MediaStream
s will have their output ignored; that is, data will be lost by the real time nature of media streams.HTMLMediaElement
s will similarly have their output ignored until the system is resumed.AudioWorkletNode
s andScriptProcessorNode
s will cease to have their processing handlers invoked while suspended, but will resume when the context is resumed. For the purpose ofAnalyserNode
window functions, the data is considered as a continuous stream - i.e. theresume()
/suspend()
does not cause silence to appear in theAnalyserNode
's stream of data. In particular, callingAnalyserNode
functions repeatedly when aAudioContext
is suspended MUST return the same data.No parameters. -
setSinkId((DOMString or AudioSinkOptions) sinkId)
-
Sets the identifier of an output device. When this method is invoked, the user agent MUST run the following steps:
-
Let sinkId be the method’s first argument.
-
If sinkId is equal to
[[sink ID]]
, return a promise, resolve it immediately and abort these steps. -
Let validationResult be the return value of sink identifier validation of sinkId.
-
If validationResult is not
null
, return a promise rejected with validationResult. Abort these steps. -
Let p be a new promise.
-
Send a control message with p and sinkId to start processing.
-
Return p.
Sending a control message to start processing duringsetSinkId()
means executing the following steps:-
Let p be the promise passed into this algorithm.
-
Let sinkId be the sink identifier passed into this algorithm.
-
If both sinkId and
[[sink ID]]
are a type ofDOMString
, and they are equal to each other, queue a media element task to resolve p and abort these steps. -
If sinkId is a type of
AudioSinkOptions
and[[sink ID]]
is a type ofAudioSinkInfo
, andtype
in sinkId andtype
in[[sink ID]]
are equal, queue a media element task to resolve p and abort these steps. -
Let wasRunning be true.
-
Set wasRunning to false if the
[[rendering thread state]]
on theAudioContext
is"suspended"
. -
Pause the renderer after processing the current render quantum.
-
Attempt to release system resources.
-
If wasRunning is true:
-
Set the
[[rendering thread state]]
on theAudioContext
to"suspended"
. -
Queue a media element task to execute the following steps:
-
If the
state
attribute of theAudioContext
is not already "suspended
":-
Set the
state
attribute of theAudioContext
to "suspended
". -
Fire an event named
statechange
at the associatedAudioContext
.
-
-
-
-
Attempt to acquire system resources to use a following audio output device based on
[[sink ID]]
for rendering:-
The default audio output device for the empty string.
-
A audio output device identified by
[[sink ID]]
.
In case of failure, reject p with "
InvalidAccessError
" abort the following steps. -
-
Queue a media element task to execute the following steps:
-
If sinkId is a type of
DOMString
, set[[sink ID]]
to sinkId. Abort these steps. -
If sinkId is a type of
AudioSinkOptions
and[[sink ID]]
is a type ofDOMString
, set[[sink ID]]
to a new instance ofAudioSinkInfo
created with the value oftype
of sinkId. -
If sinkId is a type of
AudioSinkOptions
and[[sink ID]]
is a type ofAudioSinkInfo
, settype
of[[sink ID]]
to thetype
value of sinkId. -
Resolve p.
-
Fire an event named
sinkchange
at the associatedAudioContext
.
-
-
If wasRunning is true:
-
Set the
[[rendering thread state]]
on theAudioContext
to"running"
. -
Queue a media element task to execute the following steps:
-
If the
state
attribute of theAudioContext
is not already "running
":-
Set the
state
attribute of theAudioContext
to "running
". -
Fire an event named
statechange
at the associatedAudioContext
.
-
-
-
-
Issue 2400 Access to a different output device
Issue 2400 Access to a different output device
1.2.4. Validating sinkId
This algorithm is used to validate the information provided to modify sinkId
:
-
Let document be the current settings object’s associated Document.
-
Let sinkIdArg be the value passed in to this algorithm.
-
If document is not allowed to use the feature identified by
"speaker-selection"
, return a newDOMException
whose name is "NotAllowedError
". -
If sinkIdArg is a type of
DOMString
but it is not equal to the empty string or it does not match any audio output device identified by the result that would be provided byenumerateDevices()
, return a newDOMException
whose name is "NotFoundError
". -
Return
null
.
1.2.5. AudioContextOptions
The AudioContextOptions
dictionary is used to
specify user-specified options for an AudioContext
.
Issue 2400 Access to a different output device
Issue 2450 Allow specifying a different render quantum size
dictionary AudioContextOptions { (AudioContextLatencyCategory or double )latencyHint = "interactive";float sampleRate ; };
dictionary AudioContextOptions { (AudioContextLatencyCategory or double )latencyHint = "interactive";float sampleRate ; (DOMString or AudioSinkOptions )sinkId ; (AudioContextRenderSizeCategory or unsigned long )renderSizeHint = "default"; };
1.2.5.1. Dictionary AudioContextOptions
Members
latencyHint
, of type(AudioContextLatencyCategory or double)
, defaulting to"interactive"
-
Identify the type of playback, which affects tradeoffs between audio output latency and power consumption.
The preferred value of the
latencyHint
is a value fromAudioContextLatencyCategory
. However, a double can also be specified for the number of seconds of latency for finer control to balance latency and power consumption. It is at the browser’s discretion to interpret the number appropriately. The actual latency used is given by AudioContext’sbaseLatency
attribute. sampleRate
, of type float-
Set the
sampleRate
to this value for theAudioContext
that will be created. The supported values are the same as the sample rates for anAudioBuffer
. ANotSupportedError
exception MUST be thrown if the specified sample rate is not supported.If
sampleRate
is not specified, the preferred sample rate of the output device for thisAudioContext
is used. sinkId
, of type(DOMString or AudioSinkOptions)
-
The identifier or associated information of the audio output device. See
sinkId
for more details. renderSizeHint
, of type(AudioContextRenderSizeCategory or unsigned long)
, defaulting to"default"
-
This allows users to ask for a particular render quantum size when an integer is passed, to use the default of 128 frames if nothing or
"default"
is passed, or to ask the User-Agent to pick a good render quantum size if"hardware"
is specified.It is a hint that might not be honored.
Issue 2400 Access to a different output device
Issue 2400 Access to a different output device
1.2.6. AudioSinkOptions
The AudioSinkOptions
dictionary is used to specify options for sinkId
.
dictionary AudioSinkOptions {required AudioSinkType type ; };
1.2.6.1. Dictionary AudioSinkOptions
Members
type
, of type AudioSinkType-
A value of
AudioSinkType
to specify the type of the device.
1.2.7. AudioSinkInfo
The AudioSinkInfo
interface is used to get information on the current
audio output device via sinkId
.
[Exposed =Window ]interface AudioSinkInfo {readonly attribute AudioSinkType type ; };
1.2.7.1. Attributes
type
, of type AudioSinkType, readonly-
A value of
AudioSinkType
that represents the type of the device.
1.2.8. AudioTimestamp
dictionary AudioTimestamp {double contextTime ;DOMHighResTimeStamp performanceTime ; };
1.2.8.1. Dictionary AudioTimestamp
Members
contextTime
, of type double-
Represents a point in the time coordinate system of BaseAudioContext’s
currentTime
. performanceTime
, of type DOMHighResTimeStamp-
Represents a point in the time coordinate system of a
Performance
interface implementation (described in [hr-time-3]).
1.2.9. AudioRenderCapacity
[Exposed =Window ]interface :
AudioRenderCapacity EventTarget {undefined start (optional AudioRenderCapacityOptions = {});
options undefined stop ();attribute EventHandler onupdate ; };
This interface provides rendering performance metrics of an AudioContext
. In order to calculate them, the renderer collects a load value per system-level audio callback.
1.2.9.1. Attributes
onupdate
, of type EventHandler-
The event type of this event handler is
update
. Events dispatched to the event handler will use theAudioRenderCapacityEvent
interface.
1.2.9.2. Methods
start(options)
-
Starts metric collection and analysis. This will repeatedly fire an event named
update
atAudioRenderCapacity
, usingAudioRenderCapacityEvent
, with the given update interval inAudioRenderCapacityOptions
. stop()
-
Stops metric collection and analysis. It also stops dispatching
update
events.
1.2.10. AudioRenderCapacityOptions
The AudioRenderCapacityOptions
dictionary can be used to provide user
options for an AudioRenderCapacity
.
dictionary {
AudioRenderCapacityOptions double updateInterval = 1; };
1.2.10.1. Dictionary AudioRenderCapacityOptions
Members
updateInterval
, of type double, defaulting to1
-
An update interval (in second) for dispaching
AudioRenderCapacityEvent
s. A load value is calculated per system-level audio callback, and multiple load values will be collected over the specified interval period. For example, if the renderer runs at a 48Khz sample rate and the system-level audio callback’s buffer size is 192 frames, 250 load values will be collected over 1 second interval.If the given value is smaller than the duration of the system-level audio callback,
NotSupportedError
is thrown.
1.2.11. AudioRenderCapacityEvent
[Exposed =Window ]interface :
AudioRenderCapacityEvent Event {(
constructor DOMString ,
type optional AudioRenderCapacityEventInit = {});
eventInitDict readonly attribute double timestamp ;readonly attribute double averageLoad ;readonly attribute double peakLoad ;readonly attribute double underrunRatio ; };dictionary :
AudioRenderCapacityEventInit EventInit {double = 0;
timestamp double = 0;
averageLoad double = 0;
peakLoad double = 0; };
underrunRatio
1.2.11.1. Attributes
timestamp
, of type double, readonly-
The start time of the data collection period in terms of the associated
AudioContext
'scurrentTime
. averageLoad
, of type double, readonly-
An average of collected load values over the given update interval. The precision is limited to 1/100th.
peakLoad
, of type double, readonly-
A maximum value from collected load values over the given update interval. The precision is also limited to 1/100th.
underrunRatio
, of type double, readonly-
A ratio between the number of buffer underruns (when a load value is greater than 1.0) and the total number of system-level audio callbacks over the given update interval.
Where \(u\) is the number of buffer underruns and \(N\) is the number of system-level audio callbacks over the given update interval, the buffer underrun ratio is:
-
0.0 if \(u\) = 0.
-
Otherwise, compute \(u/N\) and take a ceiling value of the nearest 100th.
-
1.3. The OfflineAudioContext
Interface
OfflineAudioContext
is a particular type of BaseAudioContext
for rendering/mixing-down
(potentially) faster than real-time. It does not render to the audio
hardware, but instead renders as quickly as possible, fulfilling the
returned promise with the rendered result as an AudioBuffer
.
[Exposed =Window ]interface OfflineAudioContext :BaseAudioContext {constructor (OfflineAudioContextOptions contextOptions );constructor (unsigned long numberOfChannels ,unsigned long length ,float sampleRate );Promise <AudioBuffer >startRendering ();Promise <undefined >resume ();Promise <undefined >suspend (double );
suspendTime readonly attribute unsigned long length ;attribute EventHandler oncomplete ; };
1.3.1. Constructors
OfflineAudioContext(contextOptions)
-
Proposed Addition Issue 2456. Add a MessagePort to the AudioWorkletGlobalScope
Issue 2450. Allow specifying a different render quantum sizeIf the current settings object's relevant global object's associated Document is NOT fully active, throw an
Let c be a newInvalidStateError
and abort these steps.OfflineAudioContext
object. Initialize c as follows:-
Set the
[[control thread state]]
for c to"suspended"
. -
Set the
[[rendering thread state]]
for c to"suspended"
. -
Construct an
AudioDestinationNode
with itschannelCount
set tocontextOptions.numberOfChannels
.
If the current settings object's relevant global object's associated Document is NOT fully active, throw an
Let c be a newInvalidStateError
and abort these steps.OfflineAudioContext
object. Initialize c as follows:-
Set the
[[control thread state]]
for c to"suspended"
. -
Set the
[[rendering thread state]]
for c to"suspended"
. -
Determine the
[[render quantum size]]
for thisOfflineAudioContext
, based on the value of therenderSizeHint
:-
If it has the default value of
"default"
or"hardware
", set the[[render quantum size]]
private slot to 128. -
Else, if an integer has been passed, the User-Agent can decide to honour this value by setting it to the
[[render quantum size]]
private slot.
-
-
Construct an
AudioDestinationNode
with itschannelCount
set tocontextOptions.numberOfChannels
. -
Let messageChannel be a new
MessageChannel
. -
Let controlSidePort be the value of messageChannel’s
port1
attribute. -
Let renderingSidePort be the value of messageChannel’s
port2
attribute. -
Let serializedRenderingSidePort be the result of StructuredSerializeWithTransfer(renderingSidePort, « renderingSidePort »).
-
Set this
audioWorklet
'sport
to controlSidePort. -
Queue a control message to set the MessagePort on the AudioContextGlobalScope, with serializedRenderingSidePort.
Arguments for the OfflineAudioContext.constructor(contextOptions) method. Parameter Type Nullable Optional Description contextOptions
The initial parameters needed to construct this context. -
OfflineAudioContext(numberOfChannels, length, sampleRate)
-
The
OfflineAudioContext
can be constructed with the same arguments as AudioContext.createBuffer. ANotSupportedError
exception MUST be thrown if any of the arguments is negative, zero, or outside its nominal range.The OfflineAudioContext is constructed as if
new OfflineAudioContext({ numberOfChannels: numberOfChannels, length: length, sampleRate: sampleRate}) were called instead.
Arguments for the OfflineAudioContext.constructor(numberOfChannels, length, sampleRate) method. Parameter Type Nullable Optional Description numberOfChannels
unsigned long
✘ ✘ Determines how many channels the buffer will have. See createBuffer()
for the supported number of channels.length
unsigned long
✘ ✘ Determines the size of the buffer in sample-frames. sampleRate
float
✘ ✘ Describes the sample-rate of the linear PCM audio data in the buffer in sample-frames per second. See createBuffer()
for valid sample rates.
1.3.2. Attributes
length
, of type unsigned long, readonly-
The size of the buffer in sample-frames. This is the same as the value of the
length
parameter for the constructor. oncomplete
, of type EventHandler-
The event type of this event handler is
complete
. The event dispatched to the event handler will use theOfflineAudioCompletionEvent
interface. It is the last event fired on anOfflineAudioContext
.
1.3.3. Methods
startRendering()
-
Given the current connections and scheduled changes, starts rendering audio.
Although the primary method of getting the rendered audio data is via its promise return value, the instance will also fire an event named
complete
for legacy reasons.Let[[rendering started]]
be an internal slot of thisOfflineAudioContext
. Initialize this slot to false.When
startRendering
is called, the following steps MUST be performed on the control thread:- If this's relevant global object's associated Document is not fully active then return a promise rejected with "
InvalidStateError
"DOMException
. - If the
[[rendering started]]
slot on theOfflineAudioContext
is true, return a rejected promise withInvalidStateError
, and abort these steps. - Set the
[[rendering started]]
slot of theOfflineAudioContext
to true. - Let promise be a new promise.
- Create a new
AudioBuffer
, with a number of channels, length and sample rate equal respectively to thenumberOfChannels
,length
andsampleRate
values passed to this instance’s constructor in thecontextOptions
parameter. Assign this buffer to an internal slot[[rendered buffer]]
in theOfflineAudioContext
. - If an exception was thrown during the preceding
AudioBuffer
constructor call, reject promise with this exception. - Otherwise, in the case that the buffer was successfully constructed, begin offline rendering.
- Append promise to
[[pending promises]]
. - Return promise.
To begin offline rendering, the following steps MUST happen on a rendering thread that is created for the occasion.- Given the current connections and scheduled changes, start
rendering
length
sample-frames of audio into[[rendered buffer]]
- For every render quantum, check and
suspend
rendering if necessary. - If a suspended context is resumed, continue to render the buffer.
-
Once the rendering is complete, queue a media element task to execute the following steps:
- Resolve the promise created by
startRendering()
with[[rendered buffer]]
. - queue a media element task to fire an event named
complete
using an instance ofOfflineAudioCompletionEvent
whoserenderedBuffer
property is set to[[rendered buffer]]
.
- Resolve the promise created by
No parameters.Return type:Promise
<AudioBuffer
> - If this's relevant global object's associated Document is not fully active then return a promise rejected with "
resume()
-
Resumes the progression of the
OfflineAudioContext
'scurrentTime
when it has been suspended.When resume is called, execute these steps:-
If this's relevant global object's associated Document is not fully active then return a promise rejected with "
InvalidStateError
"DOMException
. -
Let promise be a new Promise.
-
Abort these steps and reject promise with
InvalidStateError
when any of following conditions is true:-
The
[[control thread state]]
on theOfflineAudioContext
isclosed
. -
The
[[rendering started]]
slot on theOfflineAudioContext
is false.
-
-
Set the
[[control thread state]]
flag on theOfflineAudioContext
torunning
. -
Queue a control message to resume the
OfflineAudioContext
. -
Return promise.
Running a control message to resume anOfflineAudioContext
means running these steps on the rendering thread:-
Set the
[[rendering thread state]]
on theOfflineAudioContext
torunning
. -
Start rendering the audio graph.
-
In case of failure, queue a media element task to reject promise and abort the remaining steps.
-
queue a media element task to execute the following steps:
-
Resolve promise.
-
If the
state
attribute of theOfflineAudioContext
is not already "running
":-
Set the
state
attribute of theOfflineAudioContext
to "running
". -
queue a media element task to fire an event named
statechange
at theOfflineAudioContext
.
-
-
No parameters. -
suspend(suspendTime)
-
Schedules a suspension of the time progression in the audio context at the specified time and returns a promise. This is generally useful when manipulating the audio graph synchronously on
OfflineAudioContext
.Note that the maximum precision of suspension is the size of the render quantum and the specified suspension time will be rounded up to the nearest render quantum boundary. For this reason, it is not allowed to schedule multiple suspends at the same quantized frame. Also, scheduling should be done while the context is not running to ensure precise suspension.
Arguments for the OfflineAudioContext.suspend() method. Parameter Type Nullable Optional Description suspendTime
double
✘ ✘ Schedules a suspension of the rendering at the specified time, which is quantized and rounded up to the render quantum size. If the quantized frame number - is negative or
- is less than or equal to the current time or
- is greater than or equal to the total render duration or
- is scheduled by another suspend for the same time,
InvalidStateError
.
1.3.4. OfflineAudioContextOptions
This specifies the options to use in constructing an OfflineAudioContext
.
dictionary OfflineAudioContextOptions {unsigned long numberOfChannels = 1;required unsigned long length ;required float sampleRate ; };
dictionary OfflineAudioContextOptions {unsigned long numberOfChannels = 1;required unsigned long length ;required float sampleRate ; (AudioContextRenderSizeCategory or unsigned long )renderSizeHint = "default"; };
1.3.4.1. Dictionary OfflineAudioContextOptions
Members
length
, of type unsigned long-
The length of the rendered
AudioBuffer
in sample-frames. numberOfChannels
, of type unsigned long, defaulting to1
-
The number of channels for this
OfflineAudioContext
. sampleRate
, of type float-
The sample rate for this
OfflineAudioContext
. renderSizeHint
, of type(AudioContextRenderSizeCategory or unsigned long)
, defaulting to"default"
-
A hint for the render quantum size of this
OfflineAudioContext
.
1.3.5. The OfflineAudioCompletionEvent
Interface
This is an Event
object which is dispatched to OfflineAudioContext
for legacy reasons.
[Exposed =Window ]interface OfflineAudioCompletionEvent :Event {(
constructor DOMString ,
type OfflineAudioCompletionEventInit );
eventInitDict readonly attribute AudioBuffer renderedBuffer ; };
1.3.5.1. Attributes
renderedBuffer
, of type AudioBuffer, readonly-
An
AudioBuffer
containing the rendered audio data.
1.3.5.2. OfflineAudioCompletionEventInit
dictionary OfflineAudioCompletionEventInit :EventInit {required AudioBuffer renderedBuffer ; };
1.3.5.2.1. Dictionary OfflineAudioCompletionEventInit
Members
renderedBuffer
, of type AudioBuffer-
Value to be assigned to the
renderedBuffer
attribute of the event.
1.4. The AudioBuffer
Interface
This interface represents a memory-resident audio asset. It can contain one or
more channels with each channel appearing to be 32-bit floating-point linear PCM values with a nominal range of \([-1,1]\) but the
values are not limited to this range. Typically, it would be expected
that the length of the
PCM data would be fairly short (usually somewhat less than a minute).
For longer sounds, such as music soundtracks, streaming should be
used with the audio
element and MediaElementAudioSourceNode
.
An AudioBuffer
may be used by one or more AudioContext
s, and can be shared between an OfflineAudioContext
and an AudioContext
.
AudioBuffer
has four internal slots:
[[number of channels]]
-
The number of audio channels for this
AudioBuffer
, which is an unsigned long. [[length]]
-
The length of each channel of this
AudioBuffer
, which is an unsigned long. [[sample rate]]
-
The sample-rate, in Hz, of this
AudioBuffer
, a float. [[internal data]]
-
A data block holding the audio sample data.
[Exposed =Window ]interface AudioBuffer {constructor (AudioBufferOptions );
options readonly attribute float sampleRate ;readonly attribute unsigned long length ;readonly attribute double duration ;readonly attribute unsigned long numberOfChannels ;Float32Array getChannelData (unsigned long );
channel undefined copyFromChannel (Float32Array ,
destination unsigned long ,
channelNumber optional unsigned long = 0);
bufferOffset undefined copyToChannel (Float32Array ,
source unsigned long ,
channelNumber optional unsigned long = 0); };
bufferOffset
1.4.1. Constructors
AudioBuffer(options)
-
-
If any of the values in
options
lie outside its nominal range, throw aNotSupportedError
exception and abort the following steps. -
Let b be a new
AudioBuffer
object. -
Respectively assign the values of the attributes
numberOfChannels
,length
,sampleRate
of theAudioBufferOptions
passed in the constructor to the internal slots[[number of channels]]
,[[length]]
,[[sample rate]]
. -
Set the internal slot
[[internal data]]
of thisAudioBuffer
to the result of callingCreateByteDataBlock
(
.[[length]]
*[[number of channels]]
)Note: This initializes the underlying storage to zero.
-
Return b.
Arguments for the AudioBuffer.constructor() method. Parameter Type Nullable Optional Description options
AudioBufferOptions
✘ ✘ An AudioBufferOptions
that determine the properties for thisAudioBuffer
. -
1.4.2. Attributes
duration
, of type double, readonly-
Duration of the PCM audio data in seconds.
This is computed from the
[[sample rate]]
and the[[length]]
of theAudioBuffer
by performing a division between the[[length]]
and the[[sample rate]]
. length
, of type unsigned long, readonly-
Length of the PCM audio data in sample-frames. This MUST return the value of
[[length]]
. numberOfChannels
, of type unsigned long, readonly-
The number of discrete audio channels. This MUST return the value of
[[number of channels]]
. sampleRate
, of type float, readonly-
The sample-rate for the PCM audio data in samples per second. This MUST return the value of
[[sample rate]]
.
1.4.3. Methods
copyFromChannel(destination, channelNumber, bufferOffset)
-
The
copyFromChannel()
method copies the samples from the specified channel of theAudioBuffer
to thedestination
array.Let
buffer
be theAudioBuffer
with \(N_b\) frames, let \(N_f\) be the number of elements in thedestination
array, and \(k\) be the value ofbufferOffset
. Then the number of frames copied frombuffer
todestination
is \(\max(0, \min(N_b - k, N_f))\). If this is less than \(N_f\), then the remaining elements ofdestination
are not modified.Arguments for the AudioBuffer.copyFromChannel() method. Parameter Type Nullable Optional Description destination
Float32Array
✘ ✘ The array the channel data will be copied to. channelNumber
unsigned long
✘ ✘ The index of the channel to copy the data from. If channelNumber
is greater or equal than the number of channels of theAudioBuffer
, anIndexSizeError
MUST be thrown.bufferOffset
unsigned long
✘ ✔ An optional offset, defaulting to 0. Data from the AudioBuffer
starting at this offset is copied to thedestination
.Return type:undefined
copyToChannel(source, channelNumber, bufferOffset)
-
The
copyToChannel()
method copies the samples to the specified channel of theAudioBuffer
from thesource
array.A
UnknownError
may be thrown ifsource
cannot be copied to the buffer.Let
buffer
be theAudioBuffer
with \(N_b\) frames, let \(N_f\) be the number of elements in thesource
array, and \(k\) be the value ofbufferOffset
. Then the number of frames copied fromsource
to thebuffer
is \(\max(0, \min(N_b - k, N_f))\). If this is less than \(N_f\), then the remaining elements ofbuffer
are not modified.Arguments for the AudioBuffer.copyToChannel() method. Parameter Type Nullable Optional Description source
Float32Array
✘ ✘ The array the channel data will be copied from. channelNumber
unsigned long
✘ ✘ The index of the channel to copy the data to. If channelNumber
is greater or equal than the number of channels of theAudioBuffer
, anIndexSizeError
MUST be thrown.bufferOffset
unsigned long
✘ ✔ An optional offset, defaulting to 0. Data from the source
is copied to theAudioBuffer
starting at this offset.Return type:undefined
getChannelData(channel)
-
Proposed Correction Issue 2361-3. Use new Web IDL buffer primitives According to the rules described in acquire the content either
get a reference toallow writing into or getting a copy of the bytes stored in[[internal data]]
in a newFloat32Array
A
UnknownError
may be thrown if the[[internal data]]
or the newFloat32Array
cannot be created.Arguments for the AudioBuffer.getChannelData() method. Parameter Type Nullable Optional Description channel
unsigned long
✘ ✘ This parameter is an index representing the particular channel to get data for. An index value of 0 represents the first channel. This index value MUST be less than [[number of channels]]
or anIndexSizeError
exception MUST be thrown.Return type:Float32Array
Note: The methods copyToChannel()
and copyFromChannel()
can be used to fill part of an array by
passing in a Float32Array
that’s a view onto the larger
array. When reading data from an AudioBuffer
's channels, and
the data can be processed in chunks, copyFromChannel()
should be preferred to calling getChannelData()
and
accessing the resulting array, because it may avoid unnecessary
memory allocation and copying.
An internal operation acquire the
contents of an AudioBuffer is invoked when the
contents of an AudioBuffer
are needed by some API
implementation. This operation returns immutable channel data to the
invoker.
AudioBuffer
, run the following steps:
-
Proposed Correction Issue 2361-4. Use new Web IDL buffer primitives If
the operationany of theIsDetachedBuffer
on any of theAudioBuffer
'sArrayBuffer
sAudioBuffer
'sArrayBuffer
s are detached , returntrue
, abort these steps, and return a zero-length channel data buffer to the invoker. -
Proposed Correction Issue 2361-5. Use new Web IDL buffer primitives
DetachDetach allArrayBuffer
s for arrays previously returned bygetChannelData()
on thisAudioBuffer
.Note: Because
AudioBuffer
can only be created viacreateBuffer()
or via theAudioBuffer
constructor, this cannot throw. -
Retain the underlying
[[internal data]]
from thoseArrayBuffer
s and return references to them to the invoker. -
Attach
ArrayBuffer
s containing copies of the data to theAudioBuffer
, to be returned by the next call togetChannelData()
.
The acquire the contents of an AudioBuffer operation is invoked in the following cases:
-
When
AudioBufferSourceNode.start
is called, it acquires the contents of the node’sbuffer
. If the operation fails, nothing is played. -
When the
buffer
of anAudioBufferSourceNode
is set andAudioBufferSourceNode.start
has been previously called, the setter acquires the content of theAudioBuffer
. If the operation fails, nothing is played. -
When a
ConvolverNode
'sbuffer
is set to anAudioBuffer
it acquires the content of theAudioBuffer
. -
When the dispatch of an
AudioProcessingEvent
completes, it acquires the contents of itsoutputBuffer
.
Note: This means that copyToChannel()
cannot be used to change
the content of an AudioBuffer
currently in use by an AudioNode
that has acquired the content of an AudioBuffer since the AudioNode
will continue to use the data previously
acquired.
1.4.4. AudioBufferOptions
This specifies the options to use in constructing an AudioBuffer
. The length
and sampleRate
members are
required.
dictionary AudioBufferOptions {unsigned long numberOfChannels = 1;required unsigned long length ;required float sampleRate ; };
1.4.4.1. Dictionary AudioBufferOptions
Members
The allowed values for the members of this dictionary are constrained. See createBuffer()
.
length
, of type unsigned long-
The length in sample frames of the buffer. See
length
for constraints. numberOfChannels
, of type unsigned long, defaulting to1
-
The number of channels for the buffer. See
numberOfChannels
for constraints. sampleRate
, of type float-
The sample rate in Hz for the buffer. See
sampleRate
for constraints.
1.5. The AudioNode
Interface
AudioNode
s are the building blocks of an AudioContext
. This interface
represents audio sources, the audio destination, and intermediate
processing modules. These modules can be connected together to form processing graphs for rendering audio
to the audio hardware. Each node can have inputs and/or outputs. A source node has no inputs and a single
output. Most processing nodes such as filters will have one input and
one output. Each type of AudioNode
differs in the
details of how it processes or synthesizes audio. But, in general, an AudioNode
will process its inputs (if it has
any), and generate audio for its outputs (if it has any).
Each output has one or more channels. The exact number of channels
depends on the details of the specific AudioNode
.
An output may connect to one or more AudioNode
inputs, thus fan-out is supported. An input initially has no
connections, but may be connected from one or more AudioNode
outputs, thus fan-in is supported. When the connect()
method is called to connect an output of an AudioNode
to an input of an AudioNode
, we call that a connection to the input.
Each AudioNode
input has a specific number of
channels at any given time. This number can change depending on the connection(s) made to the input. If the input has no
connections then it has one channel which is silent.
For each input, an AudioNode
performs a
mixing of all connections to that input.
Please see § 4 Channel Up-Mixing and Down-Mixing for normative requirements and details.
The processing of inputs and the internal operations of an AudioNode
take place continuously with respect to AudioContext
time, regardless of whether the node has
connected outputs, and regardless of whether these outputs ultimately
reach an AudioContext
's AudioDestinationNode
.
[Exposed =Window ]interface AudioNode :EventTarget {AudioNode connect (AudioNode destinationNode ,optional unsigned long output = 0,optional unsigned long input = 0);undefined connect (AudioParam destinationParam ,optional unsigned long output = 0);undefined disconnect ();undefined disconnect (unsigned long output );undefined disconnect (AudioNode destinationNode );undefined disconnect (AudioNode destinationNode ,unsigned long output );undefined disconnect (AudioNode destinationNode ,unsigned long output ,unsigned long input );undefined disconnect (AudioParam destinationParam );undefined disconnect (AudioParam destinationParam ,unsigned long output );readonly attribute BaseAudioContext context ;readonly attribute unsigned long numberOfInputs ;readonly attribute unsigned long numberOfOutputs ;attribute unsigned long channelCount ;attribute ChannelCountMode channelCountMode ;attribute ChannelInterpretation channelInterpretation ; };
1.5.1. AudioNode Creation
AudioNode
s can be created in two ways: by using the
constructor for this particular interface, or by using the factory method on the BaseAudioContext
or AudioContext
.
The BaseAudioContext
passed as first argument of the
constructor of an AudioNode
s is called the associated BaseAudioContext
of the AudioNode
to be created. Similarly, when using the factory
method, the associated BaseAudioContext
of the AudioNode
is the BaseAudioContext
this factory method
is called on.
AudioNode
of a particular type n using its factory method, called on a BaseAudioContext
c, execute these steps:
-
Let node be a new object of type n.
-
Let option be a dictionary of the type associated to the interface associated to this factory method.
-
For each parameter passed to the factory method, set the dictionary member of the same name on option to the value of this parameter.
-
Call the constructor for n on node with c and option as arguments.
-
Return node
AudioNode
means executing the following
steps, given the arguments context and dict passed to
the constructor of this interface.
-
Set o’s associated
BaseAudioContext
to context. -
Set its value for
numberOfInputs
,numberOfOutputs
,channelCount
,channelCountMode
,channelInterpretation
to the default value for this specific interface outlined in the section for eachAudioNode
. -
For each member of dict passed in, execute these steps, with k the key of the member, and v its value. If any exceptions is thrown when executing these steps, abort the iteration and propagate the exception to the caller of the algorithm (constructor or factory method).
-
If k is the name of an
AudioParam
on this interface, set thevalue
attribute of thisAudioParam
to v. -
Else if k is the name of an attribute on this interface, set the object associated with this attribute to v.
-
The associated interface for a factory method is the interface of the objects that are returned from this method. The associated option object for an interface is the option object that can be passed to the constructor for this interface.
AudioNode
s are EventTarget
s, as described in [DOM].
This means that it is possible to dispatch events to AudioNode
s the same way that other EventTarget
s
accept events.
enum {
ChannelCountMode "max" ,"clamped-max" ,"explicit" };
The ChannelCountMode
, in conjuction with the node’s channelCount
and channelInterpretation
values, is used to determine
the computedNumberOfChannels that controls how inputs to a
node are to be mixed. The computedNumberOfChannels is
determined as shown below. See § 4 Channel Up-Mixing and Down-Mixing for more information on how
mixing is to be done.
Enum value | Description |
---|---|
"max "
| computedNumberOfChannels is the maximum of the number of
channels of all connections to an input. In this mode channelCount is ignored.
|
"clamped-max "
| computedNumberOfChannels is determined as for "max "
and then clamped to a maximum value of the given channelCount .
|
"explicit "
| computedNumberOfChannels is the exact value as specified
by the channelCount .
|
enum {
ChannelInterpretation "speakers" ,"discrete" };
Enum value | Description |
---|---|
"speakers "
| use up-mix equations or down-mix equations. In cases where the number of
channels do not match any of these basic speaker layouts, revert
to "discrete ".
|
"discrete "
| Up-mix by filling channels until they run out then zero out remaining channels. Down-mix by filling as many channels as possible, then dropping remaining channels. |
1.5.2. AudioNode Tail-Time
An AudioNode
can have a tail-time. This means that even when the AudioNode
is fed silence, the output can be non-silent.
AudioNode
s have a non-zero tail-time if they have internal processing state
such that input in the past affects the future output. AudioNode
s
may continue to produce non-silent output for the calculated tail-time even
after the input transitions from non-silent to silent.
1.5.3. AudioNode Lifetime
AudioNode
can be actively processing during a render quantum, if any of the following conditions hold.
-
An
AudioScheduledSourceNode
is actively processing if and only if it is playing for at least part of the current rendering quantum. -
A
MediaElementAudioSourceNode
is actively processing if and only if itsmediaElement
is playing for at least part of the current rendering quantum. -
A
MediaStreamAudioSourceNode
or aMediaStreamTrackAudioSourceNode
are actively processing when the associatedMediaStreamTrack
object has areadyState
attribute equal to"live"
, amuted
attribute equal tofalse
and anenabled
attribute equal totrue
. -
A
DelayNode
in a cycle is actively processing only when the absolute value of any output sample for the current render quantum is greater than or equal to \( 2^{-126} \). -
A
ScriptProcessorNode
is actively processing when its input or output is connected. -
An
AudioWorkletNode
is actively processing when itsAudioWorkletProcessor
's[[callable process]]
returnstrue
and either its active source flag istrue
or anyAudioNode
connected to one of its inputs is actively processing. -
All other
AudioNode
s start actively processing when anyAudioNode
connected to one of its inputs is actively processing, and stops actively processing when the input that was received from other actively processingAudioNode
no longer affects the output.
Note: This takes into account AudioNode
s that have a tail-time.
AudioNode
s that are not actively processing output a single channel of
silence.
1.5.4. Attributes
channelCount
, of type unsigned long-
channelCount
is the number of channels used when up-mixing and down-mixing connections to any inputs to the node. The default value is 2 except for specific nodes where its value is specially determined. This attribute has no effect for nodes with no inputs. If this value is set to zero or to a value greater than the implementation’s maximum number of channels the implementation MUST throw aNotSupportedError
exception.In addition, some nodes have additional channelCount constraints on the possible values for the channel count:
AudioDestinationNode
-
The behavior depends on whether the destination node is the destination of an
AudioContext
orOfflineAudioContext
:AudioContext
-
The channel count MUST be between 1 and
maxChannelCount
. AnIndexSizeError
exception MUST be thrown for any attempt to set the count outside this range. OfflineAudioContext
-
The channel count cannot be changed. An
InvalidStateError
exception MUST be thrown for any attempt to change the value.
AudioWorkletNode
-
See § 1.32.4.3.2 Configuring Channels with AudioWorkletNodeOptions Configuring Channels with AudioWorkletNodeOptions.
ChannelMergerNode
-
The channel count cannot be changed, and an
InvalidStateError
exception MUST be thrown for any attempt to change the value. ChannelSplitterNode
-
The channel count cannot be changed, and an
InvalidStateError
exception MUST be thrown for any attempt to change the value. ConvolverNode
-
The channel count cannot be greater than two, and a
NotSupportedError
exception MUST be thrown for any attempt to change it to a value greater than two. DynamicsCompressorNode
-
The channel count cannot be greater than two, and a
NotSupportedError
exception MUST be thrown for any attempt to change it to a value greater than two. PannerNode
-
The channel count cannot be greater than two, and a
NotSupportedError
exception MUST be thrown for any attempt to change it to a value greater than two. ScriptProcessorNode
-
The channel count cannot be changed, and an
NotSupportedError
exception MUST be thrown for any attempt to change the value. StereoPannerNode
-
The channel count cannot be greater than two, and a
NotSupportedError
exception MUST be thrown for any attempt to change it to a value greater than two.
See § 4 Channel Up-Mixing and Down-Mixing for more information on this attribute.
channelCountMode
, of type ChannelCountMode-
channelCountMode
determines how channels will be counted when up-mixing and down-mixing connections to any inputs to the node. The default value is "max
". This attribute has no effect for nodes with no inputs.In addition, some nodes have additional channelCountMode constraints on the possible values for the channel count mode:
AudioDestinationNode
-
If the
AudioDestinationNode
is thedestination
node of anOfflineAudioContext
, then the channel count mode cannot be changed. AnInvalidStateError
exception MUST be thrown for any attempt to change the value. ChannelMergerNode
-
The channel count mode cannot be changed from "
explicit
" and anInvalidStateError
exception MUST be thrown for any attempt to change the value. ChannelSplitterNode
-
The channel count mode cannot be changed from "
explicit
" and anInvalidStateError
exception MUST be thrown for any attempt to change the value. ConvolverNode
-
The channel count mode cannot be set to "
max
", and aNotSupportedError
exception MUST be thrown for any attempt to set it to "max
". DynamicsCompressorNode
-
The channel count mode cannot be set to "
max
", and aNotSupportedError
exception MUST be thrown for any attempt to set it to "max
". PannerNode
-
The channel count mode cannot be set to "
max
", and aNotSupportedError
exception MUST be thrown for any attempt to set it to "max
". ScriptProcessorNode
-
The channel count mode cannot be changed from "
explicit
" and anNotSupportedError
exception MUST be thrown for any attempt to change the value. StereoPannerNode
-
The channel count mode cannot be set to "
max
", and aNotSupportedError
exception MUST be thrown for any attempt to set it to "max
".
See the § 4 Channel Up-Mixing and Down-Mixing section for more information on this attribute.
channelInterpretation
, of type ChannelInterpretation-
channelInterpretation
determines how individual channels will be treated when up-mixing and down-mixing connections to any inputs to the node. The default value is "speakers
". This attribute has no effect for nodes with no inputs.In addition, some nodes have additional channelInterpretation constraints on the possible values for the channel interpretation:
ChannelSplitterNode
-
The channel intepretation can not be changed from "
discrete
" and aInvalidStateError
exception MUST be thrown for any attempt to change the value.
See § 4 Channel Up-Mixing and Down-Mixing for more information on this attribute.
context
, of type BaseAudioContext, readonly-
The
BaseAudioContext
which owns thisAudioNode
. numberOfInputs
, of type unsigned long, readonly-
The number of inputs feeding into the
AudioNode
. For source nodes, this will be 0. This attribute is predetermined for manyAudioNode
types, but someAudioNode
s, like theChannelMergerNode
and theAudioWorkletNode
, have variable number of inputs. numberOfOutputs
, of type unsigned long, readonly-
The number of outputs coming out of the
AudioNode
. This attribute is predetermined for someAudioNode
types, but can be variable, like for theChannelSplitterNode
and theAudioWorkletNode
.
1.5.5. Methods
connect(destinationNode, output, input)
-
There can only be one connection between a given output of one specific node and a given input of another specific node. Multiple connections with the same termini are ignored.
For example:nodeA
. connect( nodeB); nodeA. connect( nodeB); will have the same effect as
nodeA
. connect( nodeB); This method returns
destination
AudioNode
object.Arguments for the AudioNode.connect(destinationNode, output, input) method. Parameter Type Nullable Optional Description destinationNode
The destination
parameter is theAudioNode
to connect to. If thedestination
parameter is anAudioNode
that has been created using anotherAudioContext
, anInvalidAccessError
MUST be thrown. That is,AudioNode
s cannot be shared betweenAudioContext
s. MultipleAudioNode
s can be connected to the sameAudioNode
, this is described in Channel Upmixing and down mixing section.output
unsigned long
✘ ✔ The output
parameter is an index describing which output of theAudioNode
from which to connect. If this parameter is out-of-bounds, anIndexSizeError
exception MUST be thrown. It is possible to connect anAudioNode
output to more than one input with multiple calls to connect(). Thus, "fan-out" is supported.input
The input
parameter is an index describing which input of the destinationAudioNode
to connect to. If this parameter is out-of-bounds, anIndexSizeError
exception MUST be thrown. It is possible to connect anAudioNode
to anotherAudioNode
which creates a cycle: anAudioNode
may connect to anotherAudioNode
, which in turn connects back to the input orAudioParam
of the firstAudioNode
.Return type:AudioNode
connect(destinationParam, output)
-
Connects the
AudioNode
to anAudioParam
, controlling the parameter value with an a-rate signal.It is possible to connect an
AudioNode
output to more than oneAudioParam
with multiple calls to connect(). Thus, "fan-out" is supported.It is possible to connect more than one
AudioNode
output to a singleAudioParam
with multiple calls to connect(). Thus, "fan-in" is supported.An
AudioParam
will take the rendered audio data from anyAudioNode
output connected to it and convert it to mono by down-mixing if it is not already mono, then mix it together with other such outputs and finally will mix with the intrinsic parameter value (thevalue
theAudioParam
would normally have without any audio connections), including any timeline changes scheduled for the parameter.The down-mixing to mono is equivalent to the down-mixing for an
AudioNode
withchannelCount
= 1,channelCountMode
= "explicit
", andchannelInterpretation
= "speakers
".There can only be one connection between a given output of one specific node and a specific
AudioParam
. Multiple connections with the same termini are ignored.For example:nodeA
. connect( param); nodeA. connect( param); will have the same effect as
nodeA
. connect( param); Arguments for the AudioNode.connect(destinationParam, output) method. Parameter Type Nullable Optional Description destinationParam
AudioParam
✘ ✘ The destination
parameter is theAudioParam
to connect to. This method does not return thedestination
AudioParam
object. IfdestinationParam
belongs to anAudioNode
that belongs to aBaseAudioContext
that is different from theBaseAudioContext
that has created theAudioNode
on which this method was called, anInvalidAccessError
MUST be thrown.output
unsigned long
✘ ✔ The output
parameter is an index describing which output of theAudioNode
from which to connect. If theparameter
is out-of-bounds, anIndexSizeError
exception MUST be thrown.Return type:undefined
disconnect()
-
Disconnects all outgoing connections from the
AudioNode
.No parameters.Return type:undefined
disconnect(output)
-
Disconnects a single output of the
AudioNode
from any otherAudioNode
orAudioParam
objects to which it is connected.Arguments for the AudioNode.disconnect(output) method. Parameter Type Nullable Optional Description output
unsigned long
✘ ✘ This parameter is an index describing which output of the AudioNode
to disconnect. It disconnects all outgoing connections from the given output. If this parameter is out-of-bounds, anIndexSizeError
exception MUST be thrown.Return type:undefined
disconnect(destinationNode)
-
Disconnects all outputs of the
AudioNode
that go to a specific destinationAudioNode
.Arguments for the AudioNode.disconnect(destinationNode) method. Parameter Type Nullable Optional Description destinationNode
The destinationNode
parameter is theAudioNode
to disconnect. It disconnects all outgoing connections to the givendestinationNode
. If there is no connection to thedestinationNode
, anInvalidAccessError
exception MUST be thrown.Return type:undefined
disconnect(destinationNode, output)
-
Disconnects a specific output of the
AudioNode
from any and all inputs of some destinationAudioNode
.Arguments for the AudioNode.disconnect(destinationNode, output) method. Parameter Type Nullable Optional Description destinationNode
The destinationNode
parameter is theAudioNode
to disconnect. If there is no connection to thedestinationNode
from the given output, anInvalidAccessError
exception MUST be thrown.output
unsigned long
✘ ✘ The output
parameter is an index describing which output of theAudioNode
from which to disconnect. If this parameter is out-of-bounds, anIndexSizeError
exception MUST be thrown.Return type:undefined
disconnect(destinationNode, output, input)
-
Disconnects a specific output of the
AudioNode
from a specific input of some destinationAudioNode
.Arguments for the AudioNode.disconnect(destinationNode, output, input) method. Parameter Type Nullable Optional Description destinationNode
The destinationNode
parameter is theAudioNode
to disconnect. If there is no connection to thedestinationNode
from the given output to the given input, anInvalidAccessError
exception MUST be thrown.output
unsigned long
✘ ✘ The output
parameter is an index describing which output of theAudioNode
from which to disconnect. If this parameter is out-of-bounds, anIndexSizeError
exception MUST be thrown.input
The input
parameter is an index describing which input of the destinationAudioNode
to disconnect. If this parameter is out-of-bounds, anIndexSizeError
exception MUST be thrown.Return type:undefined
disconnect(destinationParam)
-
Disconnects all outputs of the
AudioNode
that go to a specific destinationAudioParam
. The contribution of thisAudioNode
to the computed parameter value goes to 0 when this operation takes effect. The intrinsic parameter value is not affected by this operation.Arguments for the AudioNode.disconnect(destinationParam) method. Parameter Type Nullable Optional Description destinationParam
AudioParam
✘ ✘ The destinationParam
parameter is theAudioParam
to disconnect. If there is no connection to thedestinationParam
, anInvalidAccessError
exception MUST be thrown.Return type:undefined
disconnect(destinationParam, output)
-
Disconnects a specific output of the
AudioNode
from a specific destinationAudioParam
. The contribution of thisAudioNode
to the computed parameter value goes to 0 when this operation takes effect. The intrinsic parameter value is not affected by this operation.Arguments for the AudioNode.disconnect(destinationParam, output) method. Parameter Type Nullable Optional Description destinationParam
AudioParam
✘ ✘ The destinationParam
parameter is theAudioParam
to disconnect. If there is no connection to thedestinationParam
, anInvalidAccessError
exception MUST be thrown.output
unsigned long
✘ ✘ The output
parameter is an index describing which output of theAudioNode
from which to disconnect. If theparameter
is out-of-bounds, anIndexSizeError
exception MUST be thrown.Return type:undefined
1.5.6. AudioNodeOptions
This specifies the options that can be used in constructing all AudioNode
s. All members are optional. However, the specific
values used for each node depends on the actual node.
dictionary AudioNodeOptions {unsigned long channelCount ;ChannelCountMode channelCountMode ;ChannelInterpretation channelInterpretation ; };
1.5.6.1. Dictionary AudioNodeOptions
Members
channelCount
, of type unsigned long-
Desired number of channels for the
channelCount
attribute. channelCountMode
, of type ChannelCountMode-
Desired mode for the
channelCountMode
attribute. channelInterpretation
, of type ChannelInterpretation-
Desired mode for the
channelInterpretation
attribute.
1.6. The AudioParam
Interface
AudioParam
controls an individual aspect of an AudioNode
's functionality, such as volume. The
parameter can be set immediately to a particular value using the value
attribute. Or, value changes can be scheduled to
happen at very precise times (in the coordinate system of AudioContext
's currentTime
attribute), for envelopes, volume
fades, LFOs, filter sweeps, grain windows, etc. In this way,
arbitrary timeline-based automation curves can be set on any AudioParam
. Additionally, audio signals from the
outputs of AudioNode
s can be connected to an AudioParam
, summing with the intrinsic parameter value.
Some synthesis and processing AudioNode
s have AudioParam
s as attributes whose values MUST be taken
into account on a per-audio-sample basis. For other AudioParam
s, sample-accuracy is not important and the
value changes can be sampled more coarsely. Each individual AudioParam
will specify that it is either an a-rate parameter which means that its values MUST be taken
into account on a per-audio-sample basis, or it is a k-rate parameter.
Implementations MUST use block processing, with each AudioNode
processing one render quantum.
For each render quantum,
the value of a k-rate parameter MUST be sampled at the time of the
very first sample-frame, and that value MUST be used for the entire
block. a-rate parameters MUST be sampled for
each sample-frame of the block.
Depending on the AudioParam
, its rate can be controlled by setting
the automationRate
attribute to either
"a-rate
" or "k-rate
". See the
description of the individual AudioParam
s for further details.
Each AudioParam
includes minValue
and maxValue
attributes that together form
the simple nominal range for the parameter. In effect,
value of the parameter is clamped to the range \([\mathrm{minValue},
\mathrm{maxValue}]\). See § 1.6.3 Computation of Value for full details.
For many AudioParam
s the minValue
and maxValue
is intended to be set to the maximum
possible range. In this case, maxValue
should be set to the most-positive-single-float value, which is 3.4028235e38.
(However, in JavaScript which only supports IEEE-754 double precision
float values, this must be written as 3.4028234663852886e38.)
Similarly, minValue
should be set
to the most-negative-single-float value, which is the
negative of the most-positive-single-float: -3.4028235e38.
(Similarly, this must be written in JavaScript as
-3.4028234663852886e38.)
An AudioParam
maintains a list of zero or more automation events. Each automation event
specifies changes to the parameter’s value over a specific time
range, in relation to its automation event time in the
time coordinate system of the AudioContext
's currentTime
attribute. The
list of automation events is maintained in ascending order of
automation event time.
The behavior of a given automation event is a function of the AudioContext
's current time, as well as the automation event
times of this event and of adjacent events in the list. The following automation methods change the
event list by adding a new event to the event list, of a type
specific to the method:
-
setValueAtTime()
-SetValue
-
linearRampToValueAtTime()
-LinearRampToValue
-
exponentialRampToValueAtTime()
-ExponentialRampToValue
-
setTargetAtTime()
-SetTarget
-
setValueCurveAtTime()
-SetValueCurve
The following rules will apply when calling these methods:
-
Automation event times are not quantized with respect to the prevailing sample rate. Formulas for determining curves and ramps are applied to the exact numerical times given when scheduling events.
-
If one of these events is added at a time where there is already one or more events, then it will be placed in the list after them, but before events whose times are after the event.
-
If setValueCurveAtTime() is called for time \(T\) and duration \(D\) and there are any events having a time strictly greater than \(T\), but strictly less than \(T + D\), then a
NotSupportedError
exception MUST be thrown. In other words, it’s not ok to schedule a value curve during a time period containing other events, but it’s ok to schedule a value curve exactly at the time of another event. -
Similarly a
NotSupportedError
exception MUST be thrown if any automation method is called at a time which is contained in \([T, T+D)\), \(T\) being the time of the curve and \(D\) its duration.
Note: AudioParam
attributes are read only, with the exception
of the value
attribute.
The automation rate of an AudioParam
can be selected setting the automationRate
attribute with one of the following
values. However, some AudioParam
s have constraints on whether the
automation rate can be changed.
enum {
AutomationRate "a-rate" ,"k-rate" };
Enum value | Description |
---|---|
"a-rate "
| This AudioParam is set for a-rate processing.
|
"k-rate "
| This AudioParam is set for k-rate processing.
|
Each AudioParam
has an internal slot [[current value]]
,
initially set to the AudioParam
's defaultValue
.
[Exposed =Window ]interface AudioParam {attribute float value ;attribute AutomationRate automationRate ;readonly attribute float defaultValue ;readonly attribute float minValue ;readonly attribute float maxValue ;AudioParam setValueAtTime (float ,
value double );
startTime AudioParam linearRampToValueAtTime (float ,
value double );
endTime AudioParam exponentialRampToValueAtTime (float ,
value double );
endTime AudioParam setTargetAtTime (float ,
target double ,
startTime float );
timeConstant AudioParam setValueCurveAtTime (sequence <float >,
values double ,
startTime double );
duration AudioParam cancelScheduledValues (double );
cancelTime AudioParam cancelAndHoldAtTime (double ); };
cancelTime
1.6.1. Attributes
automationRate
, of type AutomationRate-
The automation rate for the
AudioParam
. The default value depends on the actualAudioParam
; see the description of each individualAudioParam
for the default value.Some nodes have additional automation rate constraints as follows:
AudioBufferSourceNode
-
The
AudioParam
splaybackRate
anddetune
MUST be "k-rate
". AnInvalidStateError
must be thrown if the rate is changed to "a-rate
". DynamicsCompressorNode
-
The
AudioParam
sthreshold
,knee
,ratio
,attack
, andrelease
MUST be "k-rate
". AnInvalidStateError
must be thrown if the rate is changed to "a-rate
". PannerNode
-
If the
panningModel
is "HRTF
", the setting of theautomationRate
for anyAudioParam
of thePannerNode
is ignored. Likewise, the setting of theautomationRate
for anyAudioParam
of theAudioListener
is ignored. In this case, theAudioParam
behaves as if theautomationRate
were set to "k-rate
".
defaultValue
, of type float, readonly-
Initial value for the
value
attribute. maxValue
, of type float, readonly-
The nominal maximum value that the parameter can take. Together with
minValue
, this forms the nominal range for this parameter. minValue
, of type float, readonly-
The nominal minimum value that the parameter can take. Together with
maxValue
, this forms the nominal range for this parameter. value
, of type float-
The parameter’s floating-point value. This attribute is initialized to the
defaultValue
.Getting this attribute returns the contents of the
[[current value]]
slot. See § 1.6.3 Computation of Value for the algorithm for the value that is returned.Setting this attribute has the effect of assigning the requested value to the
[[current value]]
slot, and calling the setValueAtTime() method with the currentAudioContext
'scurrentTime
and[[current value]]
. Any exceptions that would be thrown bysetValueAtTime()
will also be thrown by setting this attribute.
1.6.2. Methods
cancelAndHoldAtTime(cancelTime)
-
This is similar to
cancelScheduledValues()
in that it cancels all scheduled parameter changes with times greater than or equal tocancelTime
. However, in addition, the automation value that would have happened atcancelTime
is then proprogated for all future time until other automation events are introduced.The behavior of the timeline in the face of
cancelAndHoldAtTime()
when automations are running and can be introduced at any time after callingcancelAndHoldAtTime()
and beforecancelTime
is reached is quite complicated. The behavior ofcancelAndHoldAtTime()
is therefore specified in the following algorithm.Let \(t_c\) be the value ofcancelTime
. Then-
Let \(E_1\) be the event (if any) at time \(t_1\) where \(t_1\) is the largest number satisfying \(t_1 \le t_c\).
-
Let \(E_2\) be the event (if any) at time \(t_2\) where \(t_2\) is the smallest number satisfying \(t_c \lt t_2\).
-
If \(E_2\) exists:
-
If \(E_2\) is a linear or exponential ramp,
-
Effectively rewrite \(E_2\) to be the same kind of ramp ending at time \(t_c\) with an end value that would be the value of the original ramp at time \(t_c\).
-
Go to step 5.
-
-
Otherwise, go to step 4.
-
-
If \(E_1\) exists:
-
If \(E_1\) is a
setTarget
event,-
Implicitly insert a
setValueAtTime
event at time \(t_c\) with the value that thesetTarget
would have at time \(t_c\). -
Go to step 5.
-
-
If \(E_1\) is a
setValueCurve
with a start time of \(t_3\) and a duration of \(d\)-
If \(t_c \gt t_3 + d\), go to step 5.
-
Otherwise,
-
Effectively replace this event with a
setValueCurve
event with a start time of \(t_3\) and a new duration of \(t_c-t_3\). However, this is not a true replacement; this automation MUST take care to produce the same output as the original, and not one computed using a different duration. (That would cause sampling of the value curve in a slightly different way, producing different results.) -
Go to step 5.
-
-
-
-
Remove all events with time greater than \(t_c\).
If no events are added, then the automation value after
cancelAndHoldAtTime()
is the constant value that the original timeline would have had at time \(t_c\).Candidate Correction Issue 127: ARangeError
is only thrown for negative cancelTime values for cancelAndHoldAtTime and cancelScheduledValues. See Issue 127Arguments for the AudioParam.cancelAndHoldAtTime() method. Parameter Type Nullable Optional Description cancelTime
double
✘ ✘ The time after which any previously scheduled parameter changes will be cancelled. It is a time in the same time coordinate system as the AudioContext
'scurrentTime
attribute. ARangeError
exception MUST be thrown ifcancelTime
is negativeor is not a finite number. IfcancelTime
is less thancurrentTime
, it is clamped tocurrentTime
.Return type:AudioParam
-
cancelScheduledValues(cancelTime)
-
Cancels all scheduled parameter changes with times greater than or equal to
cancelTime
. Cancelling a scheduled parameter change means removing the scheduled event from the event list. Any active automations whose automation event time is less thancancelTime
are also cancelled, and such cancellations may cause discontinuities because the original value (from before such automation) is restored immediately. Any hold values scheduled bycancelAndHoldAtTime()
are also removed if the hold time occurs aftercancelTime
.For a
setValueCurveAtTime()
, let \(T_0\) and \(T_D\) be the correspondingstartTime
andduration
, respectively of this event. Then ifcancelTime
is in the range \([T_0, T_0 + T_D]\), the event is removed from the timeline.Arguments for the AudioParam.cancelScheduledValues() method. Parameter Type Nullable Optional Description cancelTime
double
✘ ✘ The time after which any previously scheduled parameter changes will be cancelled. It is a time in the same time coordinate system as the AudioContext
'scurrentTime
attribute. ARangeError
exception MUST be thrown ifcancelTime
is negativeor is not a finite number. IfcancelTime
is less thancurrentTime
, it is clamped tocurrentTime
.Return type:AudioParam
exponentialRampToValueAtTime(value, endTime)
-
Schedules an exponential continuous change in parameter value from the previous scheduled parameter value to the given value. Parameters representing filter frequencies and playback rate are best changed exponentially because of the way humans perceive sound.
The value during the time interval \(T_0 \leq t < T_1\) (where \(T_0\) is the time of the previous event and \(T_1\) is the
endTime
parameter passed into this method) will be calculated as:$$ v(t) = V_0 \left(\frac{V_1}{V_0}\right)^\frac{t - T_0}{T_1 - T_0} $$
where \(V_0\) is the value at the time \(T_0\) and \(V_1\) is the
value
parameter passed into this method. If \(V_0\) and \(V_1\) have opposite signs or if \(V_0\) is zero, then \(v(t) = V_0\) for \(T_0 \le t \lt T_1\).This also implies an exponential ramp to 0 is not possible. A good approximation can be achieved using
setTargetAtTime()
with an appropriately chosen time constant.If there are no more events after this ExponentialRampToValue event then for \(t \geq T_1\), \(v(t) = V_1\).
If there is no event preceding this event, the exponential ramp behaves as if
setValueAtTime(value, currentTime)
were called wherevalue
is the current value of the attribute andcurrentTime
is the contextcurrentTime
at the timeexponentialRampToValueAtTime()
is called.If the preceding event is a
SetTarget
event, \(T_0\) and \(V_0\) are chosen from the current time and value ofSetTarget
automation. That is, if theSetTarget
event has not started, \(T_0\) is the start time of the event, and \(V_0\) is the value just before theSetTarget
event starts. In this case, theExponentialRampToValue
event effectively replaces theSetTarget
event. If theSetTarget
event has already started, \(T_0\) is the current context time, and \(V_0\) is the currentSetTarget
automation value at time \(T_0\). In both cases, the automation curve is continuous.Arguments for the AudioParam.exponentialRampToValueAtTime() method. Parameter Type Nullable Optional Description value
float
✘ ✘ The value the parameter will exponentially ramp to at the given time. A RangeError
exception MUST be thrown if this value is equal to 0.endTime
double
✘ ✘ The time in the same time coordinate system as the AudioContext
'scurrentTime
attribute where the exponential ramp ends. ARangeError
exception MUST be thrown ifendTime
is negative or is not a finite number. If endTime is less thancurrentTime
, it is clamped tocurrentTime
.Return type:AudioParam
linearRampToValueAtTime(value, endTime)
-
Schedules a linear continuous change in parameter value from the previous scheduled parameter value to the given value.
The value during the time interval \(T_0 \leq t < T_1\) (where \(T_0\) is the time of the previous event and \(T_1\) is the
endTime
parameter passed into this method) will be calculated as:$$ v(t) = V_0 + (V_1 - V_0) \frac{t - T_0}{T_1 - T_0} $$
where \(V_0\) is the value at the time \(T_0\) and \(V_1\) is the
value
parameter passed into this method.If there are no more events after this LinearRampToValue event then for \(t \geq T_1\), \(v(t) = V_1\).
If there is no event preceding this event, the linear ramp behaves as if
setValueAtTime(value, currentTime)
were called wherevalue
is the current value of the attribute andcurrentTime
is the contextcurrentTime
at the timelinearRampToValueAtTime()
is called.If the preceding event is a
SetTarget
event, \(T_0\) and \(V_0\) are chosen from the current time and value ofSetTarget
automation. That is, if theSetTarget
event has not started, \(T_0\) is the start time of the event, and \(V_0\) is the value just before theSetTarget
event starts. In this case, theLinearRampToValue
event effectively replaces theSetTarget
event. If theSetTarget
event has already started, \(T_0\) is the current context time, and \(V_0\) is the currentSetTarget
automation value at time \(T_0\). In both cases, the automation curve is continuous.Arguments for the AudioParam.linearRampToValueAtTime() method. Parameter Type Nullable Optional Description value
float
✘ ✘ The value the parameter will linearly ramp to at the given time. endTime
double
✘ ✘ The time in the same time coordinate system as the AudioContext
'scurrentTime
attribute at which the automation ends. ARangeError
exception MUST be thrown ifendTime
is negative or is not a finite number. If endTime is less thancurrentTime
, it is clamped tocurrentTime
.Return type:AudioParam
setTargetAtTime(target, startTime, timeConstant)
-
Start exponentially approaching the target value at the given time with a rate having the given time constant. Among other uses, this is useful for implementing the "decay" and "release" portions of an ADSR envelope. Please note that the parameter value does not immediately change to the target value at the given time, but instead gradually changes to the target value.
During the time interval: \(T_0 \leq t\), where \(T_0\) is the
startTime
parameter:$$ v(t) = V_1 + (V_0 - V_1)\, e^{-\left(\frac{t - T_0}{\tau}\right)} $$
where \(V_0\) is the initial value (the
[[current value]]
attribute) at \(T_0\) (thestartTime
parameter), \(V_1\) is equal to thetarget
parameter, and \(\tau\) is thetimeConstant
parameter.If a
LinearRampToValue
orExponentialRampToValue
event follows this event, the behavior is described inlinearRampToValueAtTime()
orexponentialRampToValueAtTime()
, respectively. For all other events, theSetTarget
event ends at the time of the next event.Arguments for the AudioParam.setTargetAtTime() method. Parameter Type Nullable Optional Description target
float
✘ ✘ The value the parameter will start changing to at the given time. startTime
double
✘ ✘ The time at which the exponential approach will begin, in the same time coordinate system as the AudioContext
'scurrentTime
attribute. ARangeError
exception MUST be thrown ifstart
is negative or is not a finite number. If startTime is less thancurrentTime
, it is clamped tocurrentTime
.timeConstant
float
✘ ✘ The time-constant value of first-order filter (exponential) approach to the target value. The larger this value is, the slower the transition will be. The value MUST be non-negative or a RangeError
exception MUST be thrown. IftimeConstant
is zero, the output value jumps immediately to the final value. More precisely, timeConstant is the time it takes a first-order linear continuous time-invariant system to reach the value \(1 - 1/e\) (around 63.2%) given a step input response (transition from 0 to 1 value).Return type:AudioParam
setValueAtTime(value, startTime)
-
Schedules a parameter value change at the given time.
If there are no more events after this
SetValue
event, then for \(t \geq T_0\), \(v(t) = V\), where \(T_0\) is thestartTime
parameter and \(V\) is thevalue
parameter. In other words, the value will remain constant.If the next event (having time \(T_1\)) after this
SetValue
event is not of typeLinearRampToValue
orExponentialRampToValue
, then, for \(T_0 \leq t < T_1\):$$ v(t) = V $$
In other words, the value will remain constant during this time interval, allowing the creation of "step" functions.
If the next event after this
SetValue
event is of typeLinearRampToValue
orExponentialRampToValue
then please seelinearRampToValueAtTime()
orexponentialRampToValueAtTime()
, respectively.Arguments for the AudioParam.setValueAtTime() method. Parameter Type Nullable Optional Description value
float
✘ ✘ The value the parameter will change to at the given time. startTime
double
✘ ✘ The time in the same time coordinate system as the BaseAudioContext
'scurrentTime
attribute at which the parameter changes to the given value. ARangeError
exception MUST be thrown ifstartTime
is negative or is not a finite number. If startTime is less thancurrentTime
, it is clamped tocurrentTime
.Return type:AudioParam
setValueCurveAtTime(values, startTime, duration)
-
Sets an array of arbitrary parameter values starting at the given time for the given duration. The number of values will be scaled to fit into the desired duration.
Let \(T_0\) be
startTime
, \(T_D\) beduration
, \(V\) be thevalues
array, and \(N\) be the length of thevalues
array. Then, during the time interval: \(T_0 \le t < T_0 + T_D\), let$$ \begin{align*} k &= \left\lfloor \frac{N - 1}{T_D}(t-T_0) \right\rfloor \\ \end{align*} $$
Then \(v(t)\) is computed by linearly interpolating between \(V[k]\) and \(V[k+1]\),
After the end of the curve time interval (\(t \ge T_0 + T_D\)), the value will remain constant at the final curve value, until there is another automation event (if any).
An implicit call to
setValueAtTime()
is made at time \(T_0 + T_D\) with value \(V[N-1]\) so that following automations will start from the end of thesetValueCurveAtTime()
event.Arguments for the AudioParam.setValueCurveAtTime() method. Parameter Type Nullable Optional Description values
sequence<float>
✘ ✘ A sequence of float values representing a parameter value curve. These values will apply starting at the given time and lasting for the given duration. When this method is called, an internal copy of the curve is created for automation purposes. Subsequent modifications of the contents of the passed-in array therefore have no effect on the AudioParam
. AnInvalidStateError
MUST be thrown if this attribute is asequence<float>
object that has a length less than 2.startTime
double
✘ ✘ The start time in the same time coordinate system as the AudioContext
'scurrentTime
attribute at which the value curve will be applied. ARangeError
exception MUST be thrown ifstartTime
is negative or is not a finite number. If startTime is less thancurrentTime
, it is clamped tocurrentTime
.duration
double
✘ ✘ The amount of time in seconds (after the startTime
parameter) where values will be calculated according to thevalues
parameter. ARangeError
exception MUST be thrown ifduration
is not strictly positive or is not a finite number.Return type:AudioParam
1.6.3. Computation of Value
There are two different kind of AudioParam
s, simple
parameters and compound parameters. Simple parameters (the default) are used
on their own to compute the final audio output of an AudioNode
. Compound
parameters are AudioParam
s that are used with other AudioParam
s to compute a value that is then used as an input
to compute the output of an AudioNode
.
The computedValue is the final value controlling the audio DSP and is computed by the audio rendering thread during each rendering time quantum.
AudioParam
consists of two parts:
-
the paramIntrinsicValue value that is computed from the
value
attribute and any automation events. -
the paramComputedValue that is the final value controlling the audio DSP and is computed by the audio rendering thread during each render quantum.
These values MUST be computed as follows:
-
paramIntrinsicValue will be calculated at each time, which is either the value set directly to the
value
attribute, or, if there are any automation events with times before or at this time, the value as calculated from these events. If automation events are removed from a given time range, then the paramIntrinsicValue value will remain unchanged and stay at its previous value until either thevalue
attribute is directly set, or automation events are added for the time range. -
Set
[[current value]]
to the value of paramIntrinsicValue at the beginning of this render quantum. -
paramComputedValue is the sum of the paramIntrinsicValue value and the value of the input AudioParam buffer. If the sum is
NaN
, replace the sum with thedefaultValue
. -
If this
AudioParam
is a compound parameter, compute its final value with otherAudioParam
s. -
Set computedValue to paramComputedValue.
The nominal range for a computedValue are the
lower and higher values this parameter can effectively have. For simple parameters, the computedValue is clamped to
the simple nominal range for this parameter. Compound
parameters have their final value clamped to their nominal
range after having been computed from the different AudioParam
values they are composed of.
When automation methods are used, clamping is still applied. However, the automation is run as if there were no clamping at all. Only when the automation values are to be applied to the output is the clamping done as specified above.
N. p. setValueAtTime( 0 , 0 ); N. p. linearRampToValueAtTime( 4 , 1 ); N. p. linearRampToValueAtTime( 0 , 2 );
The initial slope of the curve is 4, until it reaches the maximum value of 1, at which time, the output is held constant. Finally, near time 2, the slope of the curve is -4. This is illustrated in the graph below where the dashed line indicates what would have happened without clipping, and the solid line indicates the actual expected behavior of the audioparam due to clipping to the nominal range.
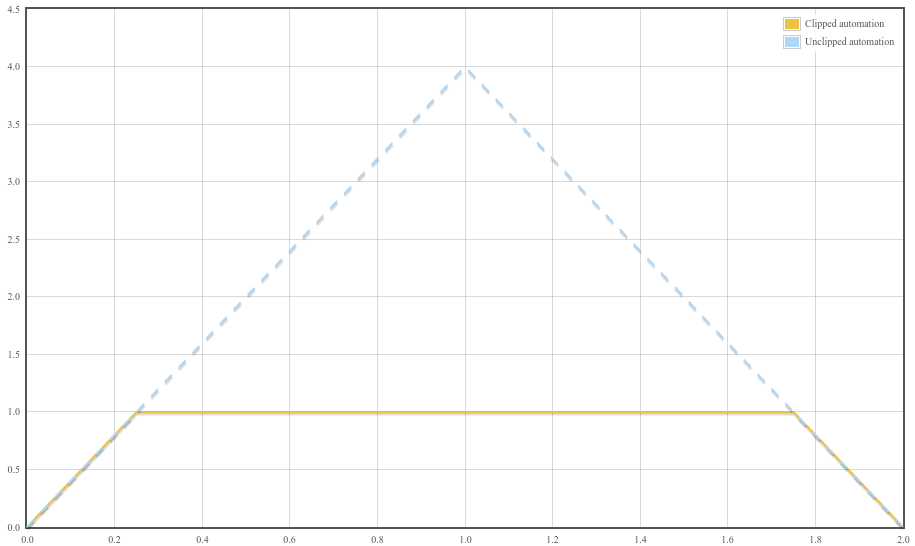
1.6.4. AudioParam
Automation Example
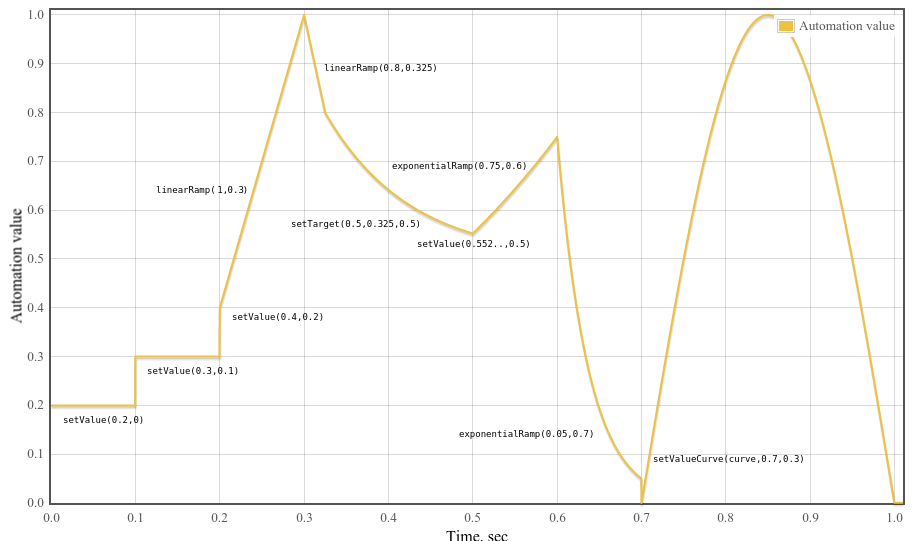
const curveLength= 44100 ; const curve= new Float32Array( curveLength); for ( const i= 0 ; i< curveLength; ++ i) curve[ i] = Math. sin( Math. PI* i/ curveLength); const t0= 0 ; const t1= 0.1 ; const t2= 0.2 ; const t3= 0.3 ; const t4= 0.325 ; const t5= 0.5 ; const t6= 0.6 ; const t7= 0.7 ; const t8= 1.0 ; const timeConstant= 0.1 ; param. setValueAtTime( 0.2 , t0); param. setValueAtTime( 0.3 , t1); param. setValueAtTime( 0.4 , t2); param. linearRampToValueAtTime( 1 , t3); param. linearRampToValueAtTime( 0.8 , t4); param. setTargetAtTime( .5 , t4, timeConstant); // Compute where the setTargetAtTime will be at time t5 so we can make // the following exponential start at the right point so there’s no // jump discontinuity. From the spec, we have // v(t) = 0.5 + (0.8 - 0.5)*exp(-(t-t4)/timeConstant) // Thus v(t5) = 0.5 + (0.8 - 0.5)*exp(-(t5-t4)/timeConstant) param. setValueAtTime( 0.5 + ( 0.8 - 0.5 ) * Math. exp( - ( t5- t4) / timeConstant), t5); param. exponentialRampToValueAtTime( 0.75 , t6); param. exponentialRampToValueAtTime( 0.05 , t7); param. setValueCurveAtTime( curve, t7, t8- t7);
1.7. The AudioScheduledSourceNode
Interface
The interface represents the common features of source nodes such
as AudioBufferSourceNode
, ConstantSourceNode
, and OscillatorNode
.
Before a source is started (by calling start()
, the source node
MUST output silence (0). After a source has been stopped (by calling stop()
),
the source MUST then output silence (0).
AudioScheduledSourceNode
cannot be instantiated directly, but
is instead extended by the concrete interfaces for the source nodes.
An AudioScheduledSourceNode
is said to be playing when
its associated BaseAudioContext
's currentTime
is
greater or equal to the time the AudioScheduledSourceNode
is set to start,
and less than the time it’s set to stop.
AudioScheduledSourceNode
s are created with an internal boolean
slot [[source started]]
, initially
set to false.
[Exposed =Window ]interface AudioScheduledSourceNode :AudioNode {attribute EventHandler onended ;undefined start (optional double when = 0);undefined stop (optional double when = 0); };
1.7.1. Attributes
onended
, of type EventHandler-
A property used to set an event handler for the
ended
event type that is dispatched toAudioScheduledSourceNode
node types. When the source node has stopped playing (as determined by the concrete node), an event that uses theEvent
interface will be dispatched to the event handler.For all
AudioScheduledSourceNode
s, theended
event is dispatched when the stop time determined bystop()
is reached. For anAudioBufferSourceNode
, the event is also dispatched because theduration
has been reached or if the entirebuffer
has been played.
1.7.2. Methods
start(when)
-
Schedules a sound to playback at an exact time.
When this method is called, execute these steps:-
If this
AudioScheduledSourceNode
internal slot[[source started]]
is true, anInvalidStateError
exception MUST be thrown. -
Check for any errors that must be thrown due to parameter constraints described below. If any exception is thrown during this step, abort those steps.
-
Set the internal slot
[[source started]]
on thisAudioScheduledSourceNode
totrue
. -
Queue a control message to start the
AudioScheduledSourceNode
, including the parameter values in the message. -
Send a control message to the associated
AudioContext
to start running its rendering thread only when all the following conditions are met:-
The context’s
[[control thread state]]
is "suspended
". -
The context is allowed to start.
-
[[suspended by user]]
flag isfalse
.
NOTE: This allows
start()
to start anAudioContext
that would otherwise not be allowed to start. -
Arguments for the AudioScheduledSourceNode.start(when) method. Parameter Type Nullable Optional Description when
double
✘ ✔ The when
parameter describes at what time (in seconds) the sound should start playing. It is in the same time coordinate system as theAudioContext
'scurrentTime
attribute. When the signal emitted by theAudioScheduledSourceNode
depends on the sound’s start time, the exact value ofwhen
is always used without rounding to the nearest sample frame. If 0 is passed in for this value or if the value is less thancurrentTime
, then the sound will start playing immediately. ARangeError
exception MUST be thrown ifwhen
is negative.Return type:undefined
-
stop(when)
-
Schedules a sound to stop playback at an exact time. If
stop
is called again after already having been called, the last invocation will be the only one applied; stop times set by previous calls will not be applied, unless the buffer has already stopped prior to any subsequent calls. If the buffer has already stopped, further calls tostop
will have no effect. If a stop time is reached prior to the scheduled start time, the sound will not play.When this method is called, execute these steps:-
If this
AudioScheduledSourceNode
internal slot[[source started]]
is nottrue
, anInvalidStateError
exception MUST be thrown. -
Check for any errors that must be thrown due to parameter constraints described below.
-
Queue a control message to stop the
AudioScheduledSourceNode
, including the parameter values in the message.
If the node is anAudioBufferSourceNode
, running a control message to stop theAudioBufferSourceNode
means invoking thehandleStop()
function in the playback algorithm.Arguments for the AudioScheduledSourceNode.stop(when) method. Parameter Type Nullable Optional Description when
double
✘ ✔ The when
parameter describes at what time (in seconds) the source should stop playing. It is in the same time coordinate system as theAudioContext
'scurrentTime
attribute. If 0 is passed in for this value or if the value is less thancurrentTime
, then the sound will stop playing immediately. ARangeError
exception MUST be thrown ifwhen
is negative.Return type:undefined
-
1.8. The AnalyserNode
Interface
This interface represents a node which is able to provide real-time frequency and time-domain analysis information. The audio stream will be passed un-processed from input to output.
Property | Value | Notes |
---|---|---|
numberOfInputs
| 1 | |
numberOfOutputs
| 1 | This output may be left unconnected. |
channelCount
| 2 | |
channelCountMode
| "max "
| |
channelInterpretation
| "speakers "
| |
tail-time | No |
[Exposed =Window ]interface AnalyserNode :AudioNode {constructor (BaseAudioContext ,
context optional AnalyserOptions = {});
options undefined getFloatFrequencyData (Float32Array );
array undefined getByteFrequencyData (Uint8Array );
array undefined getFloatTimeDomainData (Float32Array );
array undefined getByteTimeDomainData (Uint8Array );
array attribute unsigned long fftSize ;readonly attribute unsigned long frequencyBinCount ;attribute double minDecibels ;attribute double maxDecibels ;attribute double smoothingTimeConstant ; };
1.8.1. Constructors
AnalyserNode(context, options)
-
When the constructor is called with a
BaseAudioContext
c and an option object option, the user agent MUST initialize the AudioNode this, with context and options as arguments.Arguments for the AnalyserNode.constructor() method. Parameter Type Nullable Optional Description context
BaseAudioContext
✘ ✘ The BaseAudioContext
this newAnalyserNode
will be associated with.options
AnalyserOptions
✘ ✔ Optional initial parameter value for this AnalyserNode
.
1.8.2. Attributes
fftSize
, of type unsigned long-
The size of the FFT used for frequency-domain analysis (in sample-frames). This MUST be a power of two in the range 32 to 32768, otherwise an
IndexSizeError
exception MUST be thrown. The default value is 2048. Note that large FFT sizes can be costly to compute.If the
fftSize
is changed to a different value, then all state associated with smoothing of the frequency data (forgetByteFrequencyData()
andgetFloatFrequencyData()
) is reset. That is the previous block, \(\hat{X}_{-1}[k]\), used for smoothing over time is set to 0 for all \(k\).Note that increasing
fftSize
does mean that the current time-domain data must be expanded to include past frames that it previously did not. This means that theAnalyserNode
effectively MUST keep around the last 32768 sample-frames and the current time-domain data is the most recentfftSize
sample-frames out of that. frequencyBinCount
, of type unsigned long, readonly-
Half the FFT size.
maxDecibels
, of type double-
maxDecibels
is the maximum power value in the scaling range for the FFT analysis data for conversion to unsigned byte values. The default value is -30. If the value of this attribute is set to a value less than or equal tominDecibels
, anIndexSizeError
exception MUST be thrown. minDecibels
, of type double-
minDecibels
is the minimum power value in the scaling range for the FFT analysis data for conversion to unsigned byte values. The default value is -100. If the value of this attribute is set to a value more than or equal tomaxDecibels
, anIndexSizeError
exception MUST be thrown. smoothingTimeConstant
, of type double-
A value from 0 -> 1 where 0 represents no time averaging with the last analysis frame. The default value is 0.8. If the value of this attribute is set to a value less than 0 or more than 1, an
IndexSizeError
exception MUST be thrown.
1.8.3. Methods
getByteFrequencyData(array)
-
Proposed Correction Issue 2361-6. Use new Web IDL buffer primitives
Get a reference to the bytes held by theWrite the current frequency data into array. If array’s byte length is less thanUint8Array
passed as an argument. Copies the current frequency data to those bytes. If the array has fewer elements than thefrequencyBinCount
, the excess elements will be dropped. If the array has more elements than thefrequencyBinCount
frequencyBinCount
, the excess elements will be dropped. If array’s byte length is greater than thefrequencyBinCount
, the excess elements will be ignored. The most recentfftSize
frames are used in computing the frequency data.If another call to
getByteFrequencyData()
orgetFloatFrequencyData()
occurs within the same render quantum as a previous call, the current frequency data is not updated with the same data. Instead, the previously computed data is returned.The values stored in the unsigned byte array are computed in the following way. Let \(Y[k]\) be the current frequency data as described in FFT windowing and smoothing. Then the byte value, \(b[k]\), is
$$ b[k] = \left\lfloor \frac{255}{\mbox{dB}_{max} - \mbox{dB}_{min}} \left(Y[k] - \mbox{dB}_{min}\right) \right\rfloor $$
where \(\mbox{dB}_{min}\) is
minDecibels
and \(\mbox{dB}_{max}\) is
. If \(b[k]\) lies outside the range of 0 to 255, \(b[k]\) is clipped to lie in that range.maxDecibels
Arguments for the AnalyserNode.getByteFrequencyData() method. Parameter Type Nullable Optional Description array
Uint8Array
✘ ✘ This parameter is where the frequency-domain analysis data will be copied. Return type:undefined
getByteTimeDomainData(array)
-
Proposed Correction Issue 2361-7. Use new Web IDL buffer primitives
Get a reference to the bytes held by theWrite the current time-domain data (waveform data) into array. If array’s byte length is less thanUint8Array
passed as an argument. Copies the current time-domain data (waveform data) into those bytes. If the array has fewer elements than the value offftSize
, the excess elements will be dropped. If the array has more elements thanfftSize
,fftSize
, the excess elements will be dropped. If array’s byte length is greater than thefftSize
, the excess elements will be ignored. The most recentfftSize
frames are used in computing the byte data.The values stored in the unsigned byte array are computed in the following way. Let \(x[k]\) be the time-domain data. Then the byte value, \(b[k]\), is
$$ b[k] = \left\lfloor 128(1 + x[k]) \right\rfloor. $$
If \(b[k]\) lies outside the range 0 to 255, \(b[k]\) is clipped to lie in that range.
Arguments for the AnalyserNode.getByteTimeDomainData() method. Parameter Type Nullable Optional Description array
Uint8Array
✘ ✘ This parameter is where the time-domain sample data will be copied. Return type:undefined
getFloatFrequencyData(array)
-
Proposed Correction Issue 2361-8. Use new Web IDL buffer primitives
Get a reference to the bytes held by theWrite the current frequency data into array. If array has fewer elements than theFloat32Array
passed as an argument. Copies the current frequency data into those bytes. If the array has fewer elements than thefrequencyBinCount
,frequencyBinCount
, the excess elements will be dropped. If array has more elements than thefrequencyBinCount
, the excess elements will be ignored. The most recentfftSize
frames are used in computing the frequency data.If another call to
getFloatFrequencyData()
orgetByteFrequencyData()
occurs within the same render quantum as a previous call, the current frequency data is not updated with the same data. Instead, the previously computed data is returned.The frequency data are in dB units.
Arguments for the AnalyserNode.getFloatFrequencyData() method. Parameter Type Nullable Optional Description array
Float32Array
✘ ✘ This parameter is where the frequency-domain analysis data will be copied. Return type:undefined
getFloatTimeDomainData(array)
-
Proposed Correction Issue 2361-9. Use new Web IDL buffer primitives
Get a reference to the bytes held by theWrite the current time-domain data (waveform data) into array. If array has fewer elements than the value ofFloat32Array
passed as an argument. Copies the current time-domain data (waveform data) into those bytes. If the array has fewer elements than the value offftSize
, the excess elements will be dropped. If the array has more elements thanfftSize
,fftSize
, the excess elements will be dropped. If array has more elements than the value offftSize
, the excess elements will be ignored. The most recentfftSize
frames are written (after downmixing).Arguments for the AnalyserNode.getFloatTimeDomainData() method. Parameter Type Nullable Optional Description array
Float32Array
✘ ✘ This parameter is where the time-domain sample data will be copied. Return type:undefined
1.8.4. AnalyserOptions
This specifies the options to be used when constructing an AnalyserNode
. All members are optional; if not
specified, the normal default values are used to construct the
node.
dictionary AnalyserOptions :AudioNodeOptions {unsigned long fftSize = 2048;double maxDecibels = -30;double minDecibels = -100;double smoothingTimeConstant = 0.8; };
1.8.4.1. Dictionary AnalyserOptions
Members
fftSize
, of type unsigned long, defaulting to2048
-
The desired initial size of the FFT for frequency-domain analysis.
maxDecibels
, of type double, defaulting to-30
-
The desired initial maximum power in dB for FFT analysis.
minDecibels
, of type double, defaulting to-100
-
The desired initial minimum power in dB for FFT analysis.
smoothingTimeConstant
, of type double, defaulting to0.8
-
The desired initial smoothing constant for the FFT analysis.
1.8.5. Time-Domain Down-Mixing
When the current time-domain data are computed, the
input signal must be down-mixed to mono as if channelCount
is 1, channelCountMode
is
"max
" and channelInterpretation
is "speakers
". This is independent of the
settings for the AnalyserNode
itself. The most recent fftSize
frames are used for the
down-mixing operation.
1.8.6. FFT Windowing and Smoothing over Time
When the current frequency data are computed, the following operations are to be performed:
-
Compute the current time-domain data.
-
Apply a Blackman window to the time domain input data.
-
Apply a Fourier transform to the windowed time domain input data to get real and imaginary frequency data.
-
Smooth over time the frequency domain data.
In the following, let \(N\) be the value of the fftSize
attribute of this AnalyserNode
.
$$ \begin{align*} \alpha &= \mbox{0.16} \\ a_0 &= \frac{1-\alpha}{2} \\ a_1 &= \frac{1}{2} \\ a_2 &= \frac{\alpha}{2} \\ w[n] &= a_0 - a_1 \cos\frac{2\pi n}{N} + a_2 \cos\frac{4\pi n}{N}, \mbox{ for } n = 0, \ldots, N - 1 \end{align*} $$
The windowed signal \(\hat{x}[n]\) is
$$ \hat{x}[n] = x[n] w[n], \mbox{ for } n = 0, \ldots, N - 1 $$
$$ X[k] = \frac{1}{N} \sum_{n = 0}^{N - 1} \hat{x}[n]\, W^{-kn}_{N} $$
for \(k = 0, \dots, N/2-1\) where \(W_N = e^{2\pi i/N}\).
-
Let \(\hat{X}_{-1}[k]\) be the result of this operation on the previous block. The previous block is defined as being the buffer computed by the previous smoothing over time operation, or an array of \(N\) zeros if this is the first time we are smoothing over time.
-
Let \(\tau\) be the value of the
smoothingTimeConstant
attribute for thisAnalyserNode
. -
Let \(X[k]\) be the result of applying a Fourier transform of the current block.
Then the smoothed value, \(\hat{X}[k]\), is computed by
$$ \hat{X}[k] = \tau\, \hat{X}_{-1}[k] + (1 - \tau)\, \left|X[k]\right| $$
-
If \(\hat{X}[k]\) is
NaN
, positive infinity or negative infinity, set \(\hat{X}[k]\) = 0.
for \(k = 0, \ldots, N - 1\).
$$ Y[k] = 20\log_{10}\hat{X}[k] $$
for \(k = 0, \ldots, N-1\).
This array, \(Y[k]\), is copied to the output array for getFloatFrequencyData()
. For getByteFrequencyData()
, the \(Y[k]\) is clipped to lie
between minDecibels
and
and then scaled to fit in an
unsigned byte such that maxDecibels
minDecibels
is
represented by the value 0 and
is
represented by the value 255.maxDecibels
1.9. The AudioBufferSourceNode
Interface
This interface represents an audio source from an in-memory audio
asset in an AudioBuffer
. It is useful for playing audio
assets which require a high degree of scheduling flexibility and
accuracy. If sample-accurate playback of network- or disk-backed
assets is required, an implementer should use AudioWorkletNode
to implement playback.
The start()
method is used to
schedule when sound playback will happen. The start()
method may not be
issued multiple times. The playback will stop automatically when the
buffer’s audio data has been completely played (if the loop
attribute is false
), or when the stop()
method has been
called and the specified time has been reached. Please see more
details in the start()
and stop()
descriptions.
Property | Value | Notes |
---|---|---|
numberOfInputs
| 0 | |
numberOfOutputs
| 1 | |
channelCount
| 2 | |
channelCountMode
| "max "
| |
channelInterpretation
| "speakers "
| |
tail-time | No |
The number of channels of the output equals the number of channels of the
AudioBuffer assigned to the buffer
attribute,
or is one channel of silence if buffer
is null
.
In addition, if the buffer has more than one channel,
then the AudioBufferSourceNode
output must change to a single channel
of silence at the beginning of a render quantum after the time at which any one
of the following conditions holds:
-
the end of the
buffer
has been reached; -
the
duration
has been reached; -
the
stop
time has been reached.
A playhead position for an AudioBufferSourceNode
is
defined as any quantity representing a time offset in seconds,
relative to the time coordinate of the first sample frame in the
buffer. Such values are to be considered independently from the
node’s playbackRate
and detune
parameters.
In general, playhead positions may be subsample-accurate and need not
refer to exact sample frame positions. They may assume valid values
between 0 and the duration of the buffer.
The playbackRate
and detune
attributes form a compound parameter. They are used together to determine a computedPlaybackRate value:
computedPlaybackRate(t) = playbackRate(t) * pow(2, detune(t) / 1200)
The nominal range for this compound parameter is \((-\infty, \infty)\).
AudioBufferSourceNode
s are created with an internal boolean
slot [[buffer set]]
, initially set to false.
[Exposed =Window ]interface AudioBufferSourceNode :AudioScheduledSourceNode {constructor (BaseAudioContext ,
context optional AudioBufferSourceOptions = {});
options attribute AudioBuffer ?buffer ;readonly attribute AudioParam playbackRate ;readonly attribute AudioParam detune ;attribute boolean loop ;attribute double loopStart ;attribute double loopEnd ;undefined start (optional double when = 0,optional double offset ,optional double duration ); };
1.9.1. Constructors
AudioBufferSourceNode(context, options)
-
When the constructor is called with a
BaseAudioContext
c and an option object option, the user agent MUST initialize the AudioNode this, with context and options as arguments.Arguments for the AudioBufferSourceNode.constructor() method. Parameter Type Nullable Optional Description context
BaseAudioContext
✘ ✘ The BaseAudioContext
this newAudioBufferSourceNode
will be associated with.options
AudioBufferSourceOptions
✘ ✔ Optional initial parameter value for this AudioBufferSourceNode
.
1.9.2. Attributes
buffer
, of type AudioBuffer, nullable-
Represents the audio asset to be played.
To set thebuffer
attribute, execute these steps:-
Let new buffer be the
AudioBuffer
ornull
value to be assigned tobuffer
. -
If new buffer is not
null
and[[buffer set]]
is true, throw anInvalidStateError
and abort these steps. -
If new buffer is not
null
, set[[buffer set]]
to true. -
Assign new buffer to the
buffer
attribute. -
If
start()
has previously been called on this node, perform the operation acquire the content onbuffer
.
-
detune
, of type AudioParam, readonly-
An additional parameter, in cents, to modulate the speed at which is rendered the audio stream. This parameter is a compound parameter with
playbackRate
to form a computedPlaybackRate.Parameter Value Notes defaultValue
0 minValue
most-negative-single-float Approximately -3.4028235e38 maxValue
most-positive-single-float Approximately 3.4028235e38 automationRate
" k-rate
"Has automation rate constraints loop
, of type boolean-
Indicates if the region of audio data designated by
loopStart
andloopEnd
should be played continuously in a loop. The default value isfalse
. loopEnd
, of type double-
An optional playhead position where looping should end if the
loop
attribute is true. Its value is exclusive of the content of the loop. Its defaultvalue
is 0, and it may usefully be set to any value between 0 and the duration of the buffer. IfloopEnd
is less than or equal to 0, or ifloopEnd
is greater than the duration of the buffer, looping will end at the end of the buffer. loopStart
, of type double-
An optional playhead position where looping should begin if the
loop
attribute is true. Its defaultvalue
is 0, and it may usefully be set to any value between 0 and the duration of the buffer. IfloopStart
is less than 0, looping will begin at 0. IfloopStart
is greater than the duration of the buffer, looping will begin at the end of the buffer. playbackRate
, of type AudioParam, readonly-
The speed at which to render the audio stream. This is a compound parameter with
detune
to form a computedPlaybackRate.Parameter Value Notes defaultValue
1 minValue
most-negative-single-float Approximately -3.4028235e38 maxValue
most-positive-single-float Approximately 3.4028235e38 automationRate
" k-rate
"Has automation rate constraints
1.9.3. Methods
start(when, offset, duration)
-
Schedules a sound to playback at an exact time.
When this method is called, execute these steps:-
If this
AudioBufferSourceNode
internal slot[[source started]]
istrue
, anInvalidStateError
exception MUST be thrown. -
Check for any errors that must be thrown due to parameter constraints described below. If any exception is thrown during this step, abort those steps.
-
Set the internal slot
[[source started]]
on thisAudioBufferSourceNode
totrue
. -
Queue a control message to start the
AudioBufferSourceNode
, including the parameter values in the message. -
Acquire the contents of the
buffer
if thebuffer
has been set. -
Send a control message to the associated
AudioContext
to start running its rendering thread only when all the following conditions are met:-
The context’s
[[control thread state]]
issuspended
. -
The context is allowed to start.
-
[[suspended by user]]
flag isfalse
.
NOTE: This allows
start()
to start anAudioContext
that would otherwise not be allowed to start. -
Running a control message to start theAudioBufferSourceNode
means invoking thehandleStart()
function in the playback algorithm which follows.Arguments for the AudioBufferSourceNode.start(when, offset, duration) method. Parameter Type Nullable Optional Description when
double
✘ ✔ The when
parameter describes at what time (in seconds) the sound should start playing. It is in the same time coordinate system as theAudioContext
'scurrentTime
attribute. If 0 is passed in for this value or if the value is less than currentTime, then the sound will start playing immediately. ARangeError
exception MUST be thrown ifwhen
is negative.offset
double
✘ ✔ The offset
parameter supplies a playhead position where playback will begin. If 0 is passed in for this value, then playback will start from the beginning of the buffer. ARangeError
exception MUST be thrown ifoffset
is negative. Ifoffset
is greater thanloopEnd
,playbackRate
is positive or zero, andloop
istrue
, playback will begin atloopEnd
. Ifoffset
is greater thanloopStart
,playbackRate
is negative, andloop
istrue
, playback will begin atloopStart
.offset
is silently clamped to [0,duration
], whenstartTime
is reached, whereduration
is the value of theduration
attribute of theAudioBuffer
set to thebuffer
attribute of thisAudioBufferSourceNode
.duration
double
✘ ✔ The duration
parameter describes the duration of sound to be played, expressed as seconds of total buffer content to be output, including any whole or partial loop iterations. The units ofduration
are independent of the effects ofplaybackRate
. For example, aduration
of 5 seconds with a playback rate of 0.5 will output 5 seconds of buffer content at half speed, producing 10 seconds of audible output. ARangeError
exception MUST be thrown ifduration
is negative.Return type:undefined
-
1.9.4. AudioBufferSourceOptions
This specifies options for constructing a AudioBufferSourceNode
. All members are
optional; if not specified, the normal default is used in
constructing the node.
dictionary AudioBufferSourceOptions {AudioBuffer ?buffer ;float detune = 0;boolean loop =false ;double loopEnd = 0;double loopStart = 0;float playbackRate = 1; };
1.9.4.1. Dictionary AudioBufferSourceOptions
Members
buffer
, of type AudioBuffer, nullable-
The audio asset to be played. This is equivalent to assigning
buffer
to thebuffer
attribute of theAudioBufferSourceNode
. detune
, of type float, defaulting to0
-
The initial value for the
detune
AudioParam. loop
, of type boolean, defaulting tofalse
-
The initial value for the
loop
attribute. loopEnd
, of type double, defaulting to0
-
The initial value for the
loopEnd
attribute. loopStart
, of type double, defaulting to0
-
The initial value for the
loopStart
attribute. playbackRate
, of type float, defaulting to1
-
The initial value for the
playbackRate
AudioParam.
1.9.5. Looping
This section is non-normative. Please see the playback algorithm for normative requirements.
Setting the loop
attribute to true causes playback of the region of the buffer
defined by the endpoints loopStart
and loopEnd
to continue indefinitely, once
any part of the looped region has been played. While loop
remains true,
looped playback will continue until one of the following occurs:
-
stop()
is called, -
the scheduled stop time has been reached,
-
the
duration
has been exceeded, ifstart()
was called with aduration
value.
The body of the loop is considered to occupy a region from loopStart
up to, but
not including, loopEnd
. The direction of playback of
the looped region respects the sign of the node’s playback rate.
For positive playback rates, looping occurs from loopStart
to loopEnd
; for negative rates, looping
occurs from loopEnd
to loopStart
.
Looping does not affect the interpretation of the offset
argument of start()
. Playback always
starts at the requested offset, and looping only begins once the
body of the loop is encountered during playback.
The effective loop start and end points are required to lie within
the range of zero and the buffer duration, as specified in the
algorithm below. loopEnd
is further constrained to be at
or after loopStart
. If
any of these constraints are violated, the loop is considered to
include the entire buffer contents.
Loop endpoints have subsample accuracy. When endpoints do not fall on exact sample frame offsets, or when the playback rate is not equal to 1, playback of the loop is interpolated to splice the beginning and end of the loop together just as if the looped audio occurred in sequential, non-looped regions of the buffer.
Loop-related properties may be varied during playback of the buffer, and in general take effect on the next rendering quantum. The exact results are defined by the normative playback algorithm which follows.
The default values of the loopStart
and loopEnd
attributes are both 0. Since a loopEnd
value of zero
is equivalent to the length of the buffer, the default endpoints
cause the entire buffer to be included in the loop.
Note that the values of the loop endpoints are expressed as time
offsets in terms of the sample rate of the buffer, meaning that
these values are independent of the node’s playbackRate
parameter which can vary
dynamically during the course of playback.
1.9.6. Playback of AudioBuffer Contents
This normative section specifies the playback of the contents of the buffer, accounting for the fact that playback is influenced by the following factors working in combination:
-
A starting offset, which can be expressed with sub-sample precision.
-
Loop points, which can be expressed with sub-sample precision and can vary dynamically during playback.
-
Playback rate and detuning parameters, which combine to yield a single computedPlaybackRate that can assume finite values which may be positive or negative.
The algorithm to be followed internally to generate output from an AudioBufferSourceNode
conforms to the following principles:
-
Resampling of the buffer may be performed arbitrarily by the UA at any desired point to increase the efficiency or quality of the output.
-
Sub-sample start offsets or loop points may require additional interpolation between sample frames.
-
The playback of a looped buffer should behave identically to an unlooped buffer containing consecutive occurrences of the looped audio content, excluding any effects from interpolation.
The description of the algorithm is as follows:
let buffer; // AudioBuffer employed by this node let context; // AudioContext employed by this node // The following variables capture attribute and AudioParam values for the node. // They are updated on a k-rate basis, prior to each invocation of process(). let loop; let detune; let loopStart; let loopEnd; let playbackRate; // Variables for the node’s playback parameters let start= 0 , offset= 0 , duration= Infinity ; // Set by start() let stop= Infinity ; // Set by stop() // Variables for tracking node’s playback state let bufferTime= 0 , started= false , enteredLoop= false ; let bufferTimeElapsed= 0 ; let dt= 1 / context. sampleRate; // Handle invocation of start method call function handleStart( when, pos, dur) { if ( arguments. length>= 1 ) { start= when; } offset= pos; if ( arguments. length>= 3 ) { duration= dur; } } // Handle invocation of stop method call function handleStop( when) { if ( arguments. length>= 1 ) { stop= when; } else { stop= context. currentTime; } } // Interpolate a multi-channel signal value for some sample frame. // Returns an array of signal values. function playbackSignal( position) { /* This function provides the playback signal function for buffer, which is a function that maps from a playhead position to a set of output signal values, one for each output channel. If |position| corresponds to the location of an exact sample frame in the buffer, this function returns that frame. Otherwise, its return value is determined by a UA-supplied algorithm that interpolates sample frames in the neighborhood of |position|. If |position| is greater than or equal to |loopEnd| and there is no subsequent sample frame in buffer, then interpolation should be based on the sequence of subsequent frames beginning at |loopStart|. */ ... } // Generate a single render quantum of audio to be placed // in the channel arrays defined by output. Returns an array // of |numberOfFrames| sample frames to be output. function process( numberOfFrames) { let currentTime= context. currentTime; // context time of next rendered frame const output= []; // accumulates rendered sample frames // Combine the two k-rate parameters affecting playback rate const computedPlaybackRate= playbackRate* Math. pow( 2 , detune/ 1200 ); // Determine loop endpoints as applicable let actualLoopStart, actualLoopEnd; if ( loop&& buffer!= null ) { if ( loopStart>= 0 && loopEnd> 0 && loopStart< loopEnd) { actualLoopStart= loopStart; actualLoopEnd= Math. min( loopEnd, buffer. duration); } else { actualLoopStart= 0 ; actualLoopEnd= buffer. duration; } } else { // If the loop flag is false, remove any record of the loop having been entered enteredLoop= false ; } // Handle null buffer case if ( buffer== null ) { stop= currentTime; // force zero output for all time } // Render each sample frame in the quantum for ( let index= 0 ; index< numberOfFrames; index++ ) { // Check that currentTime and bufferTimeElapsed are // within allowable range for playback if ( currentTime< start|| currentTime>= stop|| bufferTimeElapsed>= duration) { output. push( 0 ); // this sample frame is silent currentTime+= dt; continue ; } if ( ! started) { // Take note that buffer has started playing and get initial // playhead position. if ( loop&& computedPlaybackRate>= 0 && offset>= actualLoopEnd) { offset= actualLoopEnd; } if ( computedPlaybackRate< 0 && loop&& offset< actualLoopStart) { offset= actualLoopStart; } bufferTime= offset; started= true ; } // Handle loop-related calculations if ( loop) { // Determine if looped portion has been entered for the first time if ( ! enteredLoop) { if ( offset< actualLoopEnd&& bufferTime>= actualLoopStart) { // playback began before or within loop, and playhead is // now past loop start enteredLoop= true ; } if ( offset>= actualLoopEnd&& bufferTime< actualLoopEnd) { // playback began after loop, and playhead is now prior // to the loop end enteredLoop= true ; } } // Wrap loop iterations as needed. Note that enteredLoop // may become true inside the preceding conditional. if ( enteredLoop) { while ( bufferTime>= actualLoopEnd) { bufferTime-= actualLoopEnd- actualLoopStart; } while ( bufferTime< actualLoopStart) { bufferTime+= actualLoopEnd- actualLoopStart; } } } if ( bufferTime>= 0 && bufferTime< buffer. duration) { output. push( playbackSignal( bufferTime)); } else { output. push( 0 ); // past end of buffer, so output silent frame } bufferTime+= dt* computedPlaybackRate; bufferTimeElapsed+= dt* computedPlaybackRate; currentTime+= dt; } // End of render quantum loop if ( currentTime>= stop) { // End playback state of this node. No further invocations of process() // will occur. Schedule a change to set the number of output channels to 1. } return output; }
The following non-normative figures illustrate the behavior of the algorithm in assorted key scenarios. Dynamic resampling of the buffer is not considered, but as long as the times of loop positions are not changed this does not materially affect the resulting playback. In all figures, the following conventions apply:
-
context sample rate is 1000 Hz
-
AudioBuffer
content is shown with the first sample frame at the x origin. -
output signals are shown with the sample frame located at time
start
at the x origin. -
linear interpolation is depicted throughout, although a UA could employ other interpolation techniques.
-
the
duration
values noted in the figures refer to thebuffer
, not arguments tostart()
This figure illustrates basic playback of a buffer, with a simple loop that ends after the last sample frame in the buffer:
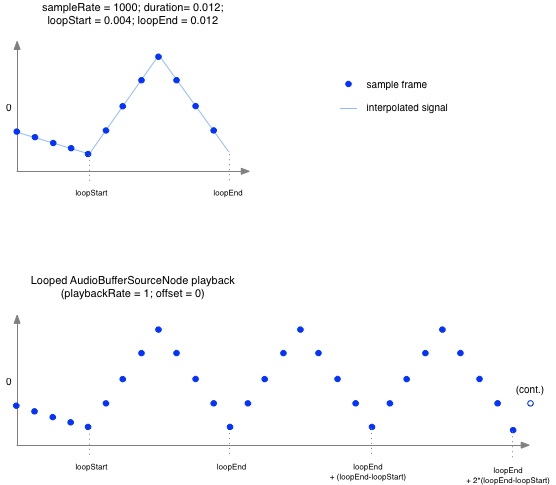
AudioBufferSourceNode
basic playback This figure illustrates playbackRate
interpolation,
showing half-speed playback of buffer contents in which every other
output sample frame is interpolated. Of particular note is the last
sample frame in the looped output, which is interpolated using the
loop start point:
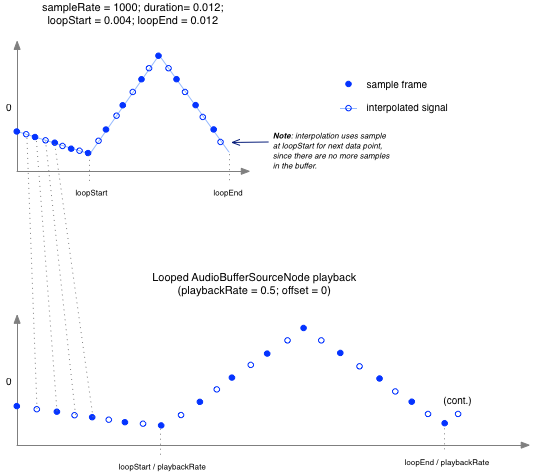
AudioBufferSourceNode
playbackRate interpolation This figure illustrates sample rate interpolation, showing playback of a buffer whose sample rate is 50% of the context sample rate, resulting in a computed playback rate of 0.5 that corrects for the difference in sample rate between the buffer and the context. The resulting output is the same as the preceding example, but for different reasons.
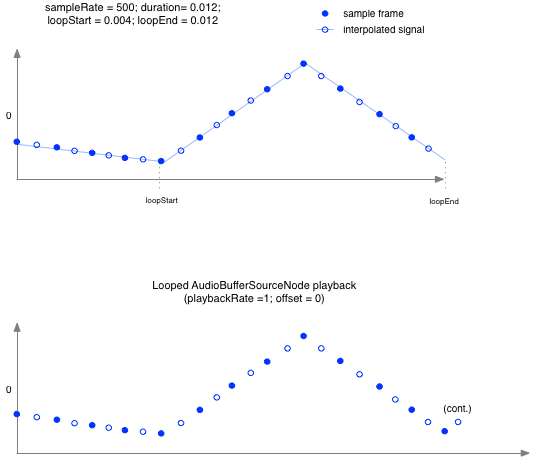
AudioBufferSourceNode
sample rate interpolation. This figure illustrates subsample offset playback, in which the offset within the buffer begins at exactly half a sample frame. Consequently, every output frame is interpolated:
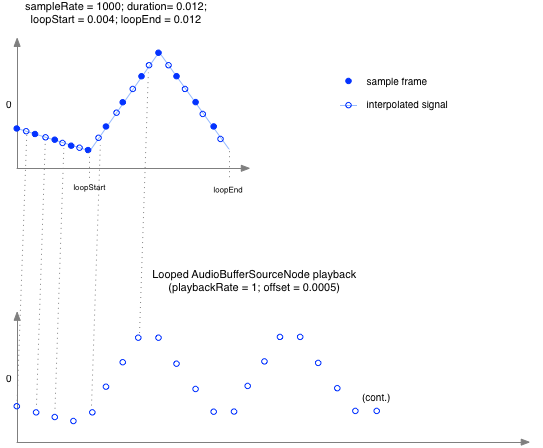
AudioBufferSourceNode
subsample offset playback This figure illustrates subsample loop playback, showing how fractional frame offsets in the loop endpoints map to interpolated data points in the buffer that respect these offsets as if they were references to exact sample frames:
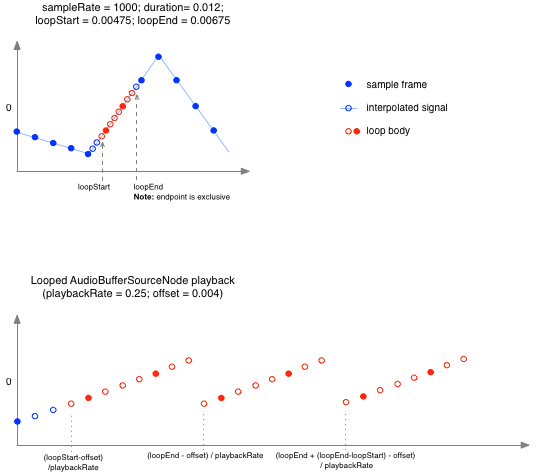
AudioBufferSourceNode
subsample loop playback 1.10. The AudioDestinationNode
Interface
This is an AudioNode
representing the final audio
destination and is what the user will ultimately hear. It can often
be considered as an audio output device which is connected to
speakers. All rendered audio to be heard will be routed to this node,
a "terminal" node in the AudioContext
's routing
graph. There is only a single AudioDestinationNode per AudioContext
, provided through the destination
attribute of AudioContext
.
The output of a AudioDestinationNode
is produced
by summing its input, allowing to
capture the output of an AudioContext
into, for
example, a MediaStreamAudioDestinationNode
, or a MediaRecorder
(described in [mediastream-recording]).
The AudioDestinationNode
can be either the destination of an AudioContext
or OfflineAudioContext
, and the channel
properties depend on what the context is.
For an AudioContext
, the defaults are
Property | Value | Notes |
---|---|---|
numberOfInputs
| 1 | |
numberOfOutputs
| 1 | |
channelCount
| 2 | |
channelCountMode
| "explicit "
| |
channelInterpretation
| "speakers "
| |
tail-time | No |
The channelCount
can be set to any
value less than or equal to maxChannelCount
. An IndexSizeError
exception MUST be thrown
if this value is not within the valid range. Giving a concrete
example, if the audio hardware supports 8-channel output, then we may
set channelCount
to 8, and render 8
channels of output.
For an OfflineAudioContext
, the defaults are
Property | Value | Notes |
---|---|---|
numberOfInputs
| 1 | |
numberOfOutputs
| 1 | |
channelCount
| numberOfChannels | |
channelCountMode
| "explicit "
| |
channelInterpretation
| "speakers "
| |
tail-time | No |
where numberOfChannels
is the number of channels
specified when constructing the OfflineAudioContext
. This
value may not be changed; a NotSupportedError
exception MUST be thrown if channelCount
is changed to a
different value.
[Exposed =Window ]interface AudioDestinationNode :AudioNode {readonly attribute unsigned long maxChannelCount ; };
1.10.1. Attributes
maxChannelCount
, of type unsigned long, readonly-
The maximum number of channels that the
channelCount
attribute can be set to. AnAudioDestinationNode
representing the audio hardware end-point (the normal case) can potentially output more than 2 channels of audio if the audio hardware is multi-channel.maxChannelCount
is the maximum number of channels that this hardware is capable of supporting.
1.11. The AudioListener
Interface
This interface represents the position and orientation of the person
listening to the audio scene. All PannerNode
objects spatialize in relation to the BaseAudioContext
's listener
. See § 6 Spatialization/Panning for more details about spatialization.
The positionX
, positionY
, and positionZ
parameters represent
the location of the listener in 3D Cartesian coordinate space. PannerNode
objects use this position relative to
individual audio sources for spatialization.
The forwardX
, forwardY
, and forwardZ
parameters represent a
direction vector in 3D space. Both a forward
vector and
an up
vector are used to determine the orientation of
the listener. In simple human terms, the forward
vector
represents which direction the person’s nose is pointing. The up
vector represents the direction the top of a person’s
head is pointing. These two vectors are expected to be linearly
independent. For normative requirements of how these values are to be
interpreted, see the § 6 Spatialization/Panning section.
[Exposed =Window ]interface AudioListener {readonly attribute AudioParam positionX ;readonly attribute AudioParam positionY ;readonly attribute AudioParam positionZ ;readonly attribute AudioParam forwardX ;readonly attribute AudioParam forwardY ;readonly attribute AudioParam forwardZ ;readonly attribute AudioParam upX ;readonly attribute AudioParam upY ;readonly attribute AudioParam upZ ;undefined setPosition (float ,
x float ,
y float );
z undefined setOrientation (float ,
x float ,
y float ,
z float ,
xUp float ,
yUp float ); };
zUp
1.11.1. Attributes
forwardX
, of type AudioParam, readonly-
Sets the x coordinate component of the forward direction the listener is pointing in 3D Cartesian coordinate space.
Parameter Value Notes defaultValue
0 minValue
most-negative-single-float Approximately -3.4028235e38 maxValue
most-positive-single-float Approximately 3.4028235e38 automationRate
" a-rate
" forwardY
, of type AudioParam, readonly-
Sets the y coordinate component of the forward direction the listener is pointing in 3D Cartesian coordinate space.
Parameter Value Notes defaultValue
0 minValue
most-negative-single-float Approximately -3.4028235e38 maxValue
most-positive-single-float Approximately 3.4028235e38 automationRate
" a-rate
" forwardZ
, of type AudioParam, readonly-
Sets the z coordinate component of the forward direction the listener is pointing in 3D Cartesian coordinate space.
Parameter Value Notes defaultValue
-1 minValue
most-negative-single-float Approximately -3.4028235e38 maxValue
most-positive-single-float Approximately 3.4028235e38 automationRate
" a-rate
" positionX
, of type AudioParam, readonly-
Sets the x coordinate position of the audio listener in a 3D Cartesian coordinate space.
Parameter Value Notes defaultValue
0 minValue
most-negative-single-float Approximately -3.4028235e38 maxValue
most-positive-single-float Approximately 3.4028235e38 automationRate
" a-rate
" positionY
, of type AudioParam, readonly-
Sets the y coordinate position of the audio listener in a 3D Cartesian coordinate space.
Parameter Value Notes defaultValue
0 minValue
most-negative-single-float Approximately -3.4028235e38 maxValue
most-positive-single-float Approximately 3.4028235e38 automationRate
" a-rate
" positionZ
, of type AudioParam, readonly-
Sets the z coordinate position of the audio listener in a 3D Cartesian coordinate space.
Parameter Value Notes defaultValue
0 minValue
most-negative-single-float Approximately -3.4028235e38 maxValue
most-positive-single-float Approximately 3.4028235e38 automationRate
" a-rate
" upX
, of type AudioParam, readonly-
Sets the x coordinate component of the up direction the listener is pointing in 3D Cartesian coordinate space.
Parameter Value Notes defaultValue
0 minValue
most-negative-single-float Approximately -3.4028235e38 maxValue
most-positive-single-float Approximately 3.4028235e38 automationRate
" a-rate
" upY
, of type AudioParam, readonly-
Sets the y coordinate component of the up direction the listener is pointing in 3D Cartesian coordinate space.
Parameter Value Notes defaultValue
1 minValue
most-negative-single-float Approximately -3.4028235e38 maxValue
most-positive-single-float Approximately 3.4028235e38 automationRate
" a-rate
" upZ
, of type AudioParam, readonly-
Sets the z coordinate component of the up direction the listener is pointing in 3D Cartesian coordinate space.
Parameter Value Notes defaultValue
0 minValue
most-negative-single-float Approximately -3.4028235e38 maxValue
most-positive-single-float Approximately 3.4028235e38 automationRate
" a-rate
"
1.11.2. Methods
setOrientation(x, y, z, xUp, yUp, zUp)
-
This method is DEPRECATED. It is equivalent to setting
forwardX
.value
,forwardY
.value
,forwardZ
.value
,upX
.value
,upY
.value
, andupZ
.value
directly with the givenx
,y
,z
,xUp
,yUp
, andzUp
values, respectively.Consequently, if any of the
forwardX
,forwardY
,forwardZ
,upX
,upY
andupZ
AudioParam
s have an automation curve set usingsetValueCurveAtTime()
at the time this method is called, aNotSupportedError
MUST be thrown.setOrientation()
describes which direction the listener is pointing in the 3D cartesian coordinate space. Both a forward vector and an up vector are provided. In simple human terms, the forward vector represents which direction the person’s nose is pointing. The up vector represents the direction the top of a person’s head is pointing. These two vectors are expected to be linearly independent. For normative requirements of how these values are to be interpreted, see the § 6 Spatialization/Panning.The
x
,y
, andz
parameters represent a forward direction vector in 3D space, with the default value being (0,0,-1).The
xUp
,yUp
, andzUp
parameters represent an up direction vector in 3D space, with the default value being (0,1,0).Arguments for the AudioListener.setOrientation() method. Parameter Type Nullable Optional Description x
float
✘ ✘ forward x direction fo the AudioListener
y
float
✘ ✘ forward y direction fo the AudioListener
z
float
✘ ✘ forward z direction fo the AudioListener
xUp
float
✘ ✘ up x direction fo the AudioListener
yUp
float
✘ ✘ up y direction fo the AudioListener
zUp
float
✘ ✘ up z direction fo the AudioListener
Return type:undefined
setPosition(x, y, z)
-
This method is DEPRECATED. It is equivalent to setting
positionX
.value
,positionY
.value
, andpositionZ
.value
directly with the givenx
,y
, andz
values, respectively.Consequently, any of the
positionX
,positionY
, andpositionZ
AudioParam
s for thisAudioListener
have an automation curve set usingsetValueCurveAtTime()
at the time this method is called, aNotSupportedError
MUST be thrown.setPosition()
sets the position of the listener in a 3D cartesian coordinate space.PannerNode
objects use this position relative to individual audio sources for spatialization.The
x
,y
, andz
parameters represent the coordinates in 3D space.The default value is (0,0,0).
Arguments for the AudioListener.setPosition() method. Parameter Type Nullable Optional Description x
float
✘ ✘ x-coordinate of the position of the AudioListener
y
float
✘ ✘ y-coordinate of the position of the AudioListener
z
float
✘ ✘ z-coordinate of the position of the AudioListener
1.11.3. Processing
Because AudioListener
's parameters can be connected with AudioNode
s and
they can also affect the output of PannerNode
s in the same graph, the node
ordering algorithm should take the AudioListener
into consideration when
computing the order of processing. For this reason, all the PannerNode
s in
the graph have the AudioListener
as input.
1.12. The AudioProcessingEvent
Interface - DEPRECATED
This is an Event
object which is dispatched to ScriptProcessorNode
nodes. It will be removed
when the ScriptProcessorNode is removed, as the replacement AudioWorkletNode
uses a different approach.
The event handler processes audio from the input (if any) by
accessing the audio data from the inputBuffer
attribute.
The audio data which is the result of the processing (or the
synthesized data if there are no inputs) is then placed into the outputBuffer
.
[Exposed =Window ]interface AudioProcessingEvent :Event {(
constructor DOMString ,
type AudioProcessingEventInit );
eventInitDict readonly attribute double playbackTime ;readonly attribute AudioBuffer inputBuffer ;readonly attribute AudioBuffer outputBuffer ; };
1.12.1. Attributes
inputBuffer
, of type AudioBuffer, readonly-
An AudioBuffer containing the input audio data. It will have a number of channels equal to the
numberOfInputChannels
parameter of the createScriptProcessor() method. This AudioBuffer is only valid while in the scope of theaudioprocess
event handler functions. Its values will be meaningless outside of this scope. outputBuffer
, of type AudioBuffer, readonly-
An AudioBuffer where the output audio data MUST be written. It will have a number of channels equal to the
numberOfOutputChannels
parameter of the createScriptProcessor() method. Script code within the scope of theaudioprocess
event handler functions are expected to modify theFloat32Array
arrays representing channel data in this AudioBuffer. Any script modifications to this AudioBuffer outside of this scope will not produce any audible effects. playbackTime
, of type double, readonly-
The time when the audio will be played in the same time coordinate system as the
AudioContext
'scurrentTime
.
1.12.2. AudioProcessingEventInit
dictionary AudioProcessingEventInit :EventInit {required double playbackTime ;required AudioBuffer inputBuffer ;required AudioBuffer outputBuffer ; };
1.12.2.1. Dictionary AudioProcessingEventInit
Members
inputBuffer
, of type AudioBuffer-
Value to be assigned to the
inputBuffer
attribute of the event. outputBuffer
, of type AudioBuffer-
Value to be assigned to the
outputBuffer
attribute of the event. playbackTime
, of type double-
Value to be assigned to the
playbackTime
attribute of the event.
1.13. The BiquadFilterNode
Interface
BiquadFilterNode
is an AudioNode
processor implementing very common
low-order filters.
Low-order filters are the building blocks of basic tone controls
(bass, mid, treble), graphic equalizers, and more advanced filters.
Multiple BiquadFilterNode
filters can be combined
to form more complex filters. The filter parameters such as frequency
can be
changed over time for filter sweeps, etc. Each BiquadFilterNode
can be configured as one of a
number of common filter types as shown in the IDL below. The default
filter type is "lowpass"
.
Both frequency
and detune
form
a compound parameter and are both a-rate. They are used
together to determine a computedFrequency value:
computedFrequency(t) = frequency(t) * pow(2, detune(t) / 1200)
The nominal range for this compound parameter is [0, Nyquist frequency].
Property | Value | Notes |
---|---|---|
numberOfInputs
| 1 | |
numberOfOutputs
| 1 | |
channelCount
| 2 | |
channelCountMode
| "max "
| |
channelInterpretation
| "speakers "
| |
tail-time | Yes | Continues to output non-silent audio with zero input. Since this is an IIR filter, the filter produces non-zero input forever, but in practice, this can be limited after some finite time where the output is sufficiently close to zero. The actual time depends on the filter coefficients. |
The number of channels of the output always equals the number of channels of the input.
enum {
BiquadFilterType "lowpass" ,"highpass" ,"bandpass" ,"lowshelf" ,"highshelf" ,"peaking" ,"notch" ,"allpass" };
Enum value | Description |
---|---|
"lowpass "
|
A lowpass
filter allows frequencies below the cutoff frequency to
pass through and attenuates frequencies above the cutoff. It
implements a standard second-order resonant lowpass filter with
12dB/octave rolloff.
|
"highpass "
|
A highpass
filter is the opposite of a lowpass filter. Frequencies
above the cutoff frequency are passed through, but frequencies
below the cutoff are attenuated. It implements a standard
second-order resonant highpass filter with 12dB/octave rolloff.
|
"bandpass "
|
A bandpass
filter allows a range of frequencies to pass through and
attenuates the frequencies below and above this frequency
range. It implements a second-order bandpass filter.
|
"lowshelf "
|
The lowshelf filter allows all frequencies through, but adds a
boost (or attenuation) to the lower frequencies. It implements
a second-order lowshelf filter.
|
"highshelf "
|
The highshelf filter is the opposite of the lowshelf filter and
allows all frequencies through, but adds a boost to the higher
frequencies. It implements a second-order highshelf filter
|
"peaking "
|
The peaking filter allows all frequencies through, but adds a
boost (or attenuation) to a range of frequencies.
|
"notch "
|
The notch filter (also known as a band-stop or
band-rejection filter) is the opposite of a bandpass
filter. It allows all frequencies through, except for a set of
frequencies.
|
"allpass "
|
An allpass filter allows all frequencies through, but changes
the phase relationship between the various frequencies. It
implements a second-order allpass filter
|
All attributes of the BiquadFilterNode
are a-rate AudioParam
s.
[Exposed =Window ]interface BiquadFilterNode :AudioNode {(
constructor BaseAudioContext ,
context optional BiquadFilterOptions = {});
options attribute BiquadFilterType type ;readonly attribute AudioParam frequency ;readonly attribute AudioParam detune ;readonly attribute AudioParam Q ;readonly attribute AudioParam gain ;undefined getFrequencyResponse (Float32Array ,
frequencyHz Float32Array ,
magResponse Float32Array ); };
phaseResponse
1.13.1. Constructors
BiquadFilterNode(context, options)
-
When the constructor is called with a
BaseAudioContext
c and an option object option, the user agent MUST initialize the AudioNode this, with context and options as arguments.Arguments for the BiquadFilterNode.constructor() method. Parameter Type Nullable Optional Description context
BaseAudioContext
✘ ✘ The BaseAudioContext
this newBiquadFilterNode
will be associated with.options
BiquadFilterOptions
✘ ✔ Optional initial parameter value for this BiquadFilterNode
.
1.13.2. Attributes
Q
, of type AudioParam, readonly-
The Q factor of the filter.
For
lowpass
andhighpass
filters theQ
value is interpreted to be in dB. For these filters the nominal range is \([-Q_{lim}, Q_{lim}]\) where \(Q_{lim}\) is the largest value for which \(10^{Q/20}\) does not overflow. This is approximately \(770.63678\).For the
bandpass
,notch
,allpass
, andpeaking
filters, this value is a linear value. The value is related to the bandwidth of the filter and hence should be a positive value. The nominal range is \([0, 3.4028235e38]\), the upper limit being the most-positive-single-float.This is not used for the
lowshelf
andhighshelf
filters.Parameter Value Notes defaultValue
1 minValue
most-negative-single-float Approximately -3.4028235e38, but see above for the actual limits for different filters maxValue
most-positive-single-float Approximately 3.4028235e38, but see above for the actual limits for different filters automationRate
" a-rate
" detune
, of type AudioParam, readonly-
A detune value, in cents, for the frequency. It forms a compound parameter with
frequency
to form the computedFrequency.Parameter Value Notes defaultValue
0 minValue
\(\approx -153600\) maxValue
\(\approx 153600\) This value is approximately \(1200\ \log_2 \mathrm{FLT\_MAX}\) where FLT_MAX is the largest float
value.automationRate
" a-rate
" frequency
, of type AudioParam, readonly-
The frequency at which the
BiquadFilterNode
will operate, in Hz. It forms a compound parameter withdetune
to form the computedFrequency.Parameter Value Notes defaultValue
350 minValue
0 maxValue
Nyquist frequency automationRate
" a-rate
" gain
, of type AudioParam, readonly-
The gain of the filter. Its value is in dB units. The gain is only used for
lowshelf
,highshelf
, andpeaking
filters.Parameter Value Notes defaultValue
0 minValue
most-negative-single-float Approximately -3.4028235e38 maxValue
\(\approx 1541\) This value is approximately \(40\ \log_{10} \mathrm{FLT\_MAX}\) where FLT_MAX is the largest float
value.automationRate
" a-rate
" type
, of type BiquadFilterType-
The type of this
BiquadFilterNode
. Its default value is "lowpass
". The exact meaning of the other parameters depend on the value of thetype
attribute.
1.13.3. Methods
getFrequencyResponse(frequencyHz, magResponse, phaseResponse)
-
Given the
[[current value]]
from each of the filter parameters, synchronously calculates the frequency response for the specified frequencies. The three parameters MUST beFloat32Array
s of the same length, or anInvalidAccessError
MUST be thrown.The frequency response returned MUST be computed with the
AudioParam
sampled for the current processing block.Arguments for the BiquadFilterNode.getFrequencyResponse() method. Parameter Type Nullable Optional Description frequencyHz
Float32Array
✘ ✘ This parameter specifies an array of frequencies, in Hz, at which the response values will be calculated. magResponse
Float32Array
✘ ✘ This parameter specifies an output array receiving the linear magnitude response values. If a value in the frequencyHz
parameter is not within [0, sampleRate/2], wheresampleRate
is the value of thesampleRate
property of theAudioContext
, the corresponding value at the same index of themagResponse
array MUST beNaN
.phaseResponse
Float32Array
✘ ✘ This parameter specifies an output array receiving the phase response values in radians. If a value in the frequencyHz
parameter is not within [0; sampleRate/2], wheresampleRate
is the value of thesampleRate
property of theAudioContext
, the corresponding value at the same index of thephaseResponse
array MUST beNaN
.Return type:undefined
1.13.4. BiquadFilterOptions
This specifies the options to be used when constructing a BiquadFilterNode
. All members are optional; if
not specified, the normal default values are used to construct the
node.
dictionary BiquadFilterOptions :AudioNodeOptions {BiquadFilterType type = "lowpass";float Q = 1;float detune = 0;float frequency = 350;float gain = 0; };
1.13.4.1. Dictionary BiquadFilterOptions
Members
Q
, of type float, defaulting to1
-
The desired initial value for
Q
. detune
, of type float, defaulting to0
-
The desired initial value for
detune
. frequency
, of type float, defaulting to350
-
The desired initial value for
frequency
. gain
, of type float, defaulting to0
-
The desired initial value for
gain
. type
, of type BiquadFilterType, defaulting to"lowpass"
-
The desired initial type of the filter.
1.13.5. Filters Characteristics
There are multiple ways of implementing the type of filters
available through the BiquadFilterNode
each
having very different characteristics. The formulas in this section
describe the filters that a conforming implementation MUST
implement, as they determine the characteristics of the different
filter types. They are inspired by formulas found in the Audio EQ Cookbook.
The BiquadFilterNode
processes audio with a transfer function of
$$ H(z) = \frac{\frac{b_0}{a_0} + \frac{b_1}{a_0}z^{-1} + \frac{b_2}{a_0}z^{-2}} {1+\frac{a_1}{a_0}z^{-1}+\frac{a_2}{a_0}z^{-2}} $$
which is equivalent to a time-domain equation of:
$$ a_0 y(n) + a_1 y(n-1) + a_2 y(n-2) = b_0 x(n) + b_1 x(n-1) + b_2 x(n-2) $$
The initial filter state is 0.
Note: While fixed filters are stable, it is possible to create
unstable biquad filters using automations of AudioParam
s. It is
the developers responsibility to manage this.
Note: The UA may produce a warning to notify the user that NaN values have occurred in the filter state. This is usually indicative of an unstable filter.
The coefficients in the transfer function above are different for
each node type. The following intermediate variables are necessary for
their computation, based on the computedValue of the AudioParam
s of the BiquadFilterNode
.
-
Let \(F_s\) be the value of the
sampleRate
attribute for thisAudioContext
. -
Let \(f_0\) be the value of the computedFrequency.
-
Let \(G\) be the value of the
gain
AudioParam
. -
Let \(Q\) be the value of the
Q
AudioParam
. -
Finally let
$$ \begin{align*} A &= 10^{\frac{G}{40}} \\ \omega_0 &= 2\pi\frac{f_0}{F_s} \\ \alpha_Q &= \frac{\sin\omega_0}{2Q} \\ \alpha_{Q_{dB}} &= \frac{\sin\omega_0}{2 \cdot 10^{Q/20}} \\ S &= 1 \\ \alpha_S &= \frac{\sin\omega_0}{2}\sqrt{\left(A+\frac{1}{A}\right)\left(\frac{1}{S}-1\right)+2} \end{align*} $$
The six coefficients (\(b_0, b_1, b_2, a_0, a_1, a_2\)) for each filter type, are:
- "
lowpass
" -
$$ \begin{align*} b_0 &= \frac{1 - \cos\omega_0}{2} \\ b_1 &= 1 - \cos\omega_0 \\ b_2 &= \frac{1 - \cos\omega_0}{2} \\ a_0 &= 1 + \alpha_{Q_{dB}} \\ a_1 &= -2 \cos\omega_0 \\ a_2 &= 1 - \alpha_{Q_{dB}} \end{align*} $$
- "
highpass
" -
$$ \begin{align*} b_0 &= \frac{1 + \cos\omega_0}{2} \\ b_1 &= -(1 + \cos\omega_0) \\ b_2 &= \frac{1 + \cos\omega_0}{2} \\ a_0 &= 1 + \alpha_{Q_{dB}} \\ a_1 &= -2 \cos\omega_0 \\ a_2 &= 1 - \alpha_{Q_{dB}} \end{align*} $$
- "
bandpass
" -
$$ \begin{align*} b_0 &= \alpha_Q \\ b_1 &= 0 \\ b_2 &= -\alpha_Q \\ a_0 &= 1 + \alpha_Q \\ a_1 &= -2 \cos\omega_0 \\ a_2 &= 1 - \alpha_Q \end{align*} $$
- "
notch
" -
$$ \begin{align*} b_0 &= 1 \\ b_1 &= -2\cos\omega_0 \\ b_2 &= 1 \\ a_0 &= 1 + \alpha_Q \\ a_1 &= -2 \cos\omega_0 \\ a_2 &= 1 - \alpha_Q \end{align*} $$
- "
allpass
" -
$$ \begin{align*} b_0 &= 1 - \alpha_Q \\ b_1 &= -2\cos\omega_0 \\ b_2 &= 1 + \alpha_Q \\ a_0 &= 1 + \alpha_Q \\ a_1 &= -2 \cos\omega_0 \\ a_2 &= 1 - \alpha_Q \end{align*} $$
- "
peaking
" -
$$ \begin{align*} b_0 &= 1 + \alpha_Q\, A \\ b_1 &= -2\cos\omega_0 \\ b_2 &= 1 - \alpha_Q\,A \\ a_0 &= 1 + \frac{\alpha_Q}{A} \\ a_1 &= -2 \cos\omega_0 \\ a_2 &= 1 - \frac{\alpha_Q}{A} \end{align*} $$
- "
lowshelf
" -
$$ \begin{align*} b_0 &= A \left[ (A+1) - (A-1) \cos\omega_0 + 2 \alpha_S \sqrt{A})\right] \\ b_1 &= 2 A \left[ (A-1) - (A+1) \cos\omega_0 )\right] \\ b_2 &= A \left[ (A+1) - (A-1) \cos\omega_0 - 2 \alpha_S \sqrt{A}) \right] \\ a_0 &= (A+1) + (A-1) \cos\omega_0 + 2 \alpha_S \sqrt{A} \\ a_1 &= -2 \left[ (A-1) + (A+1) \cos\omega_0\right] \\ a_2 &= (A+1) + (A-1) \cos\omega_0 - 2 \alpha_S \sqrt{A}) \end{align*} $$
- "
highshelf
" -
$$ \begin{align*} b_0 &= A\left[ (A+1) + (A-1)\cos\omega_0 + 2\alpha_S\sqrt{A} )\right] \\ b_1 &= -2A\left[ (A-1) + (A+1)\cos\omega_0 )\right] \\ b_2 &= A\left[ (A+1) + (A-1)\cos\omega_0 - 2\alpha_S\sqrt{A} )\right] \\ a_0 &= (A+1) - (A-1)\cos\omega_0 + 2\alpha_S\sqrt{A} \\ a_1 &= 2\left[ (A-1) - (A+1)\cos\omega_0\right] \\ a_2 &= (A+1) - (A-1)\cos\omega_0 - 2\alpha_S\sqrt{A} \end{align*} $$
1.14. The ChannelMergerNode
Interface
The ChannelMergerNode
is for use in more advanced
applications and would often be used in conjunction with ChannelSplitterNode
.
Property | Value | Notes |
---|---|---|
numberOfInputs
| see notes | Defaults to 6, but is determined by ChannelMergerOptions ,numberOfInputs or the value specified by createChannelMerger .
|
numberOfOutputs
| 1 | |
channelCount
| 1 | Has channelCount constraints |
channelCountMode
| "explicit "
| Has channelCountMode constraints |
channelInterpretation
| "speakers "
| |
tail-time | No |
This interface represents an AudioNode
for
combining channels from multiple audio streams into a single audio
stream. It has a variable number of inputs (defaulting to 6), but not
all of them need be connected. There is a single output whose audio
stream has a number of channels equal to the number of inputs when any
of the inputs is actively processing. If none of the inputs are actively processing, then output is a single channel of silence.
To merge multiple inputs into one stream, each input gets downmixed into one channel (mono) based on the specified mixing rule. An unconnected input still counts as one silent channel in the output. Changing input streams does not affect the order of output channels.
ChannelMergerNode
has
two connected stereo inputs, the first and second input will be
downmixed to mono respectively before merging. The output will be a
6-channel stream whose first two channels are be filled with the
first two (downmixed) inputs and the rest of channels will be silent.
Also the ChannelMergerNode
can be used to arrange
multiple audio streams in a certain order for the multi-channel
speaker array such as 5.1 surround set up. The merger does not
interpret the channel identities (such as left, right, etc.), but
simply combines channels in the order that they are input.
[Exposed =Window ]interface ChannelMergerNode :AudioNode {constructor (BaseAudioContext ,
context optional ChannelMergerOptions = {}); };
options
1.14.1. Constructors
ChannelMergerNode(context, options)
-
When the constructor is called with a
BaseAudioContext
c and an option object option, the user agent MUST initialize the AudioNode this, with context and options as arguments.Arguments for the ChannelMergerNode.constructor() method. Parameter Type Nullable Optional Description context
BaseAudioContext
✘ ✘ The BaseAudioContext
this newChannelMergerNode
will be associated with.options
ChannelMergerOptions
✘ ✔ Optional initial parameter value for this ChannelMergerNode
.
1.14.2. ChannelMergerOptions
dictionary ChannelMergerOptions :AudioNodeOptions {unsigned long numberOfInputs = 6; };
1.14.2.1. Dictionary ChannelMergerOptions
Members
numberOfInputs
, of type unsigned long, defaulting to6
-
The number inputs for the
ChannelMergerNode
. SeecreateChannelMerger()
for constraints on this value.
1.15. The ChannelSplitterNode
Interface
The ChannelSplitterNode
is for use in more advanced
applications and would often be used in conjunction with ChannelMergerNode
.
Property | Value | Notes |
---|---|---|
numberOfInputs
| 1 | |
numberOfOutputs
| see notes | This defaults to 6, but is otherwise determined from ChannelSplitterOptions.numberOfOutputs or the value specified by createChannelSplitter or the numberOfOutputs member of the ChannelSplitterOptions dictionary for the constructor .
|
channelCount
| numberOfOutputs
| Has channelCount constraints |
channelCountMode
| "explicit "
| Has channelCountMode constraints |
channelInterpretation
| "discrete "
| Has channelInterpretation constraints |
tail-time | No |
This interface represents an AudioNode
for
accessing the individual channels of an audio stream in the routing
graph. It has a single input, and a number of "active" outputs which
equals the number of channels in the input audio stream. For example,
if a stereo input is connected to an ChannelSplitterNode
then the number of active
outputs will be two (one from the left channel and one from the
right). There are always a total number of N outputs (determined by
the numberOfOutputs
parameter to the AudioContext
method createChannelSplitter()
), The
default number is 6 if this value is not provided. Any outputs which
are not "active" will output silence and would typically not be
connected to anything.
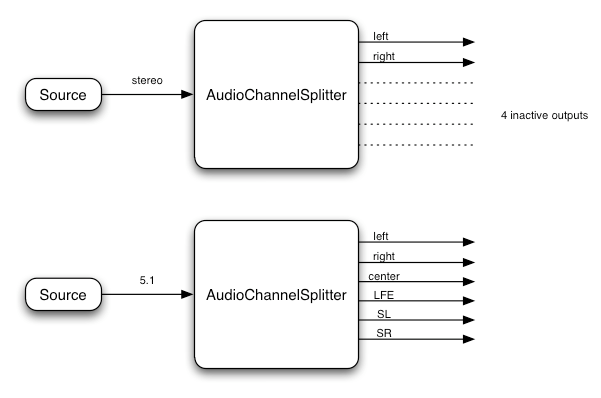
Please note that in this example, the splitter does not interpret the channel identities (such as left, right, etc.), but simply splits out channels in the order that they are input.
One application for ChannelSplitterNode
is for doing
"matrix mixing" where individual gain control of each channel is
desired.
[Exposed =Window ]interface ChannelSplitterNode :AudioNode {constructor (BaseAudioContext ,
context optional ChannelSplitterOptions = {}); };
options
1.15.1. Constructors
ChannelSplitterNode(context, options)
-
When the constructor is called with a
BaseAudioContext
c and an option object option, the user agent MUST initialize the AudioNode this, with context and options as arguments.Arguments for the ChannelSplitterNode.constructor() method. Parameter Type Nullable Optional Description context
BaseAudioContext
✘ ✘ The BaseAudioContext
this newChannelSplitterNode
will be associated with.options
ChannelSplitterOptions
✘ ✔ Optional initial parameter value for this ChannelSplitterNode
.
1.15.2. ChannelSplitterOptions
dictionary ChannelSplitterOptions :AudioNodeOptions {unsigned long numberOfOutputs = 6; };
1.15.2.1. Dictionary ChannelSplitterOptions
Members
numberOfOutputs
, of type unsigned long, defaulting to6
-
The number outputs for the
ChannelSplitterNode
. SeecreateChannelSplitter()
for constraints on this value.
1.16. The ConstantSourceNode
Interface
This interface represents a constant audio source whose output is
nominally a constant value. It is useful as a constant source node in
general and can be used as if it were a constructible AudioParam
by automating its offset
or connecting another node to it.
The single output of this node consists of one channel (mono).
Property | Value | Notes |
---|---|---|
numberOfInputs
| 0 | |
numberOfOutputs
| 1 | |
channelCount
| 2 | |
channelCountMode
| "max "
| |
channelInterpretation
| "speakers "
| |
tail-time | No |
[Exposed =Window ]interface ConstantSourceNode :AudioScheduledSourceNode {constructor (BaseAudioContext ,
context optional ConstantSourceOptions = {});
options readonly attribute AudioParam offset ; };
1.16.1. Constructors
ConstantSourceNode(context, options)
-
When the constructor is called with a
BaseAudioContext
c and an option object option, the user agent MUST initialize the AudioNode this, with context and options as arguments.Arguments for the ConstantSourceNode.constructor() method. Parameter Type Nullable Optional Description context
BaseAudioContext
✘ ✘ The BaseAudioContext
this newConstantSourceNode
will be associated with.options
ConstantSourceOptions
✘ ✔ Optional initial parameter value for this ConstantSourceNode
.
1.16.2. Attributes
offset
, of type AudioParam, readonly-
The constant value of the source.
Parameter Value Notes defaultValue
1 minValue
most-negative-single-float Approximately -3.4028235e38 maxValue
most-positive-single-float Approximately 3.4028235e38 automationRate
" a-rate
"
1.16.3. ConstantSourceOptions
This specifies options for constructing a ConstantSourceNode
. All members are optional;
if not specified, the normal defaults are used for constructing the
node.
dictionary ConstantSourceOptions {float offset = 1; };
1.16.3.1. Dictionary ConstantSourceOptions
Members
offset
, of type float, defaulting to1
-
The initial value for the
offset
AudioParam of this node.
1.17. The ConvolverNode
Interface
This interface represents a processing node which applies a linear convolution effect given an impulse response.
Property | Value | Notes |
---|---|---|
numberOfInputs
| 1 | |
numberOfOutputs
| 1 | |
channelCount
| 2 | Has channelCount constraints |
channelCountMode
| "clamped-max "
| Has channelCountMode constraints |
channelInterpretation
| "speakers "
| |
tail-time | Yes | Continues to output non-silent audio with zero input for the length of the buffer .
|
The input of this node is either mono (1 channel) or stereo (2 channels) and cannot be increased. Connections from nodes with more channels will be down-mixed appropriately.
There are channelCount constraints and channelCountMode constraints for this node. These constraints ensure that the input to the node is either mono or stereo.
[Exposed =Window ]interface ConvolverNode :AudioNode {constructor (BaseAudioContext ,
context optional ConvolverOptions = {});
options attribute AudioBuffer ?buffer ;attribute boolean normalize ; };
1.17.1. Constructors
ConvolverNode(context, options)
-
When the constructor is called with a
BaseAudioContext
context and an option object options, execute these steps:-
Set the attributes
normalize
to the inverse of the value ofdisableNormalization
. -
If
buffer
exists, set thebuffer
attribute to its value.Note: This means that the buffer will be normalized according to the value of the
normalize
attribute. -
Let o be new
AudioNodeOptions
dictionary. -
If
channelCount
exists in options, setchannelCount
on o with the same value. -
If
channelCountMode
exists in options, setchannelCountMode
on o with the same value. -
If
channelInterpretation
exists in options, setchannelInterpretation
on o with the same value. -
Initialize the AudioNode this, with c and o as argument.
Arguments for the ConvolverNode.constructor() method. Parameter Type Nullable Optional Description context
BaseAudioContext
✘ ✘ The BaseAudioContext
this newConvolverNode
will be associated with.options
ConvolverOptions
✘ ✔ Optional initial parameter value for this ConvolverNode
. -
1.17.2. Attributes
buffer
, of type AudioBuffer, nullable-
At the time when this attribute is set, the
buffer
and the state of thenormalize
attribute will be used to configure theConvolverNode
with this impulse response having the given normalization. The initial value of this attribute is null.When setting thebuffer attribute
, execute the following steps synchronously:-
If the buffer
number of channels
is not 1, 2, 4, or if thesample-rate
of the buffer is not the same as thesample-rate
of its associatedBaseAudioContext
, aNotSupportedError
MUST be thrown. -
Acquire the content of the
AudioBuffer
.
Note: If the
buffer
is set to an new buffer, audio may glitch. If this is undesirable, it is recommended to create a newConvolverNode
to replace the old, possibly cross-fading between the two.Note: The
ConvolverNode
produces a mono output only in the single case where there is a single input channel and a single-channelbuffer
. In all other cases, the output is stereo. In particular, when thebuffer
has four channels and there are two input channels, theConvolverNode
performs matrix "true" stereo convolution. For normative information please see the channel configuration diagrams -
normalize
, of type boolean-
Controls whether the impulse response from the buffer will be scaled by an equal-power normalization when the
buffer
atttribute is set. Its default value istrue
in order to achieve a more uniform output level from the convolver when loaded with diverse impulse responses. Ifnormalize
is set tofalse
, then the convolution will be rendered with no pre-processing/scaling of the impulse response. Changes to this value do not take effect until the next time thebuffer
attribute is set.If the
normalize
attribute is false when thebuffer
attribute is set then theConvolverNode
will perform a linear convolution given the exact impulse response contained within thebuffer
.Otherwise, if the
normalize
attribute is true when thebuffer
attribute is set then theConvolverNode
will first perform a scaled RMS-power analysis of the audio data contained withinbuffer
to calculate a normalizationScale given this algorithm:function calculateNormalizationScale( buffer) { const GainCalibration= 0.00125 ; const GainCalibrationSampleRate= 44100 ; const MinPower= 0.000125 ; // Normalize by RMS power. const numberOfChannels= buffer. numberOfChannels; const length= buffer. length; let power= 0 ; for ( let i= 0 ; i< numberOfChannels; i++ ) { let channelPower= 0 ; const channelData= buffer. getChannelData( i); for ( let j= 0 ; j< length; j++ ) { const sample= channelData[ j]; channelPower+= sample* sample; } power+= channelPower; } power= Math. sqrt( power/ ( numberOfChannels* length)); // Protect against accidental overload. if ( ! isFinite( power) || isNaN( power) || power< MinPower) power= MinPower; let scale= 1 / power; // Calibrate to make perceived volume same as unprocessed. scale*= GainCalibration; // Scale depends on sample-rate. if ( buffer. sampleRate) scale*= GainCalibrationSampleRate/ buffer. sampleRate; // True-stereo compensation. if ( numberOfChannels== 4 ) scale*= 0.5 ; return scale; } During processing, the ConvolverNode will then take this calculated normalizationScale value and multiply it by the result of the linear convolution resulting from processing the input with the impulse response (represented by the
buffer
) to produce the final output. Or any mathematically equivalent operation may be used, such as pre-multiplying the input by normalizationScale, or pre-multiplying a version of the impulse-response by normalizationScale.
1.17.3. ConvolverOptions
The specifies options for constructing a ConvolverNode
. All members are optional; if not
specified, the node is contructing using the normal defaults.
dictionary ConvolverOptions :AudioNodeOptions {AudioBuffer ?buffer ;boolean disableNormalization =false ; };
1.17.3.1. Dictionary ConvolverOptions
Members
buffer
, of type AudioBuffer, nullable-
The desired buffer for the
ConvolverNode
. This buffer will be normalized according to the value ofdisableNormalization
. disableNormalization
, of type boolean, defaulting tofalse
-
The opposite of the desired initial value for the
normalize
attribute of theConvolverNode
.
1.17.4. Channel Configurations for Input, Impulse Response and Output
Implementations MUST support the following allowable configurations
of impulse response channels in a ConvolverNode
to achieve various reverb effects with 1 or 2 channels of input.
As shown in the diagram below, single channel convolution operates on a mono audio input, using a
mono impulse response, and generating a mono output. The remaining
images in the diagram illustrate the supported cases for mono and
stereo playback where the number of channels of the input is 1 or 2, and the number of channels in the buffer
is 1, 2, or 4.
Developers desiring more complex and arbitrary matrixing can use a ChannelSplitterNode
, multiple single-channel ConvolverNode
s and a ChannelMergerNode
.
If this node is not actively processing, the output is a single channel of silence.
Note: The diagrams below show the outputs when actively processing.
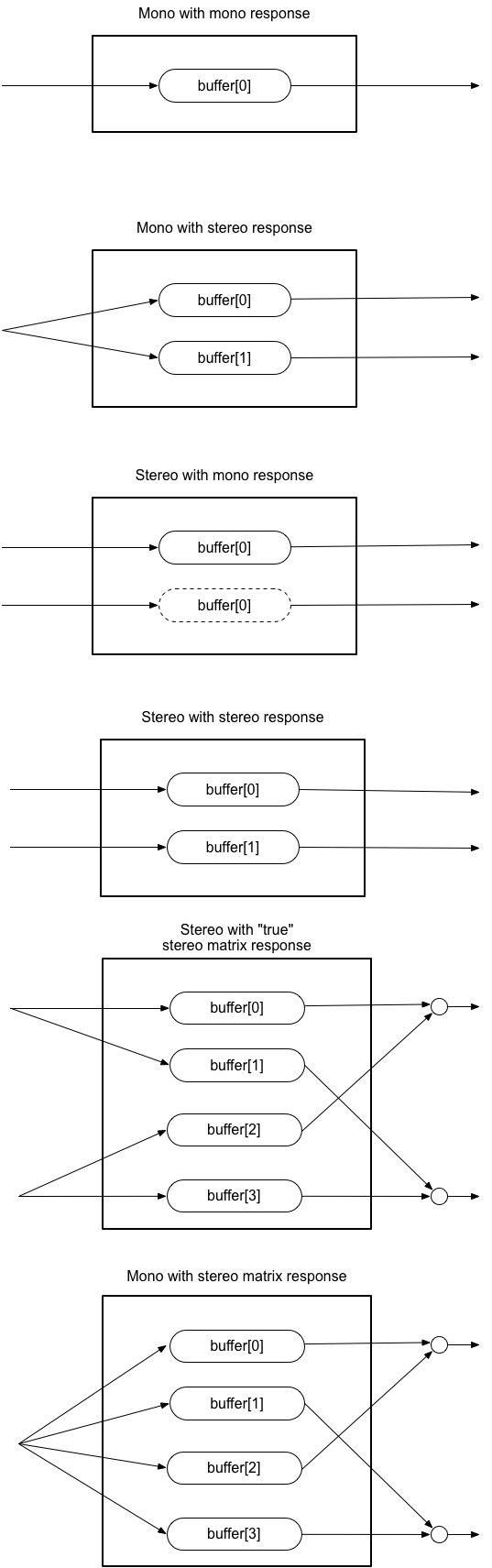
ConvolverNode
. 1.18. The DelayNode
Interface
A delay-line is a fundamental building block in audio applications.
This interface is an AudioNode
with a single
input and single output.
Property | Value | Notes |
---|---|---|
numberOfInputs
| 1 | |
numberOfOutputs
| 1 | |
channelCount
| 2 | |
channelCountMode
| "max "
| |
channelInterpretation
| "speakers "
| |
tail-time | Yes | Continues to output non-silent audio with zero input up to the maxDelayTime of the node.
|
The number of channels of the output always equals the number of channels of the input.
It delays the incoming audio signal by a certain amount.
Specifically, at each time t, input signal input(t), delay time delayTime(t) and output signal output(t), the output will be output(t) = input(t -
delayTime(t)). The default delayTime
is 0 seconds
(no delay).
When the number of channels in a DelayNode
's input changes
(thus changing the output channel count also), there may be delayed
audio samples which have not yet been output by the node and are part
of its internal state. If these samples were received earlier with a
different channel count, they MUST be upmixed or downmixed before
being combined with newly received input so that all internal
delay-line mixing takes place using the single prevailing channel
layout.
Note: By definition, a DelayNode
introduces an audio processing
latency equal to the amount of the delay.
[Exposed =Window ]interface DelayNode :AudioNode {constructor (BaseAudioContext ,
context optional DelayOptions = {});
options readonly attribute AudioParam delayTime ; };
1.18.1. Constructors
DelayNode(context, options)
-
When the constructor is called with a
BaseAudioContext
c and an option object option, the user agent MUST initialize the AudioNode this, with context and options as arguments.Arguments for the DelayNode.constructor() method. Parameter Type Nullable Optional Description context
BaseAudioContext
✘ ✘ The BaseAudioContext
this newDelayNode
will be associated with.options
DelayOptions
✘ ✔ Optional initial parameter value for this DelayNode
.
1.18.2. Attributes
delayTime
, of type AudioParam, readonly-
An
AudioParam
object representing the amount of delay (in seconds) to apply. Its defaultvalue
is 0 (no delay). The minimum value is 0 and the maximum value is determined by themaxDelayTime
argument to theAudioContext
methodcreateDelay()
or themaxDelayTime
member of theDelayOptions
dictionary for theconstructor
.If
DelayNode
is part of a cycle, then the value of thedelayTime
attribute is clamped to a minimum of one render quantum.Parameter Value Notes defaultValue
0 minValue
0 maxValue
maxDelayTime
automationRate
" a-rate
"
1.18.3. DelayOptions
This specifies options for constructing a DelayNode
. All members are optional; if not
given, the node is constructed using the normal defaults.
dictionary DelayOptions :AudioNodeOptions {double maxDelayTime = 1;double delayTime = 0; };
1.18.3.1. Dictionary DelayOptions
Members
delayTime
, of type double, defaulting to0
-
The initial delay time for the node.
maxDelayTime
, of type double, defaulting to1
-
The maximum delay time for the node. See
createDelay(maxDelayTime)
for constraints.
1.18.4. Processing
A DelayNode
has an internal buffer that holds delayTime
seconds of audio.
The processing of a DelayNode
is broken down in two parts: writing to the
delay line, and reading from the delay line. This is done via two internal AudioNode
s (that are not available to authors and exist only to ease
the description of the inner workings of the node). Both are created from a DelayNode
.
Creating a DelayWriter for a DelayNode
means creating an object
that has the same interface as an AudioNode
, and that writes the input audio
into the internal buffer of the DelayNode
. It has the same input connections
as the DelayNode
it was created from.
Creating a DelayReader for a DelayNode
means creating an object
that has the same interface as an AudioNode
, and that can read the audio
data from the internal buffer of the DelayNode
. It is connected to the same AudioNode
s as the DelayNode
it was created from. A DelayReader is
a source node.
When processing an input buffer, a DelayWriter MUST write the audio to
the internal buffer of the DelayNode
.
When producing an output buffer, a DelayReader MUST yield exactly the
audio that was written to the corresponding DelayWriter delayTime
seconds ago.
Note: This means that channel count changes are reflected after the delay time has passed.
1.19. The DynamicsCompressorNode
Interface
DynamicsCompressorNode
is an AudioNode
processor implementing a dynamics
compression effect.
Dynamics compression is very commonly used in musical production and game audio. It lowers the volume of the loudest parts of the signal and raises the volume of the softest parts. Overall, a louder, richer, and fuller sound can be achieved. It is especially important in games and musical applications where large numbers of individual sounds are played simultaneous to control the overall signal level and help avoid clipping (distorting) the audio output to the speakers.
Property | Value | Notes |
---|---|---|
numberOfInputs
| 1 | |
numberOfOutputs
| 1 | |
channelCount
| 2 | Has channelCount constraints |
channelCountMode
| "clamped-max "
| Has channelCountMode constraints |
channelInterpretation
| "speakers "
| |
tail-time | Yes | This node has a tail-time such that this node continues to output non-silent audio with zero input due to the look-ahead delay. |
[Exposed =Window ]interface DynamicsCompressorNode :AudioNode {constructor (BaseAudioContext ,
context optional DynamicsCompressorOptions = {});
options readonly attribute AudioParam threshold ;readonly attribute AudioParam knee ;readonly attribute AudioParam ratio ;readonly attribute float reduction ;readonly attribute AudioParam attack ;readonly attribute AudioParam release ; };
1.19.1. Constructors
DynamicsCompressorNode(context, options)
-
When the constructor is called with a
BaseAudioContext
c and an option object option, the user agent MUST initialize the AudioNode this, with context and options as arguments.Let
[[internal reduction]]
be a private slot on this, that holds a floating point number, in decibels. Set[[internal reduction]]
to 0.0.Arguments for the DynamicsCompressorNode.constructor() method. Parameter Type Nullable Optional Description context
BaseAudioContext
✘ ✘ The BaseAudioContext
this newDynamicsCompressorNode
will be associated with.options
DynamicsCompressorOptions
✘ ✔ Optional initial parameter value for this DynamicsCompressorNode
.
1.19.2. Attributes
attack
, of type AudioParam, readonly-
The amount of time (in seconds) to reduce the gain by 10dB.
Parameter Value Notes defaultValue
.003 minValue
0 maxValue
1 automationRate
" k-rate
"Has automation rate constraints knee
, of type AudioParam, readonly-
A decibel value representing the range above the threshold where the curve smoothly transitions to the "ratio" portion.
Parameter Value Notes defaultValue
30 minValue
0 maxValue
40 automationRate
" k-rate
"Has automation rate constraints ratio
, of type AudioParam, readonly-
The amount of dB change in input for a 1 dB change in output.
Parameter Value Notes defaultValue
12 minValue
1 maxValue
20 automationRate
" k-rate
"Has automation rate constraints reduction
, of type float, readonly-
A read-only decibel value for metering purposes, representing the current amount of gain reduction that the compressor is applying to the signal. If fed no signal the value will be 0 (no gain reduction). When this attribute is read, return the value of the private slot
[[internal reduction]]
. release
, of type AudioParam, readonly-
The amount of time (in seconds) to increase the gain by 10dB.
Parameter Value Notes defaultValue
.25 minValue
0 maxValue
1 automationRate
" k-rate
"Has automation rate constraints threshold
, of type AudioParam, readonly-
The decibel value above which the compression will start taking effect.
Parameter Value Notes defaultValue
-24 minValue
-100 maxValue
0 automationRate
" k-rate
"Has automation rate constraints
1.19.3. DynamicsCompressorOptions
This specifies the options to use in constructing a DynamicsCompressorNode
. All members are
optional; if not specified the normal defaults are used in
constructing the node.
dictionary DynamicsCompressorOptions :AudioNodeOptions {float attack = 0.003;float knee = 30;float ratio = 12;float release = 0.25;float threshold = -24; };
1.19.3.1. Dictionary DynamicsCompressorOptions
Members
attack
, of type float, defaulting to0.003
-
The initial value for the
attack
AudioParam. knee
, of type float, defaulting to30
-
The initial value for the
knee
AudioParam. ratio
, of type float, defaulting to12
-
The initial value for the
ratio
AudioParam. release
, of type float, defaulting to0.25
-
The initial value for the
release
AudioParam. threshold
, of type float, defaulting to-24
-
The initial value for the
threshold
AudioParam.
1.19.4. Processing
Dynamics compression can be implemented in a variety of ways. The DynamicsCompressorNode
implements a dynamics processor that
has the following characteristics:
-
Fixed look-ahead (this means that an
DynamicsCompressorNode
adds a fixed latency to the signal chain). -
Configurable attack speed, release speed, threshold, knee hardness and ratio.
-
Side-chaining is not supported.
-
The gain reduction is reported via the
reduction
property on theDynamicsCompressorNode
. -
The compression curve has three parts:
-
The first part is the identity: \(f(x) = x\).
-
The second part is the soft-knee portion, which MUST be a monotonically increasing function.
-
The third part is a linear function: \(f(x) = \frac{1}{ratio} \cdot x \).
This curve MUST be continuous and piece-wise differentiable, and corresponds to a target output level, based on the input level.
-
Graphically, such a curve would look something like this:
Internally, the DynamicsCompressorNode
is described with a
combination of other AudioNode
s, as well as a special
algorithm, to compute the gain reduction value.
The following AudioNode
graph is used internally, input
and output
respectively being the
input and output AudioNode
, context
the BaseAudioContext
for this DynamicsCompressorNode
, and
a new class, EnvelopeFollower, that instantiates a
special object that behaves like an AudioNode
, described
below:
const delay = new DelayNode(context, {delayTime: 0.006}); const gain = new GainNode(context); const compression = new EnvelopeFollower(); input.connect(delay).connect(gain).connect(output); input.connect(compression).connect(gain.gain);
AudioNode
s used as part of the DynamicsCompressorNode
processing algorithm. Note: This implements the pre-delay and the application of the reduction gain.
The following algorithm describes the processing performed by an EnvelopeFollower object, to be applied to the input signal to produce the gain reduction value. An EnvelopeFollower has two slots holding floating point values. Those values persist accros invocation of this algorithm.
-
Let
[[detector average]]
be a floating point number, initialized to 0.0. -
Let
[[compressor gain]]
be a floating point number, initialized to 1.0.
-
Let attack and release have the values of
attack
andrelease
, respectively, sampled at the time of processing (those are k-rate parameters), mutiplied by the sample-rate of theBaseAudioContext
thisDynamicsCompressorNode
is associated with. -
Let detector average be the value of the slot
[[detector average]]
. -
Let compressor gain be the value of the slot
[[compressor gain]]
. -
For each sample input of the render quantum to be processed, execute the following steps:
-
If the absolute value of input is less than 0.0001, let attenuation be 1.0. Else, let shaped input be the value of applying the compression curve to the absolute value of input. Let attenuation be shaped input divided by the absolute value of input.
-
Let releasing be
true
if attenuation is greater than compressor gain,false
otherwise. -
Let detector rate be the result of applying the detector curve to attenuation.
-
Subtract detector average from attenuation, and multiply the result by detector rate. Add this new result to detector average.
-
Clamp detector average to a maximum of 1.0.
-
Let envelope rate be the result of computing the envelope rate based on values of attack and release.
-
If releasing is
true
, set compressor gain to be the product of compressor gain and envelope rate, clamped to a maximum of 1.0. -
Else, if releasing is
false
, let gain increment to be detector average minus compressor gain. Multiply gain increment by envelope rate, and add the result to compressor gain. -
Compute reduction gain to be compressor gain multiplied by the return value of computing the makeup gain.
-
Compute metering gain to be reduction gain, converted to decibel.
-
-
Set
[[compressor gain]]
to compressor gain. -
Set
[[detector average]]
to detector average. -
Atomically set the internal slot
[[internal reduction]]
to the value of metering gain.Note: This step makes the metering gain update once per block, at the end of the block processing.
The makeup gain is a fixed gain stage that only depends on ratio, knee and threshold parameter of the compressor, and not on the input signal. The intent here is to increase the output level of the compressor so it is comparable to the input level.
-
Let full range gain be the value returned by applying the compression curve to the value 1.0.
-
Let full range makeup gain be the inverse of full range gain.
-
Return the result of taking the 0.6 power of full range makeup gain.
-
The envelope rate MUST be the calculated from the ratio of the compressor gain and the detector average.
Note: When attacking, this number less than or equal to 1, when releasing, this number is strictly greater than 1.
-
The attack curve MUST be a continuous, monotonically increasing function in the range \([0, 1]\). The shape of this curve MAY be controlled by
attack
. -
The release curve MUST be a continuous, monotonically decreasing function that is always greater than 1. The shape of this curve MAY be controlled by
release
.
This operation returns the value computed by applying this function to the ratio of compressor gain and detector average.
Applying the detector curve to the change rate when attacking or releasing allow implementing adaptive release. It is a function that MUST respect the following constraints:
-
The output of the function MUST be in \([0,1]\).
-
The function MUST be monotonically increasing, continuous.
Note: It is allowed, for example, to have a compressor that performs an adaptive release, that is, releasing faster the harder the compression, or to have curves for attack and release that are not of the same shape.
-
Let threshold and knee have the values of
threshold
andknee
, respectively, converted to linear units and sampled at the time of processing of this block (as k-rate parameters). -
Calculate the sum of
threshold
plusknee
also sampled at the time of processing of this block (as k-rate parameters). -
Let knee end threshold have the value of this sum converted to linear units.
-
Let ratio have the value of the
ratio
, sampled at the time of processing of this block (as a k-rate parameter). -
This function is the identity up to the value of the linear threshold (i.e., \(f(x) = x\)).
-
From the threshold up to the knee end threshold, User-Agents can choose the curve shape. The whole function MUST be monotonically increasing and continuous.
Note: If the knee is 0, the
DynamicsCompressorNode
is called a hard-knee compressor. -
This function is linear, based on the ratio, after the threshold and the soft knee (i.e., \(f(x) = \frac{1}{ratio} \cdot x \)).
-
If \(v\) is equal to zero, return -1000.
-
Else, return \( 20 \, \log_{10}{v} \).
1.20. The GainNode
Interface
Changing the gain of an audio signal is a fundamental operation in
audio applications. This
interface is an AudioNode
with a single input and
single output:
Property | Value | Notes |
---|---|---|
numberOfInputs
| 1 | |
numberOfOutputs
| 1 | |
channelCount
| 2 | |
channelCountMode
| "max "
| |
channelInterpretation
| "speakers "
| |
tail-time | No |
Each sample of each channel of the input data of the GainNode
MUST be multiplied by the computedValue of the gain
AudioParam
.
[Exposed =Window ]interface GainNode :AudioNode {constructor (BaseAudioContext ,
context optional GainOptions = {});
options readonly attribute AudioParam gain ; };
1.20.1. Constructors
GainNode(context, options)
-
When the constructor is called with a
BaseAudioContext
c and an option object option, the user agent MUST initialize the AudioNode this, with context and options as arguments.Arguments for the GainNode.constructor() method. Parameter Type Nullable Optional Description context
BaseAudioContext
✘ ✘ The BaseAudioContext
this newGainNode
will be associated with.options
GainOptions
✘ ✔ Optional initial parameter values for this GainNode
.
1.20.2. Attributes
gain
, of type AudioParam, readonly-
Represents the amount of gain to apply.
Parameter Value Notes defaultValue
1 minValue
most-negative-single-float Approximately -3.4028235e38 maxValue
most-positive-single-float Approximately 3.4028235e38 automationRate
" a-rate
"
1.20.3. GainOptions
This specifies options to use in constructing a GainNode
. All members are optional; if not
specified, the normal defaults are used in constructing the node.
dictionary GainOptions :AudioNodeOptions {float gain = 1.0; };
1.20.3.1. Dictionary GainOptions
Members
gain
, of type float, defaulting to1.0
-
The initial gain value for the
gain
AudioParam.
1.21. The IIRFilterNode
Interface
IIRFilterNode
is an AudioNode
processor implementing a general IIR Filter. In general, it is best
to use BiquadFilterNode
's to implement
higher-order filters for the following reasons:
-
Generally less sensitive to numeric issues
-
Filter parameters can be automated
-
Can be used to create all even-ordered IIR filters
However, odd-ordered filters cannot be created, so if such filters are needed or automation is not needed, then IIR filters may be appropriate.
Once created, the coefficients of the IIR filter cannot be changed.
Property | Value | Notes |
---|---|---|
numberOfInputs
| 1 | |
numberOfOutputs
| 1 | |
channelCount
| 2 | |
channelCountMode
| "max "
| |
channelInterpretation
| "speakers "
| |
tail-time | Yes | Continues to output non-silent audio with zero input. Since this is an IIR filter, the filter produces non-zero input forever, but in practice, this can be limited after some finite time where the output is sufficiently close to zero. The actual time depends on the filter coefficients. |
The number of channels of the output always equals the number of channels of the input.
[Exposed =Window ]interface IIRFilterNode :AudioNode {constructor (BaseAudioContext ,
context IIRFilterOptions );
options undefined getFrequencyResponse (Float32Array ,
frequencyHz Float32Array ,
magResponse Float32Array ); };
phaseResponse
1.21.1. Constructors
IIRFilterNode(context, options)
-
When the constructor is called with a
BaseAudioContext
c and an option object option, the user agent MUST initialize the AudioNode this, with context and options as arguments.Arguments for the IIRFilterNode.constructor() method. Parameter Type Nullable Optional Description context
BaseAudioContext
✘ ✘ The BaseAudioContext
this newIIRFilterNode
will be associated with.options
IIRFilterOptions
✘ ✘ Initial parameter value for this IIRFilterNode
.
1.21.2. Methods
getFrequencyResponse(frequencyHz, magResponse, phaseResponse)
-
Given the current filter parameter settings, synchronously calculates the frequency response for the specified frequencies. The three parameters MUST be
Float32Array
s of the same length, or anInvalidAccessError
MUST be thrown.Arguments for the IIRFilterNode.getFrequencyResponse() method. Parameter Type Nullable Optional Description frequencyHz
Float32Array
✘ ✘ This parameter specifies an array of frequencies, in Hz, at which the response values will be calculated. magResponse
Float32Array
✘ ✘ This parameter specifies an output array receiving the linear magnitude response values. If a value in the frequencyHz
parameter is not within [0, sampleRate/2], wheresampleRate
is the value of thesampleRate
property of theAudioContext
, the corresponding value at the same index of themagResponse
array MUST beNaN
.phaseResponse
Float32Array
✘ ✘ This parameter specifies an output array receiving the phase response values in radians. If a value in the frequencyHz
parameter is not within [0; sampleRate/2], wheresampleRate
is the value of thesampleRate
property of theAudioContext
, the corresponding value at the same index of thephaseResponse
array MUST beNaN
.Return type:undefined
1.21.3. IIRFilterOptions
The IIRFilterOptions
dictionary is used to specify the
filter coefficients of the IIRFilterNode
.
dictionary IIRFilterOptions :AudioNodeOptions {required sequence <double >feedforward ;required sequence <double >feedback ; };
1.21.3.1. Dictionary IIRFilterOptions
Members
feedforward
, of type sequence<double>-
The feedforward coefficients for the
IIRFilterNode
. This member is required. Seefeedforward
argument ofcreateIIRFilter()
for other constraints. feedback
, of type sequence<double>-
The feedback coefficients for the
IIRFilterNode
. This member is required. Seefeedback
argument ofcreateIIRFilter()
for other constraints.
1.21.4. Filter Definition
Let \(b_m\) be the feedforward
coefficients and
\(a_n\) be the feedback
coefficients specified by createIIRFilter()
or the IIRFilterOptions
dictionary for the constructor
. Then the
transfer function of the general IIR filter is given by
$$ H(z) = \frac{\sum_{m=0}^{M} b_m z^{-m}}{\sum_{n=0}^{N} a_n z^{-n}} $$
where \(M + 1\) is the length of the \(b\) array and \(N + 1\) is
the length of the \(a\) array. The coefficient \(a_0\) MUST not be 0 (see feedback parameter
for createIIRFilter()
).
At least one of \(b_m\) MUST be non-zero (see feedforward parameter
for createIIRFilter()
).
Equivalently, the time-domain equation is:
$$ \sum_{k=0}^{N} a_k y(n-k) = \sum_{k=0}^{M} b_k x(n-k) $$
The initial filter state is the all-zeroes state.
Note: The UA may produce a warning to notify the user that NaN values have occurred in the filter state. This is usually indicative of an unstable filter.
1.22. The MediaElementAudioSourceNode
Interface
This interface represents an audio source from an audio
or video
element.
Property | Value | Notes |
---|---|---|
numberOfInputs
| 0 | |
numberOfOutputs
| 1 | |
tail-time reference | No |
The number of channels of the output corresponds to the number of
channels of the media referenced by the HTMLMediaElement
. Thus, changes to the media element’s src
attribute can change the number of channels output
by this node.
If the sample rate of the HTMLMediaElement
differs from the sample
rate of the associated AudioContext
, then the output from the HTMLMediaElement
must be resampled to match the context’s sample rate
.
A MediaElementAudioSourceNode
is created given an HTMLMediaElement
using the AudioContext
createMediaElementSource()
method or the mediaElement
member of the MediaElementAudioSourceOptions
dictionary for the constructor
.
The number of channels of the single output equals the number of
channels of the audio referenced by the HTMLMediaElement
passed in as the argument to createMediaElementSource()
,
or is 1 if the HTMLMediaElement
has no audio.
The HTMLMediaElement
MUST behave in an identical fashion
after the MediaElementAudioSourceNode
has been created, except that the rendered audio will no longer be heard
directly, but instead will be heard as a consequence of the MediaElementAudioSourceNode
being connected through the
routing graph. Thus pausing, seeking, volume, src
attribute changes, and other aspects of the HTMLMediaElement
MUST behave as they normally would if not used with a MediaElementAudioSourceNode
.
const mediaElement= document. getElementById( 'mediaElementID' ); const sourceNode= context. createMediaElementSource( mediaElement); sourceNode. connect( filterNode);
[Exposed =Window ]interface MediaElementAudioSourceNode :AudioNode {constructor (AudioContext ,
context MediaElementAudioSourceOptions ); [
options SameObject ]readonly attribute HTMLMediaElement mediaElement ; };
1.22.1. Constructors
MediaElementAudioSourceNode(context, options)
-
-
initialize the AudioNode this, with context and options as arguments.
Arguments for the MediaElementAudioSourceNode.constructor() method. Parameter Type Nullable Optional Description context
AudioContext
✘ ✘ The AudioContext
this newMediaElementAudioSourceNode
will be associated with.options
MediaElementAudioSourceOptions
✘ ✘ Initial parameter value for this MediaElementAudioSourceNode
. -
1.22.2. Attributes
mediaElement
, of type HTMLMediaElement, readonly-
The
HTMLMediaElement
used when constructing thisMediaElementAudioSourceNode
.
1.22.3. MediaElementAudioSourceOptions
This specifies the options to use in constructing a MediaElementAudioSourceNode
.
dictionary MediaElementAudioSourceOptions {required HTMLMediaElement mediaElement ; };
1.22.3.1. Dictionary MediaElementAudioSourceOptions
Members
mediaElement
, of type HTMLMediaElement-
The media element that will be re-routed. This MUST be specified.
1.22.4. Security with MediaElementAudioSourceNode and Cross-Origin Resources
HTMLMediaElement
allows the playback of cross-origin
resources. Because Web Audio allows inspection of the content of
the resource (e.g. using a MediaElementAudioSourceNode
, and
an AudioWorkletNode
or ScriptProcessorNode
to read the samples), information
leakage can occur if scripts from one origin inspect the content of a resource from another origin.
To prevent this, a MediaElementAudioSourceNode
MUST output silence instead of the normal output of the HTMLMediaElement
if it has been created using an HTMLMediaElement
for which the execution of the fetch
algorithm [FETCH] labeled the resource as CORS-cross-origin.
1.23. The MediaStreamAudioDestinationNode
Interface
This interface is an audio destination representing a MediaStream
with a single MediaStreamTrack
whose kind
is "audio"
. This MediaStream
is
created when the node is created and is accessible via the stream
attribute. This stream can be used in a similar way
as a MediaStream
obtained via getUserMedia()
, and can, for example, be sent to a
remote peer using the RTCPeerConnection
(described in [webrtc]) addStream()
method.
Property | Value | Notes |
---|---|---|
numberOfInputs
| 1 | |
numberOfOutputs
| 0 | |
channelCount
| 2 | |
channelCountMode
| "explicit "
| |
channelInterpretation
| "speakers "
| |
tail-time | No |
The number of channels of the input is by default 2 (stereo).
[Exposed =Window ]interface MediaStreamAudioDestinationNode :AudioNode {constructor (AudioContext ,
context optional AudioNodeOptions = {});
options readonly attribute MediaStream stream ; };
1.23.1. Constructors
MediaStreamAudioDestinationNode(context, options)
-
-
Initialize the AudioNode this, with context and options as arguments.
Arguments for the MediaStreamAudioDestinationNode.constructor() method. Parameter Type Nullable Optional Description context
AudioContext
✘ ✘ The BaseAudioContext
this newMediaStreamAudioDestinationNode
will be associated with.options
AudioNodeOptions
✘ ✔ Optional initial parameter value for this MediaStreamAudioDestinationNode
. -
1.23.2. Attributes
stream
, of type MediaStream, readonly-
A
MediaStream
containing a singleMediaStreamTrack
with the same number of channels as the node itself, and whosekind
attribute has the value"audio"
.
1.24. The MediaStreamAudioSourceNode
Interface
This interface represents an audio source from a MediaStream
.
Property | Value | Notes |
---|---|---|
numberOfInputs
| 0 | |
numberOfOutputs
| 1 | |
tail-time reference | No |
The number of channels of the output corresponds to the number of channels of
the MediaStreamTrack
. When the MediaStreamTrack
ends, this AudioNode
outputs one channel of silence.
If the sample rate of the MediaStreamTrack
differs from the sample
rate of the associated AudioContext
, then the output of the MediaStreamTrack
is resampled to match the context’s sample rate
.
[Exposed =Window ]interface MediaStreamAudioSourceNode :AudioNode {constructor (AudioContext ,
context MediaStreamAudioSourceOptions ); [
options SameObject ]readonly attribute MediaStream mediaStream ; };
1.24.1. Constructors
MediaStreamAudioSourceNode(context, options)
-
-
If the
mediaStream
member ofoptions
does not reference aMediaStream
that has at least oneMediaStreamTrack
whosekind
attribute has the value"audio"
, throw anInvalidStateError
and abort these steps. Else, let this stream be inputStream. -
Let tracks be the list of all
MediaStreamTrack
s of inputStream that have akind
of"audio"
. -
Sort the elements in tracks based on their
id
attribute using an ordering on sequences of code unit values. -
Initialize the AudioNode this, with context and options as arguments.
-
Set an internal slot
[[input track]]
on thisMediaStreamAudioSourceNode
to be the first element of tracks. This is the track used as the input audio for thisMediaStreamAudioSourceNode
.
After construction, any change to the
MediaStream
that was passed to the constructor do not affect the underlying output of thisAudioNode
.The slot
[[input track]]
is only used to keep a reference to theMediaStreamTrack
.Note: This means that when removing the track chosen by the constructor of the
MediaStreamAudioSourceNode
from theMediaStream
passed into this constructor, theMediaStreamAudioSourceNode
will still take its input from the same track.Note: The behaviour for picking the track to output is arbitrary for legacy reasons.
MediaStreamTrackAudioSourceNode
can be used instead to be explicit about which track to use as input.Arguments for the MediaStreamAudioSourceNode.constructor() method. Parameter Type Nullable Optional Description context
AudioContext
✘ ✘ The AudioContext
this newMediaStreamAudioSourceNode
will be associated with.options
MediaStreamAudioSourceOptions
✘ ✘ Initial parameter value for this MediaStreamAudioSourceNode
. -
1.24.2. Attributes
mediaStream
, of type MediaStream, readonly-
The
MediaStream
used when constructing thisMediaStreamAudioSourceNode
.
1.24.3. MediaStreamAudioSourceOptions
This specifies the options for constructing a MediaStreamAudioSourceNode
.
dictionary MediaStreamAudioSourceOptions {required MediaStream mediaStream ; };
1.24.3.1. Dictionary MediaStreamAudioSourceOptions
Members
mediaStream
, of type MediaStream-
The media stream that will act as a source. This MUST be specified.
1.25. The MediaStreamTrackAudioSourceNode
Interface
This interface represents an audio source from a MediaStreamTrack
.
Property | Value | Notes |
---|---|---|
numberOfInputs
| 0 | |
numberOfOutputs
| 1 | |
tail-time reference | No |
The number of channels of the output corresponds to the number of
channels of the mediaStreamTrack
.
If the sample rate of the MediaStreamTrack
differs from the sample
rate of the associated AudioContext
, then the output of the mediaStreamTrack
is resampled
to match the context’s sample rate
.
[Exposed =Window ]interface MediaStreamTrackAudioSourceNode :AudioNode {constructor (AudioContext ,
context MediaStreamTrackAudioSourceOptions ); };
options
1.25.1. Constructors
MediaStreamTrackAudioSourceNode(context, options)
-
-
If the
mediaStreamTrack
'skind
attribute is not"audio"
, throw anInvalidStateError
and abort these steps. -
Initialize the AudioNode this, with context and options as arguments.
Arguments for the MediaStreamTrackAudioSourceNode.constructor() method. Parameter Type Nullable Optional Description context
AudioContext
✘ ✘ The AudioContext
this newMediaStreamTrackAudioSourceNode
will be associated with.options
MediaStreamTrackAudioSourceOptions
✘ ✘ Initial parameter value for this MediaStreamTrackAudioSourceNode
. -
1.25.2. MediaStreamTrackAudioSourceOptions
This specifies the options for constructing a MediaStreamTrackAudioSourceNode
. This is
required.
dictionary MediaStreamTrackAudioSourceOptions {required MediaStreamTrack mediaStreamTrack ; };
1.25.2.1. Dictionary MediaStreamTrackAudioSourceOptions
Members
mediaStreamTrack
, of type MediaStreamTrack-
The media stream track that will act as a source. If this
MediaStreamTrack
kind
attribute is not"audio"
, anInvalidStateError
MUST be thrown.
1.26. The OscillatorNode
Interface
OscillatorNode
represents an audio source
generating a periodic waveform. It can be set to a few commonly used
waveforms. Additionally, it can be set to an arbitrary periodic
waveform through the use of a PeriodicWave
object.
Oscillators are common foundational building blocks in audio
synthesis. An OscillatorNode will start emitting sound at the time
specified by the start()
method.
Mathematically speaking, a continuous-time periodic waveform can have very high (or infinitely high) frequency information when considered in the frequency domain. When this waveform is sampled as a discrete-time digital audio signal at a particular sample-rate, then care MUST be taken to discard (filter out) the high-frequency information higher than the Nyquist frequency before converting the waveform to a digital form. If this is not done, then aliasing of higher frequencies (than the Nyquist frequency) will fold back as mirror images into frequencies lower than the Nyquist frequency. In many cases this will cause audibly objectionable artifacts. This is a basic and well-understood principle of audio DSP.
There are several practical approaches that an implementation may take to avoid this aliasing. Regardless of approach, the idealized discrete-time digital audio signal is well defined mathematically. The trade-off for the implementation is a matter of implementation cost (in terms of CPU usage) versus fidelity to achieving this ideal.
It is expected that an implementation will take some care in achieving this ideal, but it is reasonable to consider lower-quality, less-costly approaches on lower-end hardware.
Both frequency
and detune
are a-rate parameters, and form a compound parameter. They are used
together to determine a computedOscFrequency value:
computedOscFrequency(t) = frequency(t) * pow(2, detune(t) / 1200)
The OscillatorNode’s instantaneous phase at each time is the definite time integral of computedOscFrequency, assuming a phase angle of zero at the node’s exact start time. Its nominal range is [-Nyquist frequency, Nyquist frequency].
The single output of this node consists of one channel (mono).
Property | Value | Notes |
---|---|---|
numberOfInputs
| 0 | |
numberOfOutputs
| 1 | |
channelCount
| 2 | |
channelCountMode
| "max "
| |
channelInterpretation
| "speakers "
| |
tail-time | No |
enum {
OscillatorType "sine" ,"square" ,"sawtooth" ,"triangle" ,"custom" };
Enum value | Description |
---|---|
"sine "
| A sine wave |
"square "
| A square wave of duty period 0.5 |
"sawtooth "
| A sawtooth wave |
"triangle "
| A triangle wave |
"custom "
| A custom periodic wave |
[Exposed =Window ]interface OscillatorNode :AudioScheduledSourceNode {constructor (BaseAudioContext ,
context optional OscillatorOptions = {});
options attribute OscillatorType type ;readonly attribute AudioParam frequency ;readonly attribute AudioParam detune ;undefined setPeriodicWave (PeriodicWave ); };
periodicWave
1.26.1. Constructors
OscillatorNode(context, options)
-
When the constructor is called with a
BaseAudioContext
c and an option object option, the user agent MUST initialize the AudioNode this, with context and options as arguments.Arguments for the OscillatorNode.constructor() method. Parameter Type Nullable Optional Description context
BaseAudioContext
✘ ✘ The BaseAudioContext
this newOscillatorNode
will be associated with.options
OscillatorOptions
✘ ✔ Optional initial parameter value for this OscillatorNode
.
1.26.2. Attributes
detune
, of type AudioParam, readonly-
A detuning value (in cents) which will offset the
frequency
by the given amount. Its defaultvalue
is 0. This parameter is a-rate. It forms a compound parameter withfrequency
to form the computedOscFrequency. The nominal range listed below allows this parameter to detune thefrequency
over the entire possible range of frequencies.Parameter Value Notes defaultValue
0 minValue
\(\approx -153600\) maxValue
\(\approx 153600\) This value is approximately \(1200\ \log_2 \mathrm{FLT\_MAX}\) where FLT_MAX is the largest float
value.automationRate
" a-rate
" frequency
, of type AudioParam, readonly-
The frequency (in Hertz) of the periodic waveform. Its default
value
is 440. This parameter is a-rate. It forms a compound parameter withdetune
to form the computedOscFrequency. Its nominal range is [-Nyquist frequency, Nyquist frequency].Parameter Value Notes defaultValue
440 minValue
-Nyquist frequency maxValue
Nyquist frequency automationRate
" a-rate
" type
, of type OscillatorType-
The shape of the periodic waveform. It may directly be set to any of the type constant values except for "
custom
". Doing so MUST throw anInvalidStateError
exception. ThesetPeriodicWave()
method can be used to set a custom waveform, which results in this attribute being set to "custom
". The default value is "sine
". When this attribute is set, the phase of the oscillator MUST be conserved.
1.26.3. Methods
setPeriodicWave(periodicWave)
-
Sets an arbitrary custom periodic waveform given a
PeriodicWave
.Arguments for the OscillatorNode.setPeriodicWave() method. Parameter Type Nullable Optional Description periodicWave
PeriodicWave
✘ ✘ custom waveform to be used by the oscillator Return type:undefined
1.26.4. OscillatorOptions
This specifies the options to be used when constructing an OscillatorNode
. All of the members are
optional; if not specified, the normal default values are used for
constructing the oscillator.
dictionary OscillatorOptions :AudioNodeOptions {OscillatorType type = "sine";float frequency = 440;float detune = 0;PeriodicWave periodicWave ; };
1.26.4.1. Dictionary OscillatorOptions
Members
detune
, of type float, defaulting to0
-
The initial detune value for the
OscillatorNode
. frequency
, of type float, defaulting to440
-
The initial frequency for the
OscillatorNode
. periodicWave
, of type PeriodicWave-
The
PeriodicWave
for theOscillatorNode
. If this is specified, then any valid value fortype
is ignored; it is treated as if "custom
" were specified. type
, of type OscillatorType, defaulting to"sine"
-
The type of oscillator to be constructed. If this is set to "custom" without also specifying a
periodicWave
, then anInvalidStateError
exception MUST be thrown. IfperiodicWave
is specified, then any valid value fortype
is ignored; it is treated as if it were set to "custom".
1.26.5. Basic Waveform Phase
The idealized mathematical waveforms for the various oscillator types are defined below. In summary, all waveforms are defined mathematically to be an odd function with a positive slope at time 0. The actual waveforms produced by the oscillator may differ to prevent aliasing affects.
The oscillator MUST produce the same result as if a PeriodicWave
,
with the appropriate Fourier
series and with disableNormalization
set to false, were used to create these
basic waveforms.
- "
sine
" -
The waveform for sine oscillator is:
$$ x(t) = \sin t $$
- "
square
" -
The waveform for the square wave oscillator is:
$$ x(t) = \begin{cases} 1 & \mbox{for } 0≤ t < \pi \\ -1 & \mbox{for } -\pi < t < 0. \end{cases} $$
This is extended to all \(t\) by using the fact that the waveform is an odd function with period \(2\pi\).
- "
sawtooth
" -
The waveform for the sawtooth oscillator is the ramp:
$$ x(t) = \frac{t}{\pi} \mbox{ for } -\pi < t ≤ \pi; $$
This is extended to all \(t\) by using the fact that the waveform is an odd function with period \(2\pi\).
- "
triangle
" -
The waveform for the triangle oscillator is:
$$ x(t) = \begin{cases} \frac{2}{\pi} t & \mbox{for } 0 ≤ t ≤ \frac{\pi}{2} \\ 1-\frac{2}{\pi} \left(t-\frac{\pi}{2}\right) & \mbox{for } \frac{\pi}{2} < t ≤ \pi. \end{cases} $$
This is extended to all \(t\) by using the fact that the waveform is an odd function with period \(2\pi\).
1.27. The PannerNode
Interface
This interface represents a processing node which positions / spatializes an incoming audio
stream in three-dimensional space. The spatialization is in relation
to the BaseAudioContext
's AudioListener
(listener
attribute).
Property | Value | Notes |
---|---|---|
numberOfInputs
| 1 | |
numberOfOutputs
| 1 | |
channelCount
| 2 | Has channelCount constraints |
channelCountMode
| "clamped-max "
| Has channelCountMode constraints |
channelInterpretation
| "speakers "
| |
tail-time | Maybe | If the panningModel is set to "HRTF ", the node will produce non-silent output for silent input due to the inherent processing for head responses. Otherwise the tail-time is zero.
|
The input of this node is either mono (1 channel) or stereo (2 channels) and cannot be increased. Connections from nodes with fewer or more channels will be up-mixed or down-mixed appropriately.
If the node is actively processing, the output of this node is hard-coded to stereo (2 channels) and cannot be configured. If the node is not actively processing, then the output is a single channel of silence.
The PanningModelType
enum determines which
spatialization algorithm will be used to position the audio in 3D
space. The default is "equalpower
".
enum {
PanningModelType "equalpower" ,"HRTF" };
Enum value | Description |
---|---|
"equalpower "
|
A simple and efficient spatialization algorithm using equal-power
panning.
Note: When this panning model is used, all the |
"HRTF "
|
A higher quality spatialization algorithm using a convolution
with measured impulse responses from human subjects. This panning
method renders stereo output.
Note:When this panning model is used, all the |
The effective automation rate for an AudioParam
of a PannerNode
is determined by the panningModel
and automationRate
of the AudioParam
. If the panningModel
is "HRTF
", the effective automation rate is "k-rate
",
independent of the setting of the automationRate
.
Otherwise the effective automation rate is the value of automationRate
.
The DistanceModelType
enum determines which
algorithm will be used to reduce the volume of an audio source as it
moves away from the listener. The default is "inverse
".
In the description of each distance model below, let \(d\) be the
distance between the listener and the panner; \(d_{ref}\) be the
value of the refDistance
attribute; \(d_{max}\) be the
value of the maxDistance
attribute; and \(f\) be the
value of the rolloffFactor
attribute.
enum {
DistanceModelType "linear" ,"inverse" ,"exponential" };
Enum value | Description |
---|---|
"linear "
|
A linear distance model which calculates distanceGain according to:
$$ 1 - f\ \frac{\max\left[\min\left(d, d’_{max}\right), d’_{ref}\right] - d’_{ref}}{d’_{max} - d’_{ref}} $$ where \(d’_{ref} = \min\left(d_{ref}, d_{max}\right)\) and \(d’_{max} = \max\left(d_{ref}, d_{max}\right)\). In the case where \(d’_{ref} = d’_{max}\), the value of the linear model is taken to be \(1-f\). Note that \(d\) is clamped to the interval \(\left[d’_{ref},\, d’_{max}\right]\). |
"inverse "
|
An inverse distance model which calculates distanceGain according to:
$$ \frac{d_{ref}}{d_{ref} + f\ \left[\max\left(d, d_{ref}\right) - d_{ref}\right]} $$ That is, \(d\) is clamped to the interval \(\left[d_{ref},\, \infty\right)\). If \(d_{ref} = 0\), the value of the inverse model is taken to be 0, independent of the value of \(d\) and \(f\). |
"exponential "
|
An exponential distance model which calculates distanceGain according to:
$$ \left[\frac{\max\left(d, d_{ref}\right)}{d_{ref}}\right]^{-f} $$ That is, \(d\) is clamped to the interval \(\left[d_{ref},\, \infty\right)\). If \(d_{ref} = 0\), the value of the exponential model is taken to be 0, independent of \(d\) and \(f\). |
[Exposed =Window ]interface PannerNode :AudioNode {constructor (BaseAudioContext ,
context optional PannerOptions = {});
options attribute PanningModelType panningModel ;readonly attribute AudioParam positionX ;readonly attribute AudioParam positionY ;readonly attribute AudioParam positionZ ;readonly attribute AudioParam orientationX ;readonly attribute AudioParam orientationY ;readonly attribute AudioParam orientationZ ;attribute DistanceModelType distanceModel ;attribute double refDistance ;attribute double maxDistance ;attribute double rolloffFactor ;attribute double coneInnerAngle ;attribute double coneOuterAngle ;attribute double coneOuterGain ;undefined setPosition (float ,
x float ,
y float );
z undefined setOrientation (float ,
x float ,
y float ); };
z
1.27.1. Constructors
PannerNode(context, options)
-
When the constructor is called with a
BaseAudioContext
c and an option object option, the user agent MUST initialize the AudioNode this, with context and options as arguments.Arguments for the PannerNode.constructor() method. Parameter Type Nullable Optional Description context
BaseAudioContext
✘ ✘ The BaseAudioContext
this newPannerNode
will be associated with.options
PannerOptions
✘ ✔ Optional initial parameter value for this PannerNode
.
1.27.2. Attributes
coneInnerAngle
, of type double-
A parameter for directional audio sources that is an angle, in degrees, inside of which there will be no volume reduction. The default value is 360. The behavior is undefined if the angle is outside the interval [0, 360].
coneOuterAngle
, of type double-
A parameter for directional audio sources that is an angle, in degrees, outside of which the volume will be reduced to a constant value of
coneOuterGain
. The default value is 360. The behavior is undefined if the angle is outside the interval [0, 360]. coneOuterGain
, of type double-
A parameter for directional audio sources that is the gain outside of the
coneOuterAngle
. The default value is 0. It is a linear value (not dB) in the range [0, 1]. AnInvalidStateError
MUST be thrown if the parameter is outside this range. distanceModel
, of type DistanceModelType-
Specifies the distance model used by this
PannerNode
. Defaults to "inverse
". maxDistance
, of type double-
The maximum distance between source and listener, after which the volume will not be reduced any further. The default value is 10000. A
RangeError
exception MUST be thrown if this is set to a non-positive value. orientationX
, of type AudioParam, readonly-
Describes the \(x\)-component of the vector of the direction the audio source is pointing in 3D Cartesian coordinate space.
Parameter Value Notes defaultValue
1 minValue
most-negative-single-float Approximately -3.4028235e38 maxValue
most-positive-single-float Approximately 3.4028235e38 automationRate
" a-rate
"Has automation rate constraints orientationY
, of type AudioParam, readonly-
Describes the \(y\)-component of the vector of the direction the audio source is pointing in 3D cartesian coordinate space.
Parameter Value Notes defaultValue
0 minValue
most-negative-single-float Approximately -3.4028235e38 maxValue
most-positive-single-float Approximately 3.4028235e38 automationRate
" a-rate
"Has automation rate constraints orientationZ
, of type AudioParam, readonly-
Describes the \(z\)-component of the vector of the direction the audio source is pointing in 3D cartesian coordinate space.
Parameter Value Notes defaultValue
0 minValue
most-negative-single-float Approximately -3.4028235e38 maxValue
most-positive-single-float Approximately 3.4028235e38 automationRate
" a-rate
"Has automation rate constraints panningModel
, of type PanningModelType-
Specifies the panning model used by this
PannerNode
. Defaults to "equalpower
". positionX
, of type AudioParam, readonly-
Sets the \(x\)-coordinate position of the audio source in a 3D Cartesian system.
Parameter Value Notes defaultValue
0 minValue
most-negative-single-float Approximately -3.4028235e38 maxValue
most-positive-single-float Approximately 3.4028235e38 automationRate
" a-rate
"Has automation rate constraints positionY
, of type AudioParam, readonly-
Sets the \(y\)-coordinate position of the audio source in a 3D Cartesian system.
Parameter Value Notes defaultValue
0 minValue
most-negative-single-float Approximately -3.4028235e38 maxValue
most-positive-single-float Approximately 3.4028235e38 automationRate
" a-rate
"Has automation rate constraints positionZ
, of type AudioParam, readonly-
Sets the \(z\)-coordinate position of the audio source in a 3D Cartesian system.
Parameter Value Notes defaultValue
0 minValue
most-negative-single-float Approximately -3.4028235e38 maxValue
most-positive-single-float Approximately 3.4028235e38 automationRate
" a-rate
"Has automation rate constraints refDistance
, of type double-
A reference distance for reducing volume as source moves further from the listener. For distances less than this, the volume is not reduced. The default value is 1. A
RangeError
exception MUST be thrown if this is set to a negative value. rolloffFactor
, of type double-
Describes how quickly the volume is reduced as source moves away from listener. The default value is 1. A
RangeError
exception MUST be thrown if this is set to a negative value.The nominal range for the
rolloffFactor
specifies the minimum and maximum values therolloffFactor
can have. Values outside the range are clamped to lie within this range. The nominal range depends on thedistanceModel
as follows:- "
linear
" -
The nominal range is \([0, 1]\).
- "
inverse
" -
The nominal range is \([0, \infty)\).
- "
exponential
" -
The nominal range is \([0, \infty)\).
Note that the clamping happens as part of the processing of the distance computation. The attribute reflects the value that was set and is not modified.
- "
1.27.3. Methods
setOrientation(x, y, z)
-
This method is DEPRECATED. It is equivalent to setting
orientationX
.value
,orientationY
.value
, andorientationZ
.value
attribute directly, with thex
,y
andz
parameters, respectively.Consequently, if any of the
orientationX
,orientationY
, andorientationZ
AudioParam
s have an automation curve set usingsetValueCurveAtTime()
at the time this method is called, aNotSupportedError
MUST be thrown.Describes which direction the audio source is pointing in the 3D cartesian coordinate space. Depending on how directional the sound is (controlled by the cone attributes), a sound pointing away from the listener can be very quiet or completely silent.
The
x, y, z
parameters represent a direction vector in 3D space.The default value is (1,0,0).
Arguments for the PannerNode.setOrientation() method. Parameter Type Nullable Optional Description x
float
✘ ✘ y
float
✘ ✘ z
float
✘ ✘ Return type:undefined
setPosition(x, y, z)
-
This method is DEPRECATED. It is equivalent to setting
positionX
.value
,positionY
.value
, andpositionZ
.value
attribute directly with thex
,y
andz
parameters, respectively.Consequently, if any of the
positionX
,positionY
, andpositionZ
AudioParam
s have an automation curve set usingsetValueCurveAtTime()
at the time this method is called, aNotSupportedError
MUST be thrown.Sets the position of the audio source relative to the
listener
attribute. A 3D cartesian coordinate system is used.The
x, y, z
parameters represent the coordinates in 3D space.The default value is (0,0,0).
Arguments for the PannerNode.setPosition() method. Parameter Type Nullable Optional Description x
float
✘ ✘ y
float
✘ ✘ z
float
✘ ✘ Return type:undefined
1.27.4. PannerOptions
This specifies options for constructing a PannerNode
. All members are optional; if not
specified, the normal default is used in constructing the node.
dictionary PannerOptions :AudioNodeOptions {PanningModelType panningModel = "equalpower";DistanceModelType distanceModel = "inverse";float positionX = 0;float positionY = 0;float positionZ = 0;float orientationX = 1;float orientationY = 0;float orientationZ = 0;double refDistance = 1;double maxDistance = 10000;double rolloffFactor = 1;double coneInnerAngle = 360;double coneOuterAngle = 360;double coneOuterGain = 0; };
1.27.4.1. Dictionary PannerOptions
Members
coneInnerAngle
, of type double, defaulting to360
-
The initial value for the
coneInnerAngle
attribute of the node. coneOuterAngle
, of type double, defaulting to360
-
The initial value for the
coneOuterAngle
attribute of the node. coneOuterGain
, of type double, defaulting to0
-
The initial value for the
coneOuterGain
attribute of the node. distanceModel
, of type DistanceModelType, defaulting to"inverse"
-
The distance model to use for the node.
maxDistance
, of type double, defaulting to10000
-
The initial value for the
maxDistance
attribute of the node. orientationX
, of type float, defaulting to1
-
The initial \(x\)-component value for the
orientationX
AudioParam. orientationY
, of type float, defaulting to0
-
The initial \(y\)-component value for the
orientationY
AudioParam. orientationZ
, of type float, defaulting to0
-
The initial \(z\)-component value for the
orientationZ
AudioParam. panningModel
, of type PanningModelType, defaulting to"equalpower"
-
The panning model to use for the node.
positionX
, of type float, defaulting to0
-
The initial \(x\)-coordinate value for the
positionX
AudioParam. positionY
, of type float, defaulting to0
-
The initial \(y\)-coordinate value for the
positionY
AudioParam. positionZ
, of type float, defaulting to0
-
The initial \(z\)-coordinate value for the
positionZ
AudioParam. refDistance
, of type double, defaulting to1
-
The initial value for the
refDistance
attribute of the node. rolloffFactor
, of type double, defaulting to1
-
The initial value for the
rolloffFactor
attribute of the node.
1.27.5. Channel Limitations
The set of channel
limitations for StereoPannerNode
also apply
to PannerNode
.
1.28. The PeriodicWave
Interface
PeriodicWave
represents an arbitrary periodic waveform to be used
with an OscillatorNode
.
A conforming implementation MUST support PeriodicWave
up to at least 8192 elements.
[Exposed =Window ]interface PeriodicWave {constructor (BaseAudioContext ,
context optional PeriodicWaveOptions = {}); };
options
1.28.1. Constructors
PeriodicWave(context, options)
-
-
Let p be a new
PeriodicWave
object. Let[[real]]
and[[imag]]
be two internal slots of typeFloat32Array
, and let[[normalize]]
be an internal slot. -
Process
options
according to one of the following cases:-
If both
options.real
andoptions.imag
are present-
If the lengths of
options.real
andoptions.imag
are different or if either length is less than 2, throw anIndexSizeError
and abort this algorithm. -
Set
[[real]]
and[[imag]]
to new arrays with the same length asoptions.real
. -
Copy all elements from
options.real
to[[real]]
andoptions.imag
to[[imag]]
.
-
-
If only
options.real
is present-
If length of
options.real
is less than 2, throw anIndexSizeError
and abort this algorithm. -
Set
[[real]]
and[[imag]]
to arrays with the same length asoptions.real
. -
Copy
options.real
to[[real]]
and set[[imag]]
to all zeros.
-
-
If only
options.imag
is present-
If length of
options.imag
is less than 2, throw anIndexSizeError
and abort this algorithm. -
Set
[[real]]
and[[imag]]
to arrays with the same length asoptions.imag
. -
Copy
options.imag
to[[imag]]
and set[[real]]
to all zeros.
-
-
Otherwise
-
Set
[[real]]
and[[imag]]
to zero-filled arrays of length 2. -
Set element at index 1 of
[[imag]]
to 1.
Note: When setting this
PeriodicWave
on anOscillatorNode
, this is equivalent to using the built-in type "sine
". -
-
-
Set element at index 0 of both
[[real]]
and[[imag]]
to 0. (This sets the DC component to 0.) -
Initialize
[[normalize]]
to the inverse of thedisableNormalization
attribute of thePeriodicWaveConstraints
on thePeriodicWaveOptions
. -
Return p.
Arguments for the PeriodicWave.constructor() method. Parameter Type Nullable Optional Description context
BaseAudioContext
✘ ✘ The BaseAudioContext
this newPeriodicWave
will be associated with. UnlikeAudioBuffer
,PeriodicWave
s can’t be shared accrossAudioContext
s orOfflineAudioContext
s. It is associated with a particularBaseAudioContext
.options
PeriodicWaveOptions
✘ ✔ Optional initial parameter value for this PeriodicWave
. -
1.28.2. PeriodicWaveConstraints
The PeriodicWaveConstraints
dictionary is used to
specify how the waveform is normalized.
dictionary PeriodicWaveConstraints {boolean disableNormalization =false ; };
1.28.2.1. Dictionary PeriodicWaveConstraints
Members
disableNormalization
, of type boolean, defaulting tofalse
-
Controls whether the periodic wave is normalized or not. If
true
, the waveform is not normalized; otherwise, the waveform is normalized.
1.28.3. PeriodicWaveOptions
The PeriodicWaveOptions
dictionary is used to specify
how the waveform is constructed. If only one of real
or imag
is specified. The other is treated as if it
were an array of all zeroes of the same length, as specified below
in description of
the dictionary members. If neither is given, a PeriodicWave
is created that MUST be equivalent
to an OscillatorNode
with type
"sine
". If
both are given, the sequences must have the same length; otherwise
an error of type NotSupportedError
MUST be thrown.
dictionary PeriodicWaveOptions :PeriodicWaveConstraints {sequence <float >real ;sequence <float >imag ; };
1.28.3.1. Dictionary PeriodicWaveOptions
Members
imag
, of type sequence<float>-
The
imag
parameter represents an array ofsine
terms. The first element (index 0) does not exist in the Fourier series. The second element (index 1) represents the fundamental frequency. The third represents the first overtone and so on. real
, of type sequence<float>-
The
real
parameter represents an array ofcosine
terms. The first element (index 0) is the DC-offset of the periodic waveform. The second element (index 1) represents the fundmental frequency. The third represents the first overtone and so on.
1.28.4. Waveform Generation
The createPeriodicWave()
method takes two arrays to specify the Fourier coefficients of the PeriodicWave
. Let \(a\) and \(b\) represent the [[real]]
and [[imag]]
arrays of length \(L\), respectively. Then the basic time-domain waveform,
\(x(t)\), can be computed using:
$$ x(t) = \sum_{k=1}^{L-1} \left[a[k]\cos2\pi k t + b[k]\sin2\pi k t\right] $$
This is the basic (unnormalized) waveform.
1.28.5. Waveform Normalization
If the internal slot [[normalize]]
of this PeriodicWave
is true
(the default), the
waveform defined in the previous section is normalized so that the
maximum value is 1. The normalization is done as follows.
Let
$$ \tilde{x}(n) = \sum_{k=1}^{L-1} \left(a[k]\cos\frac{2\pi k n}{N} + b[k]\sin\frac{2\pi k n}{N}\right) $$
where \(N\) is a power of two. (Note: \(\tilde{x}(n)\) can conveniently be computed using an inverse FFT.) The fixed normalization factor \(f\) is computed as follows.
$$ f = \max_{n = 0, \ldots, N - 1} |\tilde{x}(n)| $$
Thus, the actual normalized waveform \(\hat{x}(n)\) is:
$$ \hat{x}(n) = \frac{\tilde{x}(n)}{f} $$
This fixed normalization factor MUST be applied to all generated waveforms.
1.28.6. Oscillator Coefficients
The builtin oscillator types are created using PeriodicWave
objects. For completeness the coefficients for the PeriodicWave
for
each of the builtin oscillator types is given here. This is useful
if a builtin type is desired but without the default normalization.
In the following descriptions, let \(a\) be the array of real
coefficients and \(b\) be the array of imaginary coefficients for createPeriodicWave()
. In
all cases \(a[n] = 0\) for all \(n\) because the waveforms are odd
functions. Also, \(b[0] = 0\) in all cases. Hence, only \(b[n]\)
for \(n \ge 1\) is specified below.
- "
sine
" -
$$ b[n] = \begin{cases} 1 & \mbox{for } n = 1 \\ 0 & \mbox{otherwise} \end{cases} $$
- "
square
" -
$$ b[n] = \frac{2}{n\pi}\left[1 - (-1)^n\right] $$
- "
sawtooth
" -
$$ b[n] = (-1)^{n+1} \dfrac{2}{n\pi} $$
- "
triangle
" -
$$ b[n] = \frac{8\sin\dfrac{n\pi}{2}}{(\pi n)^2} $$
1.29. The ScriptProcessorNode
Interface - DEPRECATED
This interface is an AudioNode
which can
generate, process, or analyse audio directly using a script. This
node type is deprecated, to be replaced by the AudioWorkletNode
; this text is only here for informative
purposes until implementations remove this node type.
Property | Value | Notes |
---|---|---|
numberOfInputs
| 1 | |
numberOfOutputs
| 1 | |
channelCount
| numberOfInputChannels
| This is the number of channels specified when constructing this node. There are channelCount constraints. |
channelCountMode
| "explicit "
| Has channelCountMode constraints |
channelInterpretation
| "speakers "
| |
tail-time | No |
The ScriptProcessorNode
is constructed with a bufferSize
which MUST be one of the following values: 256,
512, 1024, 2048, 4096, 8192, 16384. This value controls how
frequently the audioprocess
event is dispatched and how
many sample-frames need to be processed each call. audioprocess
events are only
dispatched if the ScriptProcessorNode
has at
least one input or one output connected. Lower numbers for bufferSize
will result in
a lower (better) latency. Higher numbers will
be necessary to avoid audio breakup and glitches. This value will be picked by the
implementation if the bufferSize argument to createScriptProcessor()
is not passed in, or is set to 0.
numberOfInputChannels
and numberOfOutputChannels
determine the number of input and output channels. It is invalid for
both numberOfInputChannels
and numberOfOutputChannels
to be zero.
[Exposed =Window ]interface ScriptProcessorNode :AudioNode {attribute EventHandler onaudioprocess ;readonly attribute long bufferSize ; };
1.29.1. Attributes
bufferSize
, of type long, readonly-
The size of the buffer (in sample-frames) which needs to be processed each time
audioprocess
is fired. Legal values are (256, 512, 1024, 2048, 4096, 8192, 16384). onaudioprocess
, of type EventHandler-
A property used to set an event handler for the
audioprocess
event type that is dispatched toScriptProcessorNode
node types. The event dispatched to the event handler uses theAudioProcessingEvent
interface.
1.30. The StereoPannerNode
Interface
This interface represents a processing node which positions an incoming audio stream in a stereo image using a low-cost panning algorithm. This panning effect is common in positioning audio components in a stereo stream.
Property | Value | Notes |
---|---|---|
numberOfInputs
| 1 | |
numberOfOutputs
| 1 | |
channelCount
| 2 | Has channelCount constraints |
channelCountMode
| "clamped-max "
| Has channelCountMode constraints |
channelInterpretation
| "speakers "
| |
tail-time | No |
The input of this node is stereo (2 channels) and cannot be increased. Connections from nodes with fewer or more channels will be up-mixed or down-mixed appropriately.
The output of this node is hard-coded to stereo (2 channels) and cannot be configured.
[Exposed =Window ]interface StereoPannerNode :AudioNode {constructor (BaseAudioContext ,
context optional StereoPannerOptions = {});
options readonly attribute AudioParam pan ; };
1.30.1. Constructors
StereoPannerNode(context, options)
-
When the constructor is called with a
BaseAudioContext
c and an option object option, the user agent MUST initialize the AudioNode this, with context and options as arguments.Arguments for the StereoPannerNode.constructor() method. Parameter Type Nullable Optional Description context
BaseAudioContext
✘ ✘ The BaseAudioContext
this newStereoPannerNode
will be associated with.options
StereoPannerOptions
✘ ✔ Optional initial parameter value for this StereoPannerNode
.
1.30.2. Attributes
pan
, of type AudioParam, readonly-
The position of the input in the output’s stereo image. -1 represents full left, +1 represents full right.
Parameter Value Notes defaultValue
0 minValue
-1 maxValue
1 automationRate
" a-rate
"
1.30.3. StereoPannerOptions
This specifies the options to use in constructing a StereoPannerNode
. All members are optional; if
not specified, the normal default is used in constructing the node.
dictionary StereoPannerOptions :AudioNodeOptions {float pan = 0; };
1.30.3.1. Dictionary StereoPannerOptions
Members
pan
, of type float, defaulting to0
-
The initial value for the
pan
AudioParam.
1.30.4. Channel Limitations
Because its processing is constrained by the above definitions, StereoPannerNode
is limited to mixing no more
than 2 channels of audio, and producing exactly 2 channels. It is
possible to use a ChannelSplitterNode
,
intermediate processing by a subgraph of GainNode
s and/or other nodes, and recombination
via a ChannelMergerNode
to realize arbitrary
approaches to panning and mixing.
1.31. The WaveShaperNode
Interface
WaveShaperNode
is an AudioNode
processor implementing non-linear
distortion effects.
Non-linear waveshaping distortion is commonly used for both subtle non-linear warming, or more obvious distortion effects. Arbitrary non-linear shaping curves may be specified.
Property | Value | Notes |
---|---|---|
numberOfInputs
| 1 | |
numberOfOutputs
| 1 | |
channelCount
| 2 | |
channelCountMode
| "max "
| |
channelInterpretation
| "speakers "
| |
tail-time | Maybe | There is a tail-time only if the oversample attribute is set to "2x " or "4x ". The actual duration of this tail-time depends on the implementation.
|
The number of channels of the output always equals the number of channels of the input.
enum {
OverSampleType "none" ,"2x" ,"4x" };
Enum value | Description |
---|---|
"none "
| Don’t oversample |
"2x "
| Oversample two times |
"4x "
| Oversample four times |
[Exposed =Window ]interface WaveShaperNode :AudioNode {constructor (BaseAudioContext ,
context optional WaveShaperOptions = {});
options attribute Float32Array ?curve ;attribute OverSampleType oversample ; };
1.31.1. Constructors
WaveShaperNode(context, options)
-
When the constructor is called with a
BaseAudioContext
c and an option object option, the user agent MUST initialize the AudioNode this, with context and options as arguments.Also, let
[[curve set]]
be an internal slot of thisWaveShaperNode
. Initialize this slot tofalse
. Ifoptions
is given and specifies acurve
, set[[curve set]]
totrue
.Arguments for the WaveShaperNode.constructor() method. Parameter Type Nullable Optional Description context
BaseAudioContext
✘ ✘ The BaseAudioContext
this newWaveShaperNode
will be associated with.options
WaveShaperOptions
✘ ✔ Optional initial parameter value for this WaveShaperNode
.
1.31.2. Attributes
curve
, of type Float32Array, nullable-
The shaping curve used for the waveshaping effect. The input signal is nominally within the range [-1, 1]. Each input sample within this range will index into the shaping curve, with a signal level of zero corresponding to the center value of the curve array if there are an odd number of entries, or interpolated between the two centermost values if there are an even number of entries in the array. Any sample value less than -1 will correspond to the first value in the curve array. Any sample value greater than +1 will correspond to the last value in the curve array.
The implementation MUST perform linear interpolation between adjacent points in the curve. Initially the curve attribute is null, which means that the WaveShaperNode will pass its input to its output without modification.
Values of the curve are spread with equal spacing in the [-1; 1] range. This means that a
curve
with a even number of value will not have a value for a signal at zero, and acurve
with an odd number of value will have a value for a signal at zero. The output is determined by the following algorithm.-
Let \(x\) be the input sample, \(y\) be the corresponding output of the node, \(c_k\) be the \(k\)'th element of the
curve
, and \(N\) be the length of thecurve
. -
Let
$$ \begin{align*} v &= \frac{N-1}{2}(x + 1) \\ k &= \lfloor v \rfloor \\ f &= v - k \end{align*} $$
-
Then
$$ \begin{align*} y &= \begin{cases} c_0 & v \lt 0 \\ c_{N-1} & v \ge N - 1 \\ (1-f)\,c_k + fc_{k+1} & \mathrm{otherwise} \end{cases} \end{align*} $$
A
InvalidStateError
MUST be thrown if this attribute is set with aFloat32Array
that has alength
less than 2.When this attribute is set, an internal copy of the curve is created by the
WaveShaperNode
. Subsequent modifications of the contents of the array used to set the attribute therefore have no effect.To set thecurve
attribute, execute these steps:-
Let new curve be a
Float32Array
to be assigned tocurve
ornull
. . -
If new curve is not
null
and[[curve set]]
is true, throw anInvalidStateError
and abort these steps. -
If new curve is not
null
, set[[curve set]]
to true. -
Assign new curve to the
curve
attribute.
Note: The use of a curve that produces a non-zero output value for zero input value will cause this node to produce a DC signal even if there are no inputs connected to this node. This will persist until the node is disconnected from downstream nodes.
-
oversample
, of type OverSampleType-
Specifies what type of oversampling (if any) should be used when applying the shaping curve. The default value is "
none
", meaning the curve will be applied directly to the input samples. A value of "2x
" or "4x
" can improve the quality of the processing by avoiding some aliasing, with the "4x
" value yielding the highest quality. For some applications, it’s better to use no oversampling in order to get a very precise shaping curve. -
Up-sample the input samples to 2x or 4x the sample-rate of the
AudioContext
. Thus for each render quantum, generate 256 (for 2x) or 512 (for 4x) samples. -
Apply the shaping curve.
-
Down-sample the result back to the sample-rate of the
AudioContext
. Thus taking the 256 (or 512) processed samples, generating 128 as the final result. -
Up-sample the input samples to 2x or 4x the sample-rate of the
AudioContext
. Thus for each render quantum, generate twice (for 2x) or four times (for 4x) samples. -
Apply the shaping curve.
-
Down-sample the result back to the sample-rate of the
AudioContext
. Thus taking the previously processed samples processed samples, generating a single render quantum worth of samples as the final result.
The exact up-sampling and down-sampling filters are not specified, and can be tuned for sound quality (low aliasing, etc.), low latency, or performance.
Note: Use of oversampling introduces some degree of audio processing latency due to the up-sampling and down-sampling filters. The amount of this latency can vary from one implementation to another.
1.31.3. WaveShaperOptions
This specifies the options for constructing a WaveShaperNode
. All members are optional; if
not specified, the normal default is used in constructing the node.
dictionary WaveShaperOptions :AudioNodeOptions {sequence <float >curve ;OverSampleType oversample = "none"; };
1.31.3.1. Dictionary WaveShaperOptions
Members
curve
, of type sequence<float>-
The shaping curve for the waveshaping effect.
oversample
, of type OverSampleType, defaulting to"none"
-
The type of oversampling to use for the shaping curve.
1.32. The AudioWorklet
Interface
[Exposed =Window ,SecureContext ]interface AudioWorklet :Worklet { };
[Exposed =Window ,SecureContext ]interface AudioWorklet :Worklet {readonly attribute MessagePort port ; };
1.32.1. Attributes
port
, of type MessagePort, readonly-
A
MessagePort
connected to the port on theAudioWorkletGlobalScope
.Note: Authors that register an event listener on the
"message"
event of thisport
should callclose
on either end of theMessageChannel
(either in theAudioWorklet
or theAudioWorkletGlobalScope
side) to allow for resources to be collected.
1.32.2. Concepts
The AudioWorklet
object allows developers to supply scripts
(such as JavaScript or WebAssembly code) to process audio on the rendering thread, supporting custom AudioNode
s. This
processing mechanism ensures synchronous execution of the
script code with other built-in AudioNode
s in the audio
graph.
An associated pair of objects MUST be defined in order to realize
this mechanism: AudioWorkletNode
and AudioWorkletProcessor
. The former represents the interface
for the main global scope similar to other AudioNode
objects, and the latter implements the internal audio processing
within a special scope named AudioWorkletGlobalScope
.

AudioWorkletNode
and AudioWorkletProcessor
Each BaseAudioContext
possesses exactly one AudioWorklet
.
The AudioWorklet
's worklet global scope type is AudioWorkletGlobalScope
.
The AudioWorklet
's worklet destination type is "audioworklet"
.
Importing a script via the addModule(moduleUrl)
method registers class definitions of AudioWorkletProcessor
under the AudioWorkletGlobalScope
. There are two internal
storage areas for the imported class constructor and the active
instances created from the constructor.
AudioWorklet
has one internal slot:
-
node name to parameter descriptor map which is a map containing an identical set of string keys from node name to processor constructor map that are associated with the matching parameterDescriptors values. This internal storage is populated as a consequence of calling the
registerProcessor()
method in the rendering thread. The population is guaranteed to complete prior to the resolution of the promise returned byaddModule()
on a context’saudioWorklet
.
// bypass-processor.js script file, runs on AudioWorkletGlobalScope class BypassProcessorextends AudioWorkletProcessor{ process( inputs, outputs) { // Single input, single channel. const input= inputs[ 0 ]; const output= outputs[ 0 ]; output[ 0 ]. set( input[ 0 ]); // Process only while there are active inputs. return false ; } }; registerProcessor( 'bypass-processor' , BypassProcessor);
// The main global scope const context= new AudioContext(); context. audioWorklet. addModule( 'bypass-processor.js' ). then(() => { const bypassNode= new AudioWorkletNode( context, 'bypass-processor' ); });
At the instantiation of AudioWorkletNode
in the main global
scope, the counterpart AudioWorkletProcessor
will also be
created in AudioWorkletGlobalScope
. These two objects
communicate via the asynchronous message passing described in § 2 Processing model.
1.32.3. The AudioWorkletGlobalScope
Interface
This special execution context is designed to enable the
generation, processing, and analysis of audio data directly using a
script in the audio rendering thread. The user-supplied
script code is evaluated in this scope to define one or more AudioWorkletProcessor
subclasses, which in turn are used to
instantiate AudioWorkletProcessor
s, in a 1:1 association
with AudioWorkletNode
s in the main scope.
Exactly one AudioWorkletGlobalScope
exists for each AudioContext
that contains one or more AudioWorkletNode
s. The running of imported scripts is
performed by the UA as defined in [HTML]. Overriding the default
specified in [HTML], AudioWorkletGlobalScope
s must not be terminated arbitrarily by the user
agent.
An AudioWorkletGlobalScope
has the following internal slots:
-
node name to processor constructor map which is a map that stores key-value pairs of processor name →
AudioWorkletProcessorConstructor
instance. Initially this map is empty and populated when theregisterProcessor()
method is called. -
pending processor construction data stores temporary data generated by the
AudioWorkletNode
constructor for the instantiation of the correspondingAudioWorkletProcessor
. The pending processor construction data contains the following items:-
node reference which is initially empty. This storage is for an
AudioWorkletNode
reference that is transferred from theAudioWorkletNode
constructor. -
transferred port which is initially empty. This storage is for a deserialized
MessagePort
that is transferred from theAudioWorkletNode
constructor.
-
Note: The AudioWorkletGlobalScope
may also contain any other data
and code to be shared by these instances. As an example, multiple
processors might share an ArrayBuffer defining a wavetable or an
impulse response.
Note: An AudioWorkletGlobalScope
is associated with a single BaseAudioContext
, and with a single audio rendering thread
for that context. This prevents data races from occurring in global
scope code running within concurrent threads.
callback AudioWorkletProcessorConstructor =AudioWorkletProcessor (object options ); [Global =(Worklet ,AudioWorklet ),Exposed =AudioWorklet ]interface AudioWorkletGlobalScope :WorkletGlobalScope {undefined registerProcessor (DOMString name ,AudioWorkletProcessorConstructor processorCtor );readonly attribute unsigned long long currentFrame ;readonly attribute double currentTime ;readonly attribute float sampleRate ; };
callback =
AudioWorkletProcessorConstructor AudioWorkletProcessor (object ); [
options Global =(Worklet ,AudioWorklet ),Exposed =AudioWorklet ]interface AudioWorkletGlobalScope :WorkletGlobalScope {undefined registerProcessor (DOMString name ,AudioWorkletProcessorConstructor processorCtor );readonly attribute unsigned long long currentFrame ;readonly attribute double currentTime ;readonly attribute float sampleRate ;readonly attribute unsigned long renderQuantumSize ;readonly attribute MessagePort port ; };
1.32.3.1. Attributes
currentFrame
, of type unsigned long long, readonly-
The current frame of the block of audio being processed. This must be equal to the value of the
[[current frame]]
internal slot of theBaseAudioContext
. currentTime
, of type double, readonly-
The context time of the block of audio being processed. By definition this will be equal to the value of
BaseAudioContext
'scurrentTime
attribute that was most recently observable in the control thread. sampleRate
, of type float, readonly-
The sample rate of the associated
BaseAudioContext
. renderQuantumSize
, of type unsigned long, readonly-
The value of the private slot [[render quantum size]] of the associated
BaseAudioContext
. port
, of type MessagePort, readonly-
A
MessagePort
connected to the port on theAudioWorklet
.Note: Authors that register an event listener on the
"message"
event of thisport
should callclose
on either end of theMessageChannel
(either in theAudioWorklet
or theAudioWorkletGlobalScope
side) to allow for resources to be collected.
1.32.3.2. Methods
registerProcessor(name, processorCtor)
-
Registers a class constructor derived from
AudioWorkletProcessor
.When theregisterProcessor(name, processorCtor)
method is called, perform the following steps. If an exception is thrown in any step, abort the remaining steps.-
If name is an empty string, throw a
NotSupportedError
. -
If name already exists as a key in the node name to processor constructor map, throw a
NotSupportedError
. -
If the result of
IsConstructor(argument=processorCtor)
isfalse
, throw aTypeError
. -
Let
prototype
be the result ofGet(O=processorCtor, P="prototype")
. -
If the result of
Type(argument=prototype)
is notObject
, throw aTypeError
. -
Let parameterDescriptorsValue be the result of
Get(O=processorCtor, P="parameterDescriptors")
. -
If parameterDescriptorsValue is not
undefined
, execute the following steps:-
Let parameterDescriptorSequence be the result of the conversion from parameterDescriptorsValue to an IDL value of type
sequence<AudioParamDescriptor>
. -
Let paramNames be an empty Array.
-
For each descriptor of parameterDescriptorSequence:
-
Let paramName be the value of the member
name
in descriptor. Throw aNotSupportedError
if paramNames already contains paramName value. -
Append paramName to the paramNames array.
-
Let defaultValue be the value of the member
defaultValue
in descriptor. -
Let minValue be the value of the member
minValue
in descriptor. -
Let maxValue be the value of the member
maxValue
in descriptor. -
If the expresstion minValue <= defaultValue <= maxValue is false, throw an
InvalidStateError
.
-
-
-
Append the key-value pair name → processorCtor to node name to processor constructor map of the associated
AudioWorkletGlobalScope
. -
queue a media element task to append the key-value pair name → parameterDescriptorSequence to the node name to parameter descriptor map of the associated
BaseAudioContext
.
Note: The class constructor should only be looked up once, thus it does not have the opportunity to dynamically change after registration.
Arguments for the AudioWorkletGlobalScope.registerProcessor(name, processorCtor) method. Parameter Type Nullable Optional Description name
DOMString
✘ ✘ A string key that represents a class constructor to be registered. This key is used to look up the constructor of AudioWorkletProcessor
during construction of anAudioWorkletNode
.processorCtor
AudioWorkletProcessorConstructor
✘ ✘ A class constructor extended from AudioWorkletProcessor
.Return type:undefined
-
1.32.3.3. The instantiation of AudioWorkletProcessor
At the end of the AudioWorkletNode
construction,
A struct named processor construction data will be prepared for cross-thread transfer. This struct contains the following items:
-
name which is a
DOMString
that is to be looked up in the node name to processor constructor map. -
node which is a reference to the
AudioWorkletNode
created. -
options which is a serialized
AudioWorkletNodeOptions
given to theAudioWorkletNode
'sconstructor
. -
port which is a serialized
MessagePort
paired with theAudioWorkletNode
'sport
.
Upon the arrival of the transferred data on the AudioWorkletGlobalScope
,
the rendering thread will invoke the algorithm below:
-
Let constructionData be the processor construction data transferred from the control thread.
-
Let processorName, nodeReference and serializedPort be constructionData’s name, node, and port respectively.
-
Let serializedOptions be constructionData’s options.
-
Let deserializedPort be the result of StructuredDeserialize(serializedPort, the current Realm).
-
Let deserializedOptions be the result of StructuredDeserialize(serializedOptions, the current Realm).
-
Let processorCtor be the result of looking up processorName on the
AudioWorkletGlobalScope
's node name to processor constructor map. -
Store nodeReference and deserializedPort to node reference and transferred port of this
AudioWorkletGlobalScope
's pending processor construction data respectively. -
Construct a callback function from processorCtor with the argument of deserializedOptions. If any exceptions are thrown in the callback, queue a task to the control thread to fire an event named
processorerror
usingErrorEvent
at nodeReference. -
Empty the pending processor construction data slot.
1.32.4. The AudioWorkletNode
Interface
This interface represents a user-defined AudioNode
which
lives on the control thread. The user can create an AudioWorkletNode
from a BaseAudioContext
, and such a
node can be connected with other built-in AudioNode
s to form
an audio graph.
Property | Value | Notes |
---|---|---|
numberOfInputs
| 1 | |
numberOfOutputs
| 1 | |
channelCount
| 2 | |
channelCountMode
| "max "
| |
channelInterpretation
| "speakers "
| |
tail-time | See notes | Any tail-time is handled by the node itself |
Every AudioWorkletProcessor
has an associated active source flag, initially true
. This flag causes
the node to be retained in memory and perform audio processing in
the absence of any connected inputs.
All tasks posted from an AudioWorkletNode
are posted to the task queue of
its associated BaseAudioContext
.
[Exposed =Window ]interface {
AudioParamMap readonly maplike <DOMString ,AudioParam >; };
This interface has "entries", "forEach", "get", "has", "keys",
"values", @@iterator methods and a "size" getter brought by readonly maplike
.
[Exposed =Window ,SecureContext ]interface AudioWorkletNode :AudioNode {constructor (BaseAudioContext ,
context DOMString ,
name optional AudioWorkletNodeOptions = {});
options readonly attribute AudioParamMap parameters ;readonly attribute MessagePort port ;attribute EventHandler onprocessorerror ; };
1.32.4.1. Constructors
AudioWorkletNode(context, name, options)
-
Arguments for the AudioWorkletNode.constructor() method. Parameter Type Nullable Optional Description context
BaseAudioContext
✘ ✘ The BaseAudioContext
this newAudioWorkletNode
will be associated with.name
DOMString
✘ ✘ A string that is a key for the BaseAudioContext
’s node name to parameter descriptor map.options
AudioWorkletNodeOptions
✘ ✔ Optional initial parameters value for this AudioWorkletNode
.When the constructor is called, the user agent MUST perform the following steps on the control thread:
When theAudioWorkletNode
constructor is invoked with context, nodeName, options:-
If nodeName does not exist as a key in the
BaseAudioContext
’s node name to parameter descriptor map, throw aInvalidStateError
exception and abort these steps. -
Let node be this value.
-
Initialize the AudioNode node with context and options as arguments.
-
Configure input, output and output channels of node with options. Abort the remaining steps if any exception is thrown.
-
Let messageChannel be a new
MessageChannel
. -
Let nodePort be the value of messageChannel’s
port1
attribute. -
Let processorPortOnThisSide be the value of messageChannel’s
port2
attribute. -
Let serializedProcessorPort be the result of StructuredSerializeWithTransfer(processorPortOnThisSide, « processorPortOnThisSide »).
-
Convert options dictionary to optionsObject.
-
Let serializedOptions be the result of StructuredSerialize(optionsObject).
-
Set node’s
port
to nodePort. -
Let parameterDescriptors be the result of retrieval of nodeName from node name to parameter descriptor map:
-
Let audioParamMap be a new
AudioParamMap
object. -
For each descriptor of parameterDescriptors:
-
Let paramName be the value of
name
member in descriptor. -
Let audioParam be a new
AudioParam
instance withautomationRate
,defaultValue
,minValue
, andmaxValue
having values equal to the values of corresponding members on descriptor. -
Append a key-value pair paramName → audioParam to audioParamMap’s entries.
-
-
If
parameterData
is present on options, perform the following steps:-
Let parameterData be the value of
parameterData
. -
For each paramName → paramValue of parameterData:
-
If there exists a map entry on audioParamMap with key paramName, let audioParamInMap be such entry.
-
Set
value
property of audioParamInMap to paramValue.
-
-
-
Set node’s
parameters
to audioParamMap.
-
-
Queue a control message to invoke the
constructor
of the correspondingAudioWorkletProcessor
with the processor construction data that consists of: nodeName, node, serializedOptions, and serializedProcessorPort.
-
1.32.4.2. Attributes
onprocessorerror
, of type EventHandler-
When an unhandled exception is thrown from the processor’s
constructor
,process
method, or any user-defined class method, the processor will queue a media element task to fire an event namedprocessorerror
using ErrorEvent at the associatedAudioWorkletNode
.The
ErrorEvent
is created and initialized appropriately with itsmessage
,filename
,lineno
,colno
attributes on the control thread.Note that once a unhandled exception is thrown, the processor will output silence throughout its lifetime.
parameters
, of type AudioParamMap, readonly-
The
parameters
attribute is a collection ofAudioParam
objects with associated names. This maplike object is populated from a list ofAudioParamDescriptor
s in theAudioWorkletProcessor
class constructor at the instantiation. port
, of type MessagePort, readonly-
Every
AudioWorkletNode
has an associatedport
which is theMessagePort
. It is connected to the port on the correspondingAudioWorkletProcessor
object allowing bidirectional communication between theAudioWorkletNode
and itsAudioWorkletProcessor
.Note: Authors that register an event listener on the
"message"
event of thisport
should callclose
on either end of theMessageChannel
(either in theAudioWorkletProcessor
or theAudioWorkletNode
side) to allow for resources to be collected.
1.32.4.3. AudioWorkletNodeOptions
The AudioWorkletNodeOptions
dictionary can be used
to initialize attibutes in the instance of an AudioWorkletNode
.
dictionary AudioWorkletNodeOptions :AudioNodeOptions {unsigned long numberOfInputs = 1;unsigned long numberOfOutputs = 1;sequence <unsigned long >outputChannelCount ;record <DOMString ,double >parameterData ;object processorOptions ; };
1.32.4.3.1. Dictionary AudioWorkletNodeOptions
Members
numberOfInputs
, of type unsigned long, defaulting to1
-
This is used to initialize the value of the
AudioNode
numberOfInputs
attribute. numberOfOutputs
, of type unsigned long, defaulting to1
-
This is used to initialize the value of the
AudioNode
numberOfOutputs
attribute. outputChannelCount
, of typesequence<unsigned long>
-
This array is used to configure the number of channels in each output.
parameterData
, of type record<DOMString, double>-
This is a list of user-defined key-value pairs that are used to set the initial
value
of anAudioParam
with the matched name in theAudioWorkletNode
. processorOptions
, of type object-
This holds any user-defined data that may be used to initialize custom properties in an
AudioWorkletProcessor
instance that is associated with theAudioWorkletNode
.
1.32.4.3.2. Configuring Channels with AudioWorkletNodeOptions
The following algorithm describes how an AudioWorkletNodeOptions
can be
used to configure various channel configurations.
-
Let node be an
AudioWorkletNode
instance that is given to this algorithm. -
If both
numberOfInputs
andnumberOfOutputs
are zero, throw aNotSupportedError
and abort the remaining steps. -
-
If any value in
outputChannelCount
is zero or greater than the implementation’s maximum number of channels, throw aNotSupportedError
and abort the remaining steps. -
If the length of
outputChannelCount
does not equalnumberOfOutputs
, throw anIndexSizeError
and abort the remaining steps. -
If both
numberOfInputs
andnumberOfOutputs
are 1, set the channel count of the node output to the one value inoutputChannelCount
. -
Otherwise set the channel count of the kth output of the node to the kth element of
outputChannelCount
sequence and return.
-
-
If
outputChannelCount
does not exists,-
If both
numberOfInputs
andnumberOfOutputs
are 1, set the initial channel count of the node output to 1 and return.NOTE: For this case, the output chanel count will change to computedNumberOfChannels dynamically based on the input and the
channelCountMode
at runtime. -
Otherwise set the channel count of each output of the node to 1 and return.
-
1.32.5. The AudioWorkletProcessor
Interface
This interface represents an audio processing code that runs on the
audio rendering thread. It lives in the AudioWorkletGlobalScope
,
and the definition of the class manifests the actual audio processing.
Note that the an AudioWorkletProcessor
construction can only happen as a
result of an AudioWorkletNode
contruction.
[Exposed =AudioWorklet ]interface AudioWorkletProcessor {constructor ();readonly attribute MessagePort port ; };callback AudioWorkletProcessCallback =boolean (FrozenArray <FrozenArray <Float32Array >>,
inputs FrozenArray <FrozenArray <Float32Array >>,
outputs object );
parameters
AudioWorkletProcessor
has two internal slots:
[[node reference]]
-
A reference to the associated
AudioWorkletNode
. [[callable process]]
-
A boolean flag representing whether process() is a valid function that can be invoked.
1.32.5.1. Constructors
AudioWorkletProcessor()
-
When the constructor for
AudioWorkletProcessor
is invoked, the following steps are performed on the rendering thread.-
Let nodeReference be the result of looking up node reference on the pending processor construction data of the current
AudioWorkletGlobalScope
. Throw aTypeError
exception if the slot is empty. -
Let processor be the this value.
-
Set processor’s
[[node reference]]
to nodeReference. -
Set processor’s
[[callable process]]
totrue
. -
Let deserializedPort be the result of looking up transferred port from the pending processor construction data.
-
Set processor’s
port
to deserializedPort. -
Empty the pending processor construction data slot.
-
1.32.5.2. Attributes
port
, of type MessagePort, readonly-
Every
AudioWorkletProcessor
has an associatedport
which is aMessagePort
. It is connected to the port on the correspondingAudioWorkletNode
object allowing bidirectional communication between anAudioWorkletNode
and itsAudioWorkletProcessor
.Note: Authors that register an event listener on the
"message"
event of thisport
should callclose
on either end of theMessageChannel
(either in theAudioWorkletProcessor
or theAudioWorkletNode
side) to allow for resources to be collected.
1.32.5.3. Callback AudioWorkletProcessCallback
Users can define a custom audio processor by extending AudioWorkletProcessor
. The subclass MUST define an AudioWorkletProcessCallback
named process()
that implements the audio processing
algorithm and may have a static property named parameterDescriptors
which is an iterable
of AudioParamDescriptor
s.
The process() callback function is handled as specified when rendering a graph.
AudioWorkletProcessor
's associated AudioWorkletNode
.
This lifetime policy can support a variety of approaches found in built-in nodes, including the following:
-
Nodes that transform their inputs, and are active only while connected inputs and/or script references exist. Such nodes SHOULD return
false
from process() which allows the presence or absence of connected inputs to determine whether theAudioWorkletNode
is actively processing. -
Nodes that transform their inputs, but which remain active for a tail-time after their inputs are disconnected. In this case, process() SHOULD return
true
for some period of time afterinputs
is found to contain zero channels. The current time may be obtained from the global scope’scurrentTime
to measure the start and end of this tail-time interval, or the interval could be calculated dynamically depending on the processor’s internal state. -
Nodes that act as sources of output, typically with a lifetime. Such nodes SHOULD return
true
from process() until the point at which they are no longer producing an output.
Note that the preceding definition implies that when no
return value is provided from an implementation of process(), the effect is identical to returning false
(since the effective return value is the falsy
value undefined
). This is a reasonable behavior for
any AudioWorkletProcessor
that is active only when it has
active inputs.
The example below shows how AudioParam
can be defined and used in an AudioWorkletProcessor
.
class MyProcessorextends AudioWorkletProcessor{ static get parameterDescriptors() { return [{ name: 'myParam' , defaultValue: 0.5 , minValue: 0 , maxValue: 1 , automationRate: "k-rate" }]; } process( inputs, outputs, parameters) { // Get the first input and output. const input= inputs[ 0 ]; const output= outputs[ 0 ]; const myParam= parameters. myParam; // A simple amplifier for single input and output. Note that the // automationRate is "k-rate", so it will have a single value at index [0] // for each render quantum. for ( let channel= 0 ; channel< output. length; ++ channel) { for ( let i= 0 ; i< output[ channel]. length; ++ i) { output[ channel][ i] = input[ channel][ i] * myParam[ 0 ]; } } } }
1.32.5.3.1. Callback AudioWorkletProcessCallback
Parameters
The following describes the parameters to the AudioWorkletProcessCallback
function.
In general, the inputs
and outputs
arrays will be reused
between calls so that no memory allocation is done. However, if the
topology changes, because, say, the number of channels in the input or the
output changes, new arrays are reallocated. New arrays are also
reallocated if any part of the inputs
or outputs
arrays are
transferred.
inputs
, of typeFrozenArray
<FrozenArray
<Float32Array
>>-
The input audio buffer from the incoming connections provided by the user agent.
inputs[n][m]
is aFloat32Array
of audio samples for the \(m\)th channel of the \(n\)th input. While the number of inputs is fixed at construction, the number of channels can be changed dynamically based on computedNumberOfChannels.If there are no actively processing
AudioNode
s connected to the \(n\)th input of theAudioWorkletNode
for the current render quantum, then the content ofinputs[n]
is an empty array, indicating that zero channels of input are available. This is the only circumstance under which the number of elements ofinputs[n]
can be zero. outputs
, of typeFrozenArray
<FrozenArray
<Float32Array
>>-
The output audio buffer that is to be consumed by the user agent.
outputs[n][m]
is aFloat32Array
object containing the audio samples for \(m\)th channel of \(n\)th output. Each of theFloat32Array
s are zero-filled. The number of channels in the output will match computedNumberOfChannels only when the node has a single output. parameters
, of typeobject
-
An ordered map of name → parameterValues.
parameters["name"]
returns parameterValues, which is aFrozenArray
<Float32Array
> with the automation values of the nameAudioParam
.For each array, the array contains the computedValue of the parameter for all frames in the render quantum. However, if no automation is scheduled during this render quantum, the array MAY have length 1 with the array element being the constant value of the
AudioParam
for the render quantum.This object is frozen according the the following steps
-
Let parameter be the ordered map of the name and parameter values.
-
SetIntegrityLevel(parameter, frozen)
This frozen ordered map computed in the algorithm is passed to the
parameters
argument.Note: This means the object cannot be modified and hence the same object can be used for successive calls unless length of an array changes.
-
1.32.5.4. AudioParamDescriptor
The AudioParamDescriptor
dictionary is used to
specify properties for an AudioParam
object
that is used in an AudioWorkletNode
.
dictionary AudioParamDescriptor {required DOMString name ;float defaultValue = 0;float minValue = -3.4028235e38;float maxValue = 3.4028235e38;AutomationRate automationRate = "a-rate"; };
1.32.5.4.1. Dictionary AudioParamDescriptor
Members
There are constraints on the values for these members. See the algorithm for handling an AudioParamDescriptor for the constraints.
automationRate
, of type AutomationRate, defaulting to"a-rate"
-
Represents the default automation rate.
defaultValue
, of type float, defaulting to0
-
Represents the default value of the parameter.
maxValue
, of type float, defaulting to3.4028235e38
-
Represents the maximum value.
minValue
, of type float, defaulting to-3.4028235e38
-
Represents the minimum value.
name
, of type DOMString-
Represents the name of the parameter.
1.32.6. AudioWorklet Sequence of Events
The following figure illustrates an idealized sequence of events
occurring relative to an AudioWorklet
:
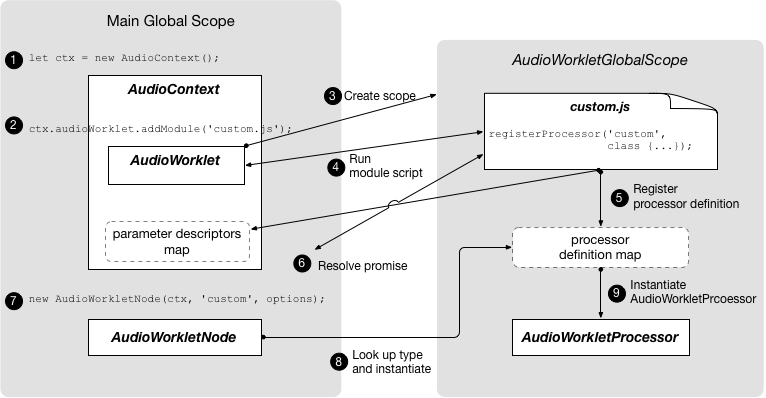
AudioWorklet
sequence The steps depicted in the diagram are one possible sequence of
events involving the creation of an AudioContext
and an
associated AudioWorkletGlobalScope
, followed by the creation
of an AudioWorkletNode
and its associated AudioWorkletProcessor
.
-
An
AudioContext
is created. -
In the main scope,
context.audioWorklet
is requested to add a script module. -
Since none exists yet, a new
AudioWorkletGlobalScope
is created in association with the context. This is the global scope in whichAudioWorkletProcessor
class definitions will be evaluated. (On subsequent calls, this previously created scope will be used.) -
The imported script is run in the newly created global scope.
-
As part of running the imported script, an
AudioWorkletProcessor
is registered under a key ("custom"
in the above diagram) within theAudioWorkletGlobalScope
. This populates maps both in the global scope and in theAudioContext
. -
The promise for the
addModule()
call is resolved. -
In the main scope, an
AudioWorkletNode
is created using the user-specified key along with a dictionary of options. -
As part of the node’s creation, this key is used to look up the correct
AudioWorkletProcessor
subclass for instantiation. -
An instance of the
AudioWorkletProcessor
subclass is instantiated with a structured clone of the same options dictionary. This instance is paired with the previously createdAudioWorkletNode
.
1.32.7. AudioWorklet Examples
1.32.7.1. The BitCrusher Node
Bitcrushing is a mechanism by which the quality of an audio
stream is reduced both by quantizing the sample value (simulating
a lower bit-depth), and by quantizing in time resolution
(simulating a lower sample rate). This example shows how to use AudioParam
s (in this case, treated as a-rate) inside an AudioWorkletProcessor
.
const context= new AudioContext(); context. audioWorklet. addModule( 'bitcrusher.js' ). then(() => { const osc= new OscillatorNode( context); const amp= new GainNode( context); // Create a worklet node. 'BitCrusher' identifies the // AudioWorkletProcessor previously registered when // bitcrusher.js was imported. The options automatically // initialize the correspondingly named AudioParams. const bitcrusher= new AudioWorkletNode( context, 'bitcrusher' , { parameterData: { bitDepth: 8 } }); osc. connect( bitcrusher). connect( amp). connect( context. destination); osc. start(); });
class Bitcrusherextends AudioWorkletProcessor{ static get parameterDescriptors() { return [{ name: 'bitDepth' , defaultValue: 12 , minValue: 1 , maxValue: 16 }, { name: 'frequencyReduction' , defaultValue: 0.5 , minValue: 0 , maxValue: 1 }]; } constructor ( options) { // The initial parameter value can be set by passing |options| // to the processor’s constructor. super ( options); this . _phase= 0 ; this . _lastSampleValue= 0 ; } process( inputs, outputs, parameters) { const input= inputs[ 0 ]; const output= outputs[ 0 ]; const bitDepth= parameters. bitDepth; const frequencyReduction= parameters. frequencyReduction; if ( bitDepth. length> 1 ) { for ( let channel= 0 ; channel< output. length; ++ channel) { for ( let i= 0 ; i< output[ channel]. length; ++ i) { let step= Math. pow( 0.5 , bitDepth[ i]); // Use modulo for indexing to handle the case where // the length of the frequencyReduction array is 1. this . _phase+= frequencyReduction[ i% frequencyReduction. length]; if ( this . _phase>= 1.0 ) { this . _phase-= 1.0 ; this . _lastSampleValue= step* Math. floor( input[ channel][ i] / step+ 0.5 ); } output[ channel][ i] = this . _lastSampleValue; } } } else { // Because we know bitDepth is constant for this call, // we can lift the computation of step outside the loop, // saving many operations. const step= Math. pow( 0.5 , bitDepth[ 0 ]); for ( let channel= 0 ; channel< output. length; ++ channel) { for ( let i= 0 ; i< output[ channel]. length; ++ i) { this . _phase+= frequencyReduction[ i% frequencyReduction. length]; if ( this . _phase>= 1.0 ) { this . _phase-= 1.0 ; this . _lastSampleValue= step* Math. floor( input[ channel][ i] / step+ 0.5 ); } output[ channel][ i] = this . _lastSampleValue; } } } // No need to return a value; this node’s lifetime is dependent only on its // input connections. } }; registerProcessor( 'bitcrusher' , Bitcrusher);
Note: In the definition of AudioWorkletProcessor
class, an InvalidStateError
will be thrown if the
author-supplied constructor has an explicit return value that is not this
or does not properly call super()
.
1.32.7.2. VU Meter Node
This example of a simple sound level meter further illustrates
how to create an AudioWorkletNode
subclass
that acts like a native AudioNode
, accepting
constructor options and encapsulating the inter-thread
communication (asynchronous) between AudioWorkletNode
and AudioWorkletProcessor
. This node does not use any output.
/* vumeter-node.js: Main global scope */ export default class VUMeterNodeextends AudioWorkletNode{ constructor ( context, updateIntervalInMS) { super ( context, 'vumeter' , { numberOfInputs: 1 , numberOfOutputs: 0 , channelCount: 1 , processorOptions: { updateIntervalInMS: updateIntervalInMS|| 16.67 ; } }); // States in AudioWorkletNode this . _updateIntervalInMS= updateIntervalInMS; this . _volume= 0 ; // Handles updated values from AudioWorkletProcessor this . port. onmessage= event=> { if ( event. data. volume) this . _volume= event. data. volume; } this . port. start(); } get updateInterval() { return this . _updateIntervalInMS; } set updateInterval( updateIntervalInMS) { this . _updateIntervalInMS= updateIntervalInMS; this . port. postMessage({ updateIntervalInMS: updateIntervalInMS}); } draw() { // Draws the VU meter based on the volume value // every |this._updateIntervalInMS| milliseconds. } };
/* vumeter-processor.js: AudioWorkletGlobalScope */ const SMOOTHING_FACTOR= 0.9 ; const MINIMUM_VALUE= 0.00001 ; registerProcessor( 'vumeter' , class extends AudioWorkletProcessor{ constructor ( options) { super (); this . _volume= 0 ; this . _updateIntervalInMS= options. processorOptions. updateIntervalInMS; this . _nextUpdateFrame= this . _updateIntervalInMS; this . port. onmessage= event=> { if ( event. data. updateIntervalInMS) this . _updateIntervalInMS= event. data. updateIntervalInMS; } } get intervalInFrames() { return this . _updateIntervalInMS/ 1000 * sampleRate; } process( inputs, outputs, parameters) { const input= inputs[ 0 ]; // Note that the input will be down-mixed to mono; however, if no inputs are // connected then zero channels will be passed in. if ( input. length> 0 ) { const samples= input[ 0 ]; let sum= 0 ; let rms= 0 ; // Calculated the squared-sum. for ( let i= 0 ; i< samples. length; ++ i) sum+= samples[ i] * samples[ i]; // Calculate the RMS level and update the volume. rms= Math. sqrt( sum/ samples. length); this . _volume= Math. max( rms, this . _volume* SMOOTHING_FACTOR); // Update and sync the volume property with the main thread. this . _nextUpdateFrame-= samples. length; if ( this . _nextUpdateFrame< 0 ) { this . _nextUpdateFrame+= this . intervalInFrames; this . port. postMessage({ volume: this . _volume}); } } // Keep on processing if the volume is above a threshold, so that // disconnecting inputs does not immediately cause the meter to stop // computing its smoothed value. return this . _volume>= MINIMUM_VALUE; } });
/* index.js: Main global scope, entry point */ import VUMeterNodefrom './vumeter-node.js' ; const context= new AudioContext(); context. audioWorklet. addModule( 'vumeter-processor.js' ). then(() => { const oscillator= new OscillatorNode( context); const vuMeterNode= new VUMeterNode( context, 25 ); oscillator. connect( vuMeterNode); oscillator. start(); function drawMeter() { vuMeterNode. draw(); requestAnimationFrame( drawMeter); } drawMeter(); });
2. Processing model
2.1. Background
This section is non-normative.
Real-time audio systems that require low latency are often implemented using callback functions, where the operating system calls the program back when more audio has to be computed in order for the playback to stay uninterrupted. Such a callback is ideally called on a high priority thread (often the highest priority on the system). This means that a program that deals with audio only executes code from this callback. Crossing thread boundaries or adding some buffering between a rendering thread and the callback would naturally add latency or make the system less resilient to glitches.
For this reason, the traditional way of executing asynchronous operations on the Web Platform, the event loop, does not work here, as the thread is not continuously executing. Additionally, a lot of unnecessary and potentially blocking operations are available from traditional execution contexts (Windows and Workers), which is not something that is desirable to reach an acceptable level of performance.
Additionally, the Worker model makes creating a dedicated thread
necessary for a script execution context, while all AudioNode
s
usually share the same execution context.
Note: This section specifies how the end result should look like, not how it should be implemented. In particular, instead of using message queue, implementors can use memory that is shared between threads, as long as the memory operations are not reordered.
2.2. Control Thread and Rendering Thread
The Web Audio API MUST be implemented using a control thread, and a rendering thread.
The control thread is the thread from which the AudioContext
is instantiated, and from which authors
manipulate the audio graph, that is, from where the operation on a BaseAudioContext
are invoked. The rendering thread is the thread on which the actual audio output is computed, in
reaction to the calls from the control thread. It can be a
real-time, callback-based audio thread, if computing audio for an AudioContext
, or a normal thread if computing audio for an OfflineAudioContext
.
The control thread uses a traditional event loop, as described in [HTML].
The rendering thread uses a specialized rendering loop, described in the section Rendering an audio graph
Communication from the control thread to the rendering thread is done using control message passing. Communication in the other direction is done using regular event loop tasks.
Each AudioContext
has a single control message
queue that is a list of control
messages that are operations running on the rendering
thread.
Queuing a control message means adding the message to the end of the control message queue of an BaseAudioContext
.
Note: For example, successfuly calling start()
on an AudioBufferSourceNode
source
adds a control
message to the control message
queue of the associated BaseAudioContext
.
Control messages in a control message queue are ordered by time of insertion. The oldest message is therefore the one at the front of the control message queue.
-
Let QC be a new, empty control message queue.
-
Move all the control messages QA to QC.
-
Move all the control messages QB to QA.
-
Move all the control messages QC to QB.
2.3. Asynchronous Operations
Calling methods on AudioNode
s is effectively asynchronous, and
MUST to be done in two phases: a synchronous part and an asynchronous
part. For each method, some part of the execution happens on the control thread (for example, throwing an exception in case of
invalid parameters), and some part happens on the rendering
thread (for example, changing the value of an AudioParam
).
In the description of each operation on AudioNode
s and BaseAudioContext
s, the synchronous section is marked with a ⌛. All
the other operations are executed in parallel, as described in [HTML].
The synchronous section is executed on the control thread, and happens immediately. If it fails, the method execution is aborted, possibly throwing an exception. If it succeeds, a control message, encoding the operation to be executed on the rendering thread is enqueued on the control message queue of this rendering thread.
The synchronous and asynchronous sections order with respect to other events MUST be the same: given two operation A and B with respective synchronous and asynchronous section ASync and AAsync, and BSync and BAsync, if A happens before B, then ASync happens before BSync, and AAsync happens before BAsync. In other words, synchronous and asynchronous sections can’t be reordered.
2.4. Rendering an Audio Graph
BaseAudioContext
. The number of
sample-frames in a block is called render quantum size, and the block
itself is called a render quantum. Its default value is 128, and it can
be configured by setting renderSizeHint
.
Operations that happen atomically on a given thread can only be executed when no other atomic operation is running on another thread.
The algorithm for rendering a block of audio from a BaseAudioContext
G with a control message queue Q is comprised of multiple steps and explained in further detail
in the algorithm of rendering a graph.
AudioContext
rendering thread is driven by a system-level audio callback, that is periodically
at regular intevals. Each call has a system-level audio callback buffer
size, which is a varying number of sample-frames that needs to be
computed on time before the next system-level audio callback arrives.
A load value is computed for each system-level audio
callback, by dividing its execution duration by the system-level
audio callback buffer size divided by sampleRate
.
Ideally the load value is below 1.0, meaning that it took less time to render the audio that it took to play it out. An audio buffer underrun happens when this load value is greater than 1.0: the system could not render audio fast enough for real-time.
Note that the concepts of system-level audio callback and load
value do not apply to OfflineAudioContext
s.
AudioContext
rendering thread is
often running off a system-level audio callback, that executes in
an isochronous fashion.
An OfflineAudioContext
is not required to have a
system-level audio callback, but behaves as if it did with the
callback happening as soon as the previous callback is
finished.
The audio callback is also queued as a task in the control message queue. The UA MUST perform
the following algorithms to process render quanta to fulfill such task by
filling up the requested buffer size. Along with the control message
queue, each AudioContext
has a regular task
queue, called its associated task queue for tasks that are posted to the rendering thread from the control thread. An
additional microtask checkpoint is performed after processing a render quantum
to run any microtasks that might have been queued during the execution of the process
methods of AudioWorkletProcessor
.
All tasks posted from an AudioWorkletNode
are posted to the associated
task queue of its associated BaseAudioContext
.
-
Set the internal slot
[[current frame]]
of theBaseAudioContext
to 0. Also setcurrentTime
to 0.
-
Let render result be
false
. -
Process the control message queue.
-
Let Qrendering be an empty control message queue. Atomically swap Qrendering with the current control message queue.
-
While there are messages in Qrendering, execute the following steps:
-
Execute the asynchronous section of the oldest message of Qrendering.
-
Remove the oldest message of Qrendering.
-
-
-
Process the
BaseAudioContext
's associated task queue.-
Let task queue be the
BaseAudioContext
's associated task queue. -
Let task count be the number of tasks in the in task queue
-
While task count is not equal to 0, execute the following steps:
-
Let oldest task be the first runnable task in task queue, and remove it from task queue.
-
Set the rendering loop’s currently running task to oldest task.
-
Perform oldest task’s steps.
-
Set the rendering loop currently running task back to
null
. -
Decrement task count
-
Perform a microtask checkpoint.
-
-
-
Process a render quantum.
-
If the
[[rendering thread state]]
of theBaseAudioContext
is notrunning
, return false. -
Order the
AudioNode
s of theBaseAudioContext
to be processed.-
Let ordered node list be an empty list of
AudioNode
s andAudioListener
. It will contain an ordered list ofAudioNode
s and theAudioListener
when this ordering algorithm terminates. -
Let nodes be the set of all nodes created by this
BaseAudioContext
, and still alive. -
Add the
AudioListener
to nodes. -
Let cycle breakers be an empty set of
DelayNode
s. It will contain all theDelayNode
s that are part of a cycle. -
For each
AudioNode
node in nodes:-
If node is a
DelayNode
that is part of a cycle, add it to cycle breakers and remove it from nodes.
-
-
For each
DelayNode
delay in cycle breakers:-
Let delayWriter and delayReader respectively be a DelayWriter and a DelayReader, for delay. Add delayWriter and delayReader to nodes. Disconnect delay from all its input and outputs.
Note: This breaks the cycle: if a
DelayNode
is in a cycle, its two ends can be considered separately, because delay lines cannot be smaller than one render quantum when in a cycle.
-
-
If nodes contains cycles, mute all the
AudioNode
s that are part of this cycle, and remove them from nodes. -
Consider all elements in nodes to be unmarked. While there are unmarked elements in nodes:
-
Choose an element node in nodes.
-
Visit node.
Visiting a node means performing the following steps: -
-
Reverse the order of ordered node list.
-
-
Compute the value(s) of the
AudioListener
'sAudioParam
s for this block. -
For each
AudioNode
, in ordered node list:-
For each
AudioParam
of thisAudioNode
, execute these steps:-
If this
AudioParam
has anyAudioNode
connected to it, sum the buffers made available for reading by allAudioNode
connected to thisAudioParam
, down mix the resulting buffer down to a mono channel, and call this buffer the input AudioParam buffer. -
Compute the value(s) of this
AudioParam
for this block. -
Queue a control message to set the
[[current value]]
slot of thisAudioParam
according to § 1.6.3 Computation of Value.
-
-
If this
AudioNode
has anyAudioNode
s connected to its input, sum the buffers made available for reading by allAudioNode
s connected to thisAudioNode
. The resulting buffer is called the input buffer. Up or down-mix it to match if number of input channels of thisAudioNode
. -
If this
AudioNode
is a source node, compute a block of audio, and make it available for reading. -
If this
AudioNode
is anAudioWorkletNode
, execute these substeps:-
Let processor be the associated
AudioWorkletProcessor
instance ofAudioWorkletNode
. -
Let O be the ECMAScript object corresponding to processor.
-
Let processCallback be an uninitialized variable.
-
Let completion be an uninitialized variable.
-
Prepare to run script with the current settings object.
-
Let getResult be Get(O, "process").
-
If getResult is an abrupt completion, set completion to getResult and jump to the step labeled return.
-
Set processCallback to getResult.[[Value]].
-
If ! IsCallable(processCallback) is
false
, then:-
Set completion to new Completion {[[Type]]: throw, [[Value]]: a newly created TypeError object, [[Target]]: empty}.
-
Jump to the step labeled return.
-
-
Set
[[callable process]]
totrue
. -
Perform the following substeps:
-
Let args be a Web IDL arguments list consisting of
inputs
,outputs
, andparameters
. -
Let esArgs be the result of converting args to an ECMAScript arguments list.
-
Let callResult be the Call(processCallback, O, esArgs). This operation computes a block of audio with esArgs. Upon a successful function call, a buffer containing copies of the elements of the
Float32Array
s passed via theoutputs
is made available for reading. AnyPromise
resolved within this call will be queued into the microtask queue in theAudioWorkletGlobalScope
. -
If callResult is an abrupt completion, set completion to callResult and jump to the step labeled return.
-
Set processor’s active source flag to ToBoolean(callResult.[[Value]]).
-
-
Return: at this point completion will be set to an ECMAScript completion value.
-
Clean up after running a callback with the current settings object.
-
Clean up after running script with the current settings object.
-
If completion is an abrupt completion:
-
Set
[[callable process]]
tofalse
. -
Set processor’s active source flag to
false
. -
Queue a task to the control thread fire an
ErrorEvent
namedprocessorerror
at the associatedAudioWorkletNode
.
-
-
-
-
If this
AudioNode
is a destination node, record the input of thisAudioNode
. -
Else, process the input buffer, and make available for reading the resulting buffer.
-
-
Atomically perform the following steps:
-
Increment
[[current frame]]
by the render quantum size. -
Set
currentTime
to[[current frame]]
divided bysampleRate
.
-
-
Set render result to
true
.
-
-
Return render result.
Muting an AudioNode
means that its
output MUST be silence for the rendering of this audio block.
Making a buffer available for reading from an AudioNode
means putting it in a state where other AudioNode
s connected to this AudioNode
can safely
read from it.
Note: For example, implementations can choose to allocate a new buffer, or have a more elaborate mechanism, reusing an existing buffer that is now unused.
Recording the input of an AudioNode
means copying the input data of this AudioNode
for future
usage.
Computing a block of audio means
AudioNode
to produce 128
sample-frames. AudioNode
to produce [[render quantum size]]
sample-frames.
Processing an input buffer means
running the algorithm for an AudioNode
, using an input
buffer and the value(s) of the AudioParam
(s) of this AudioNode
as the input for this algorithm.
2.5. Unloading a document
Additional unloading document cleanup steps are defined for documents that useBaseAudioContext
:
-
Reject all the promises of
[[pending promises]]
withInvalidStateError
, for eachAudioContext
andOfflineAudioContext
whose relevant global object is the same as the document’s associated Window. -
Stop all
decoding thread
s. -
Queue a control message to
close()
theAudioContext
orOfflineAudioContext
.
3. Dynamic Lifetime
3.1. Background
Note: The normative description of AudioContext
and AudioNode
lifetime characteristics is
described by the AudioContext lifetime and AudioNode lifetime.
This section is non-normative.
In addition to allowing the creation of static routing configurations, it should also be possible to do custom effect routing on dynamically allocated voices which have a limited lifetime. For the purposes of this discussion, let’s call these short-lived voices "notes". Many audio applications incorporate the ideas of notes, examples being drum machines, sequencers, and 3D games with many one-shot sounds being triggered according to game play.
In a traditional software synthesizer, notes are dynamically allocated and released from a pool of available resources. The note is allocated when a MIDI note-on message is received. It is released when the note has finished playing either due to it having reached the end of its sample-data (if non-looping), it having reached a sustain phase of its envelope which is zero, or due to a MIDI note-off message putting it into the release phase of its envelope. In the MIDI note-off case, the note is not released immediately, but only when the release envelope phase has finished. At any given time, there can be a large number of notes playing but the set of notes is constantly changing as new notes are added into the routing graph, and old ones are released.
The audio system automatically deals with tearing-down the part of
the routing graph for individual "note" events. A "note" is
represented by an AudioBufferSourceNode
, which
can be directly connected to other processing nodes. When the note
has finished playing, the context will automatically release the
reference to the AudioBufferSourceNode
, which in
turn will release references to any nodes it is connected to, and so
on. The nodes will automatically get disconnected from the graph and
will be deleted when they have no more references. Nodes in the graph
which are long-lived and shared between dynamic voices can be managed
explicitly. Although it sounds complicated, this all happens
automatically with no extra handling required.
3.2. Example
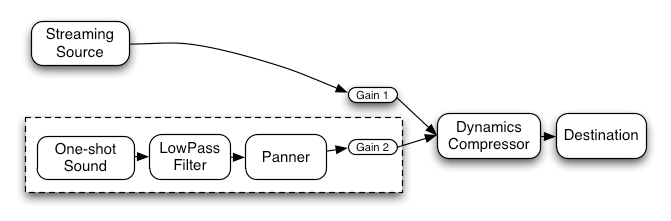
The low-pass filter, panner, and second gain nodes are directly connected from the one-shot sound. So when it has finished playing the context will automatically release them (everything within the dotted line). If there are no longer any references to the one-shot sound and connected nodes, then they will be immediately removed from the graph and deleted. The streaming source has a global reference and will remain connected until it is explicitly disconnected. Here’s how it might look in JavaScript:
let context= 0 ; let compressor= 0 ; let gainNode1= 0 ; let streamingAudioSource= 0 ; // Initial setup of the "long-lived" part of the routing graph function setupAudioContext() { context= new AudioContext(); compressor= context. createDynamicsCompressor(); gainNode1= context. createGain(); // Create a streaming audio source. const audioElement= document. getElementById( 'audioTagID' ); streamingAudioSource= context. createMediaElementSource( audioElement); streamingAudioSource. connect( gainNode1); gainNode1. connect( compressor); compressor. connect( context. destination); } // Later in response to some user action (typically mouse or key event) // a one-shot sound can be played. function playSound() { const oneShotSound= context. createBufferSource(); oneShotSound. buffer= dogBarkingBuffer; // Create a filter, panner, and gain node. const lowpass= context. createBiquadFilter(); const panner= context. createPanner(); const gainNode2= context. createGain(); // Make connections oneShotSound. connect( lowpass); lowpass. connect( panner); panner. connect( gainNode2); gainNode2. connect( compressor); // Play 0.75 seconds from now (to play immediately pass in 0) oneShotSound. start( context. currentTime+ 0.75 ); }
4. Channel Up-Mixing and Down-Mixing
This section is normative.
An AudioNode input has mixing rules for combining the channels from all
of the connections to it. As a simple example, if an input is connected
from a mono output and a stereo output, then the mono connection will
usually be up-mixed to stereo and summed with the stereo connection.
But, of course, it’s important to define the exact mixing
rules for every input to every AudioNode
. The
default mixing rules for all of the inputs have been chosen so that
things "just work" without worrying too much about the details,
especially in the very common case of mono and stereo streams. Of
course, the rules can be changed for advanced use cases, especially
multi-channel.
To define some terms, up-mixing refers to the process of taking a stream with a smaller number of channels and converting it to a stream with a larger number of channels. down-mixing refers to the process of taking a stream with a larger number of channels and converting it to a stream with a smaller number of channels.
An AudioNode
input needs to mix all the outputs
connected to this input. As part of this process it computes an
internal value computedNumberOfChannels representing the actual
number of channels of the input at any given time.
AudioNode
, an implementation
MUST:
-
Compute computedNumberOfChannels.
-
For each connection to the input:
-
up-mix or down-mix the connection to computedNumberOfChannels according to the
ChannelInterpretation
value given by the node’schannelInterpretation
attribute. -
Mix it together with all of the other mixed streams (from other connections). This is a straight-forward summing together of each of the corresponding channels that have been up-mixed or down-mixed in step 1 for each connection.
-
4.1. Speaker Channel Layouts
When channelInterpretation
is
"speakers
" then the up-mixing and down-mixing is defined for specific channel layouts.
Mono (one channel), stereo (two channels), quad (four channels), and 5.1 (six channels) MUST be supported. Other channel layouts may be supported in future version of this specification.
4.2. Channel Ordering
Channel ordering is defined by the following table. Individual multichannel formats MAY not support all intermediate channels. Implementations MUST present the channels provided in the order defined below, skipping over those channels not present.
Order | Label | Mono | Stereo | Quad | 5.1 |
---|---|---|---|---|---|
0 | SPEAKER_FRONT_LEFT | 0 | 0 | 0 | 0 |
1 | SPEAKER_FRONT_RIGHT | 1 | 1 | 1 | |
2 | SPEAKER_FRONT_CENTER | 2 | |||
3 | SPEAKER_LOW_FREQUENCY | 3 | |||
4 | SPEAKER_BACK_LEFT | 2 | 4 | ||
5 | SPEAKER_BACK_RIGHT | 3 | 5 | ||
6 | SPEAKER_FRONT_LEFT_OF_CENTER | ||||
7 | SPEAKER_FRONT_RIGHT_OF_CENTER | ||||
8 | SPEAKER_BACK_CENTER | ||||
9 | SPEAKER_SIDE_LEFT | ||||
10 | SPEAKER_SIDE_RIGHT | ||||
11 | SPEAKER_TOP_CENTER | ||||
12 | SPEAKER_TOP_FRONT_LEFT | ||||
13 | SPEAKER_TOP_FRONT_CENTER | ||||
14 | SPEAKER_TOP_FRONT_RIGHT | ||||
15 | SPEAKER_TOP_BACK_LEFT | ||||
16 | SPEAKER_TOP_BACK_CENTER | ||||
17 | SPEAKER_TOP_BACK_RIGHT |
4.3. Implication of tail-time on input and output channel count
When an AudioNode
has a non-zero tail-time, and an output channel
count that depends on the input channels count, the AudioNode
's tail-time must be taken into account when the input channel count
changes.
When there is a decrease in input channel count, the change in output channel count MUST happen when the input that was received with greater channel count no longer affects the output.
When there is an increase in input channel count, the behavior depends on the AudioNode
type:
-
For a
DelayNode
or aDynamicsCompressorNode
, the number of output channels MUST increase when the input that was received with greater channel count begins to affect the output. -
For other
AudioNode
s that have a tail-time, the number of output channels MUST increase immediately.Note: For a
ConvolverNode
, this only applies to the case where the impulse response is mono. Otherwise, theConvolverNode
always outputs a stereo signal regardless of its input channel count.
Note: Intuitively, this allows not losing stereo information as part of processing: when multiple input render quanta of different channel count contribute to an output render quantum then the output render quantum’s channel count is a superset of the input channel count of the input render quantums.
4.4. Up Mixing Speaker Layouts
Mono up-mix: 1 -> 2 : up-mix from mono to stereo output.L = input; output.R = input; 1 -> 4 : up-mix from mono to quad output.L = input; output.R = input; output.SL = 0; output.SR = 0; 1 -> 5.1 : up-mix from mono to 5.1 output.L = 0; output.R = 0; output.C = input; // put in center channel output.LFE = 0; output.SL = 0; output.SR = 0; Stereo up-mix: 2 -> 4 : up-mix from stereo to quad output.L = input.L; output.R = input.R; output.SL = 0; output.SR = 0; 2 -> 5.1 : up-mix from stereo to 5.1 output.L = input.L; output.R = input.R; output.C = 0; output.LFE = 0; output.SL = 0; output.SR = 0; Quad up-mix: 4 -> 5.1 : up-mix from quad to 5.1 output.L = input.L; output.R = input.R; output.C = 0; output.LFE = 0; output.SL = input.SL; output.SR = input.SR;
4.5. Down Mixing Speaker Layouts
A down-mix will be necessary, for example, if processing 5.1 source material, but playing back stereo.
Mono down-mix: 2 -> 1 : stereo to mono output = 0.5 * (input.L + input.R); 4 -> 1 : quad to mono output = 0.25 * (input.L + input.R + input.SL + input.SR); 5.1 -> 1 : 5.1 to mono output = sqrt(0.5) * (input.L + input.R) + input.C + 0.5 * (input.SL + input.SR) Stereo down-mix: 4 -> 2 : quad to stereo output.L = 0.5 * (input.L + input.SL); output.R = 0.5 * (input.R + input.SR); 5.1 -> 2 : 5.1 to stereo output.L = L + sqrt(0.5) * (input.C + input.SL) output.R = R + sqrt(0.5) * (input.C + input.SR) Quad down-mix: 5.1 -> 4 : 5.1 to quad output.L = L + sqrt(0.5) * input.C output.R = R + sqrt(0.5) * input.C output.SL = input.SL output.SR = input.SR
4.6. Channel Rules Examples
// Set gain node to explicit 2-channels (stereo). gain. channelCount= 2 ; gain. channelCountMode= "explicit" ; gain. channelInterpretation= "speakers" ; // Set "hardware output" to 4-channels for DJ-app with two stereo output busses. context. destination. channelCount= 4 ; context. destination. channelCountMode= "explicit" ; context. destination. channelInterpretation= "discrete" ; // Set "hardware output" to 8-channels for custom multi-channel speaker array // with custom matrix mixing. context. destination. channelCount= 8 ; context. destination. channelCountMode= "explicit" ; context. destination. channelInterpretation= "discrete" ; // Set "hardware output" to 5.1 to play an HTMLAudioElement. context. destination. channelCount= 6 ; context. destination. channelCountMode= "explicit" ; context. destination. channelInterpretation= "speakers" ; // Explicitly down-mix to mono. gain. channelCount= 1 ; gain. channelCountMode= "explicit" ; gain. channelInterpretation= "speakers" ;
5. Audio Signal Values
5.1. Audio sample format
Linear pulse code modulation (linear PCM) describes a format where the audio values are sampled at a regular interval, and where the quantization levels between two successive values are linearly uniform.
Whenever signal values are exposed to script in this specification, they are in
linear 32-bit floating point pulse code modulation format (linear 32-bit float
PCM), often in the form of Float32Array
objects.
5.2. Rendering
The range of all audio signals at a destination node of any audio graph
is nominally [-1, 1]. The audio rendition of signal values outside this
range, or of the values NaN
, positive infinity or negative
infinity, is undefined by this specification.
6. Spatialization/Panning
6.1. Background
A common feature requirement for modern 3D games is the ability to dynamically spatialize and move multiple audio sources in 3D space. For example OpenAL has this ability.
Using a PannerNode
, an audio stream can be
spatialized or positioned in space relative to an AudioListener
. A BaseAudioContext
will contain a single AudioListener
. Both panners and listeners have a
position in 3D space using a right-handed cartesian coordinate
system. The units used in the coordinate system are not defined, and
do not need to be because the effects calculated with these
coordinates are independent/invariant of any particular units such as
meters or feet. PannerNode
objects (representing
the source stream) have an orientation vector representing
in which direction the sound is projecting. Additionally, they have a sound cone representing how directional the sound is. For
example, the sound could be omnidirectional, in which case it would
be heard anywhere regardless of its orientation, or it can be more
directional and heard only if it is facing the listener. AudioListener
objects (representing a person’s
ears) have forward and up vectors
representing in which direction the person is facing.
The coordinate system for spatialization is shown in the diagram
below, with the default values shown. The locations for the AudioListener
and PannerNode
are moved from the default
positions so we can see things better.
During rendering, the PannerNode
calculates an azimuth and elevation. These values are used
internally by the implementation in order to render the
spatialization effect. See the Panning Algorithm section for
details of how these values are used.
6.2. Azimuth and Elevation
The following algorithm MUST be used to calculate the azimuth and elevation for the PannerNode
. The implementation must appropriately account for whether the various AudioParam
s below are "a-rate
" or "k-rate
".
// Let |context| be a BaseAudioContext and let |panner| be a // PannerNode created in |context|. // Calculate the source-listener vector. const listener= context. listener; const sourcePosition= new Vec3( panner. positionX. value, panner. positionY. value, panner. positionZ. value); const listenerPosition= new Vec3( listener. positionX. value, listener. positionY. value, listener. positionZ. value); const sourceListener= sourcePosition. diff( listenerPosition). normalize(); if ( sourceListener. magnitude== 0 ) { // Handle degenerate case if source and listener are at the same point. azimuth= 0 ; elevation= 0 ; return ; } // Align axes. const listenerForward= new Vec3( listener. forwardX. value, listener. forwardY. value, listener. forwardZ. value); const listenerUp= new Vec3( listener. upX. value, listener. upY. value, listener. upZ. value); const listenerRight= listenerForward. cross( listenerUp); if ( listenerRight. magnitude== 0 ) { // Handle the case where listener’s 'up' and 'forward' vectors are linearly // dependent, in which case 'right' cannot be determined azimuth= 0 ; elevation= 0 ; return ; } // Determine a unit vector orthogonal to listener’s right, forward const listenerRightNorm= listenerRight. normalize(); const listenerForwardNorm= listenerForward. normalize(); const up= listenerRightNorm. cross( listenerForwardNorm); const upProjection= sourceListener. dot( up); const projectedSource= sourceListener. diff( up. scale( upProjection)). normalize(); azimuth= 180 * Math. acos( projectedSource. dot( listenerRightNorm)) / Math. PI; // Source in front or behind the listener. const frontBack= projectedSource. dot( listenerForwardNorm); if ( frontBack< 0 ) azimuth= 360 - azimuth; // Make azimuth relative to "forward" and not "right" listener vector. if (( azimuth>= 0 ) && ( azimuth<= 270 )) azimuth= 90 - azimuth; else azimuth= 450 - azimuth; elevation= 90 - 180 * Math. acos( sourceListener. dot( up)) / Math. PI; if ( elevation> 90 ) elevation= 180 - elevation; else if ( elevation< - 90 ) elevation= - 180 - elevation;
6.3. Panning Algorithm
Mono-to-stereo and stereo-to-stereo panning MUST be supported. Mono-to-stereo processing is used when all connections to the input are mono. Otherwise stereo-to-stereo processing is used.
6.3.1. PannerNode "equalpower" Panning
This is a simple and relatively inexpensive algorithm which
provides basic, but reasonable results. It is used for the PannerNode
when the panningModel
attribute is set to
"equalpower
", in which case the elevation value is ignored. This algorithm MUST be implemented using
the appropriate rate as specified by the automationRate
. If any of the PannerNode
's AudioParam
s or the AudioListener
's AudioParam
s are
"a-rate
", a-rate processing must be used.
-
For each sample to be computed by this
AudioNode
:-
Let azimuth be the value computed in the azimuth and elevation section.
-
The azimuth value is first contained to be within the range [-90, 90] according to:
// First, clamp azimuth to allowed range of [-180, 180]. azimuth= max( - 180 , azimuth); azimuth= min( 180 , azimuth); // Then wrap to range [-90, 90]. if ( azimuth< - 90 ) azimuth= - 180 - azimuth; else if ( azimuth> 90 ) azimuth= 180 - azimuth; -
A normalized value x is calculated from azimuth for a mono input as:
x
= ( azimuth+ 90 ) / 180 ; Or for a stereo input as:
if ( azimuth<= 0 ) { // -90 -> 0 // Transform the azimuth value from [-90, 0] degrees into the range [-90, 90]. x= ( azimuth+ 90 ) / 90 ; } else { // 0 -> 90 // Transform the azimuth value from [0, 90] degrees into the range [-90, 90]. x= azimuth/ 90 ; } -
Left and right gain values are calculated as:
gainL
= cos( x* Math. PI/ 2 ); gainR= sin( x* Math. PI/ 2 ); -
For mono input, the stereo output is calculated as:
outputL
= input* gainL; outputR= input* gainR; Else for stereo input, the output is calculated as:
if ( azimuth<= 0 ) { outputL= inputL+ inputR* gainL; outputR= inputR* gainR; } else { outputL= inputL* gainL; outputR= inputR+ inputL* gainR; } -
Apply the distance gain and cone gain where the computation of the distance is described in Distance Effects and the cone gain is described in Sound Cones:
let distance= distance(); let distanceGain= distanceModel( distance); let totalGain= coneGain() * distanceGain(); outputL= totalGain* outputL; outputR= totalGain* outputR;
-
6.3.2. PannerNode "HRTF" Panning (Stereo Only)
This requires a set of HRTF (Head-related Transfer Function) impulse responses recorded at a variety of azimuths and elevations. The implementation requires a highly optimized convolution function. It is somewhat more costly than "equalpower", but provides more perceptually spatialized sound.
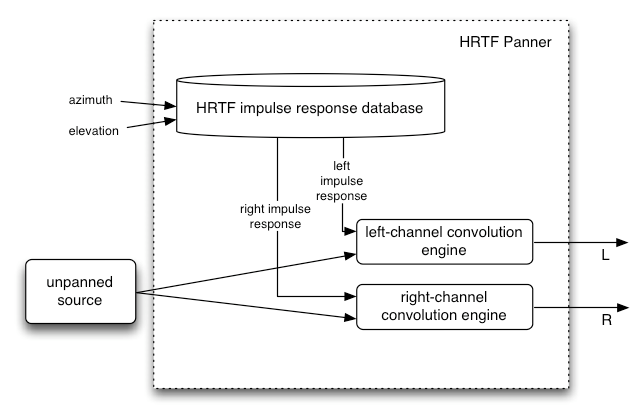
6.3.3. StereoPannerNode Panning
StereoPannerNode
, the following algorithm
MUST be implemented.
-
For each sample to be computed by this
AudioNode
-
Let pan be the computedValue of the
pan
AudioParam
of thisStereoPannerNode
. -
Clamp pan to [-1, 1].
pan
= max( - 1 , pan); pan= min( 1 , pan); -
Calculate x by normalizing pan value to [0, 1]. For mono input:
x
= ( pan+ 1 ) / 2 ; For stereo input:
if ( pan<= 0 ) x= pan+ 1 ; else x= pan; -
Left and right gain values are calculated as:
gainL
= cos( x* Math. PI/ 2 ); gainR= sin( x* Math. PI/ 2 ); -
For mono input, the stereo output is calculated as:
outputL
= input* gainL; outputR= input* gainR; Else for stereo input, the output is calculated as:
if ( pan<= 0 ) { outputL= inputL+ inputR* gainL; outputR= inputR* gainR; } else { outputL= inputL* gainL; outputR= inputR+ inputL* gainR; }
-
6.4. Distance Effects
Sounds which are closer are louder, while sounds further away are
quieter. Exactly how a sound’s volume changes according to
distance from the listener depends on the distanceModel
attribute.
During audio rendering, a distance value will be calculated based on the panner and listener positions according to:
function distance( panner) { const pannerPosition= new Vec3( panner. positionX. value, panner. positionY. value, panner. positionZ. value); const listener= context. listener; const listenerPosition= new Vec3( listener. positionX. value, listener. positionY. value, listener. positionZ. value); return pannerPosition. diff( listenerPosition). magnitude; }
distance will then be used to calculate distanceGain which depends on the distanceModel
attribute. See the DistanceModelType
section for details of how
this is calculated for each distance model.
As part of its processing, the PannerNode
scales/multiplies the input audio signal by distanceGain to
make distant sounds quieter and nearer ones louder.
6.5. Sound Cones
The listener and each sound source have an orientation vector describing which way they are facing. Each sound source’s sound projection characteristics are described by an inner and outer "cone" describing the sound intensity as a function of the source/listener angle from the source’s orientation vector. Thus, a sound source pointing directly at the listener will be louder than if it is pointed off-axis. Sound sources can also be omni-directional.
The following diagram ilustrates the relationship between the source’s
cone with respect to the listener. In the diagram,
and coneInnerAngle
= 50
. That
is, the inner cone extends 25 deg on each side of the direction
vector. Similarly, the outer cone is 60 deg on each side.coneOuterAngle
= 120
The following algorithm MUST be used to calculate the gain
contribution due to the cone effect, given the source (the PannerNode
) and the listener:
function coneGain() { const sourceOrientation= new Vec3( source. orientationX, source. orientationY, source. orientationZ); if ( sourceOrientation. magnitude== 0 || (( source. coneInnerAngle== 360 ) && ( source. coneOuterAngle== 360 ))) return 1 ; // no cone specified - unity gain // Normalized source-listener vector const sourcePosition= new Vec3( panner. positionX. value, panner. positionY. value, panner. positionZ. value); const listenerPosition= new Vec3( listener. positionX. value, listener. positionY. value, listener. positionZ. value); const sourceToListener= sourcePosition. diff( listenerPosition). normalize(); const normalizedSourceOrientation= sourceOrientation. normalize(); // Angle between the source orientation vector and the source-listener vector const angle= 180 * Math. acos( sourceToListener. dot( normalizedSourceOrientation)) / Math. PI; const absAngle= Math. abs( angle); // Divide by 2 here since API is entire angle (not half-angle) const absInnerAngle= Math. abs( source. coneInnerAngle) / 2 ; const absOuterAngle= Math. abs( source. coneOuterAngle) / 2 ; let gain= 1 ; if ( absAngle<= absInnerAngle) { // No attenuation gain= 1 ; } else if ( absAngle>= absOuterAngle) { // Max attenuation gain= source. coneOuterGain; } else { // Between inner and outer cones // inner -> outer, x goes from 0 -> 1 const x= ( absAngle- absInnerAngle) / ( absOuterAngle- absInnerAngle); gain= ( 1 - x) + source. coneOuterGain* x; } return gain; }
7. Performance Considerations
7.1. Latency
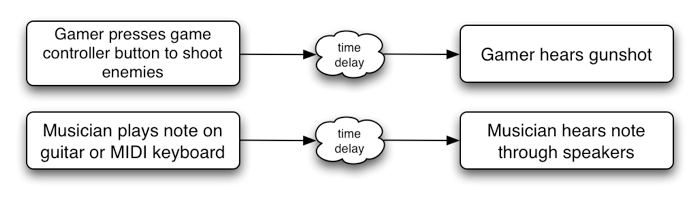
For web applications, the time delay between mouse and keyboard events (keydown, mousedown, etc.) and a sound being heard is important.
This time delay is called latency and is caused by several factors (input device latency, internal buffering latency, DSP processing latency, output device latency, distance of user’s ears from speakers, etc.), and is cumulative. The larger this latency is, the less satisfying the user’s experience is going to be. In the extreme, it can make musical production or game-play impossible. At moderate levels it can affect timing and give the impression of sounds lagging behind or the game being non-responsive. For musical applications the timing problems affect rhythm. For gaming, the timing problems affect precision of gameplay. For interactive applications, it generally cheapens the users experience much in the same way that very low animation frame-rates do. Depending on the application, a reasonable latency can be from as low as 3-6 milliseconds to 25-50 milliseconds.
Implementations will generally seek to minimize overall latency.
Along with minimizing overall latency, implementations will generally
seek to minimize the difference between an AudioContext
's currentTime
and an AudioProcessingEvent
's playbackTime
.
Deprecation of ScriptProcessorNode
will make this
consideration less important over time.
Additionally, some AudioNode
s can add latency to some paths
of the audio graph, notably:
-
The
AudioWorkletNode
can run a script that buffers internally, adding delay to the signal path. -
The
DelayNode
, whose role is to add controlled latency time. -
The
BiquadFilterNode
andIIRFilterNode
filter design can delay incoming samples, as a natural consequence of the causal filtering process. -
The
ConvolverNode
depending on the impulse, can delay incoming samples, as a natural result of the convolution operation. -
The
DynamicsCompressorNode
has a look ahead algorithm that causes delay in the signal path. -
The
MediaStreamAudioSourceNode
,MediaStreamTrackAudioSourceNode
andMediaStreamAudioDestinationNode
, depending on the implementation, can add buffers internally that add delays. -
The
ScriptProcessorNode
can have buffers between the control thread and the rendering thread. -
The
WaveShaperNode
, when oversampling, and depending on the oversampling technique, add delays to the signal path.
7.2. Audio Buffer Copying
When an acquire the content operation is performed on an AudioBuffer
, the entire operation
can usually be implemented without copying channel data. In
particular, the last step SHOULD be performed lazily at the next getChannelData()
call.
That means a sequence of consecutive acquire the contents operations with no
intervening getChannelData()
(e.g. multiple AudioBufferSourceNode
s playing the same AudioBuffer
) can be implemented with no
allocations or copying.
Implementations can perform an additional optimization: if getChannelData()
is called on an AudioBuffer
, fresh ArrayBuffer
s have not yet been
allocated, but all invokers of previous acquire the content operations on an AudioBuffer
have stopped using the AudioBuffer
's data,
the raw data buffers can be recycled for use with new AudioBuffer
s, avoiding any reallocation or copying of the
channel data.
7.3. AudioParam Transitions
While no automatic smoothing is done when directly setting the value
attribute of an AudioParam
, for certain parameters, smooth
transition are preferable to directly setting the value.
Using the setTargetAtTime()
method with a low timeConstant
allows authors to perform a smooth
transition.
7.4. Audio Glitching
Audio glitches are caused by an interruption of the normal continuous audio stream, resulting in loud clicks and pops. It is considered to be a catastrophic failure of a multi-media system and MUST be avoided. It can be caused by problems with the threads responsible for delivering the audio stream to the hardware, such as scheduling latencies caused by threads not having the proper priority and time-constraints. It can also be caused by the audio DSP trying to do more work than is possible in real-time given the CPU’s speed.
8. Security and Privacy Considerations
Per the Self-Review Questionnaire: Security and Privacy § questions:
-
Does this specification deal with personally-identifiable information?
It would be possible to perform a hearing test using Web Audio API, thus revealing the range of frequencies audible to a person (this decreases with age). It is difficult to see how this could be done without the realization and consent of the user, as it requires active particpation.
-
Does this specification deal with high-value data?
No. Credit card information and the like is not used in Web Audio. It is possible to use Web Audio to process or analyze voice data, which might be a privacy concern, but access to the user’s microphone is permission-based via
getUserMedia()
. -
Does this specification introduce new state for an origin that persists across browsing sessions?
No. AudioWorklet does not persist across browsing sessions.
-
Does this specification expose persistent, cross-origin state to the web?
Yes, the supported audio sample rate(s) and the output device channel count are exposed. See
AudioContext
. -
Does this specification expose any other data to an origin that it doesn’t currently have access to?
Yes. When giving various information on available
AudioNode
s, the Web Audio API potentially exposes information on characteristic features of the client (such as audio hardware sample-rate) to any page that makes use of theAudioNode
interface. Additionally, timing information can be collected through theAnalyserNode
orScriptProcessorNode
interface. The information could subsequently be used to create a fingerprint of the client.Research by Princeton CITP’s Web Transparency and Accountability Project has shown that
DynamicsCompressorNode
andOscillatorNode
can be used to gather entropy from a client to fingerprint a device. This is due to small, and normally inaudible, differences in DSP architecture, resampling strategies and rounding trade-offs between differing implementations. The precise compiler flags used and also the CPU architecture (ARM vs. x86) contribute to this entropy.In practice however, this merely allows deduction of information already readily available by easier means (User Agent string), such as "this is browser X running on platform Y". However, to reduce the possibility of additional fingerprinting, we mandate browsers take action to mitigate fingerprinting issues that might be possible from the output of any node.
Fingerprinting via clock skew has been described by Steven J Murdoch and Sebastian Zander. It might be possible to determine this from
getOutputTimestamp
. Skew-based fingerprinting has also been demonstrated by Nakibly et. al. for HTML. The High Resolution Time § 10. Privacy Considerations section should be consulted for further information on clock resolution and drift.Fingerprinting via latency is also possible; it might be possible to deduce this from
baseLatency
andoutputLatency
. Mitigation strategies include adding jitter (dithering) and quantization so that the exact skew is incorrectly reported. Note however that most audio systems aim for low latency, to synchronise the audio generated by WebAudio to other audio or video sources or to visual cues (for example in a game, or an audio recording or music making environment). Excessive latency decreases usability and may be an accessibility issue.Fingerprining via the sample rate of the
AudioContext
is also possible. We recommend the following steps to be taken to minimize this:-
44.1 kHz and 48 kHz are allowed as default rates; the system will choose between them for best applicability. (Obviously, if the audio device is natively 44.1, 44.1 will be chosen, etc., but also the system may choose the most "compatible" rate—e.g. if the system is natively 96kHz, 48kHz would likely be chosen, not 44.1kHz.
-
The system should resample to one of those two rates for devices that are natively at different rates, despite the fact that this may cause extra battery drain due to resampled audio. (Again, the system will choose the most compatible rate—e.g. if the native system is 16kHz, it’s expected that 48kHz would be chosen.)
-
It is expected (though not mandated) that browsers would offer a user affordance to force use of the native rate—e.g. by setting a flag in the browser on the device. This setting would not be exposed in the API.
-
It is also expected behavior that a different rate could be explicitly requested in the constructor for
AudioContext
(this is already in the specification; it normally causes the audio rendering to be done at the requested sampleRate, and then up- or down-sampled to the device output), and if that rate is natively supported, the rendering could be passed straight through. This would enable apps to render to higher rates without user intervention (although it’s not observable from Web Audio that the audio output is not downsampled on output)—for example, ifMediaDevices
capabilities were read (with user intervention) and indicated a higher rate was supported.
Fingerprinting via the number of output channels for the
AudioContext
is possible as well. We recommend thatmaxChannelCount
be set to two (stereo). Stereo is by far the most common number of channels. -
-
Does this specification enable new script execution/loading mechanisms?
No. It does use the [HTML] script execution method, defined in that specification.
-
Does this specification allow an origin access to a user’s location?
No.
-
Does this specification allow an origin access to sensors on a user’s device?
Not directly. Currently, audio input is not specified in this document, but it will involve gaining access to the client machine’s audio input or microphone. This will require asking the user for permission in an appropriate way, probably via the
getUserMedia()
API.Additionally, the security and privacy considerations from the Media Capture and Streams specification should be noted. In particular, analysis of ambient audio or playing unique audio may enable identification of user location down to the level of a room or even simultaneous occupation of a room by disparate users or devices. Access to both audio output and audio input might also enable communication between otherwise partitioned contexts in one browser.
-
Does this specification allow an origin access to aspects of a user’s local computing environment?
Not directly; all requested sample rates are supported, with upsampling if needed. It is possible to use Media Capture and Streams to probe for supported audio sample rates with MediaTrackSupportedConstraints. This requires explicit user consent. This does provide a small measure of fingerprinting. However, in practice most consumer and prosumer devices use one of two standardized sample rates: 44.1kHz (originally used by CD) and 48kHz (originally used by DAT). Highly resource constrained devices may support the speech-quality 11kHz sample rate, and higher-end devices often support 88.2, 96, or even the audiophile 192kHz rate.
Requiring all implementations to upsample to a single, commonly-supported rate such as 48kHz would increase CPU cost for no particular benefit, and requiring higher-end devices to use a lower rate would merely result in Web Audio being labelled as unsuitable for professional use.
-
Does this specification allow an origin access to other devices?
It typically does not allow access to other networked devices (an exception in a high-end recording studio might be Dante networked devices, although these typically use a separate, dedicated network). It does of necessity allow access to the user’s audio output device or devices, which are sometimes separate units to the computer.
For voice or sound-actuated devices, Web Audio API might be used to control other devices. In addition, if the sound-operated device is sensitive to near ultrasonic frequencies, such control might not be audible. This possibility also exists with HTML, through either the <audio> or <video> element. At common audio sampling rates, there is (by design) insufficient headroom for much ultrasonic information:
The limit of human hearing is usually stated as 20kHz. For a 44.1kHz sampling rate, the Nyquist limit is 22.05kHz. Given that a true brickwall filter cannot be physically realized, the space between 20kHz and 22.05kHz is used for a rapid rolloff filter to strongly attenuate all frequencies above Nyquist.
At 48kHz sampling rate, there is still rapid attenuation in the 20kHz to 24kHz band (but it is easier to avoid phase ripple errors in the passband).
-
Does this specification allow an origin some measure of control over a user agent’s native UI?
If the UI has audio components, such as a voice assistant or screenreader, Web Audio API might be used to emulate aspects of the native UI to make an attack seem more like a local system event. This possibility also exists with HTML, through the <audio> element.
-
Does this specification expose temporary identifiers to the web?
No.
-
Does this specification distinguish between behavior in first-party and third-party contexts?
No.
-
How should this specification work in the context of a user agent’s "incognito" mode?
Not differently.
-
Does this specification persist data to a user’s local device?
No.
-
Does this specification have a "Security Considerations" and "Privacy Considerations" section?
Yes (you are reading it).
-
Does this specification allow downgrading default security characteristics?
No.
9. Requirements and Use Cases
Please see [webaudio-usecases].
10. Common Definitions for Specification Code
This section describes common functions and classes employed by JavaScript code used within this specification.
// Three dimensional vector class. class Vec3{ // Construct from 3 coordinates. constructor ( x, y, z) { this . x= x; this . y= y; this . z= z; } // Dot product with another vector. dot( v) { return ( this . x* v. x) + ( this . y* v. y) + ( this . z* v. z); } // Cross product with another vector. cross( v) { return new Vec3(( this . y* v. z) - ( this . z* v. y), ( this . z* v. x) - ( this . x* v. z), ( this . x* v. y) - ( this . y* v. x)); } // Difference with another vector. diff( v) { return new Vec3( this . x- v. x, this . y- v. y, this . z- v. z); } // Get the magnitude of this vector. get magnitude() { return Math. sqrt( dot( this )); } // Get a copy of this vector multiplied by a scalar. scale( s) { return new Vec3( this . x* s, this . y* s, this . z* s); } // Get a normalized copy of this vector. normalize() { const m= magnitude; if ( m== 0 ) { return new Vec3( 0 , 0 , 0 ); } return scale( 1 / m); } }
11. Change Log
11.1. Changes since Recommendation of 17 Jun 2021
-
Issue 2450: Allow specifying a different render-quantum-size for an AudioContext or OfflineAudioContext
-
Issue 2512: Specify AnalyserNode treatment of non-fininte values.
-
Issue 2456: Add a MessagePort to the AudioWorkletGlobalScope
-
Issue 2444: Add AudioRenderCapacity interface and related classes
-
Issue 2361: Use new Web IDL buffer primitives
-
Issue 127: RangeError is thrown only for negative cancelTime
-
Issue 2359: Fix a typo in the VUMeterNode example
-
Issue 2373: Fix a typo: initially instead of initialy
-
Issue 2321: Warn about corrupted fils in decodeAudioData.
-
Issue 2375: decodeAudioData only decodes the first track of a multi-track audio file.
-
Issue 2475: Add definition for various concepts related to system-level audio callbacks
-
Issue 2400 : Access to a different output device: AudioContext.setSinkId()
-
11.2. Since Proposed Recommendation of 6 May 2021
-
Styling, status and boilerplate updates for Recommendation
-
Updated links to RFC2119, High-Resolution Time, and Audio EQ Cookbook
-
A spelling error was corrected
11.3. Since Candidate Recommendation of 14 January 2021
-
PR 2333: Update links to point to W3C versions
-
PR 2334: Use bikeshed to link to ErrorEvent
-
PR 2331: Add MIMESniff to normative references
-
PR 2328: MediaStream must be resampled to match the context sample rate
-
PR 2318: Restore empty of pending processor construction data after successful initialization of AudioWorkProcessor#port.
-
PR 2317: Standardize h3/h4 interface and dictionary markup
-
PR 2312: Rework description of control thread state and rendering thread state
-
PR 2311: Adjust the steps to process a context’s regular task queue
-
PR 2310: OscillatorNode output is mono
-
PR 2308: Refine phrasing for "allowed to start"
-
PR 2307: Replace "queue a task" with "queue a media element task"
-
PR 2306: Move some steps from AudioWorkletProcessor constructor to the instantiation algorithm
-
PR 2304: Add required components for ES operations in the rendering loop
-
PR 2301: Define when and how regular tasks are processed wrt the processing model
-
PR 2286: Clean up ABSN start algorithm
-
PR 2277: Fix compression curve diagram
-
PR 2273: Clarify units used in threshold & knee value calculations
-
PR 2256: ABSN extrapolates the last output
-
PR 2250: Use FrozenArray for AudioWorkletProcessor process()
-
PR 2298: Bikeshed HTML validation issues
11.4. Since Candidate Recommendation of 11 June 2020
-
PR 2202: Fixed wrong optionality of IIRFilterNode options
-
Issue 2191: Restrict sounds beyond normal hearing
-
PR 2210: Return rejected promise when the document is not fully active, for operations returning promises
-
Issue 2191: Destination of request created by
addModule
-
Issue 2213: The message queue is for message running on the rendering thread.
-
Issue 2216: Use inclusive language in the spec
-
PR 2219: Update more terminology in images and markdown documents
-
Issue 2206: PannerNode.rollOffFactor with "linear" distance model is not clamped to [0, 1] in main browser engines
-
Issue 2169: AudioParamDescriptor has member constraints that are redundant
-
Issue 1457: [privacy] Exposing data to an origin: fingerprinting
-
Issue 2061: Privacy re-review of latest changes
-
Issue 2225: Describe "Planar versus interleaved buffers"
-
Issue 2231: WaveShaper [[curve set]] not defined
-
Issue 2240: Align with Web IDL specification
-
Issue 2242: LInk to undefined instead of using
<code>
-
Issue 2227: Clarify buffer.copyToChannel() must be called before source.buffer = buffer else nothing is played
-
PR 2253: Fix duplicated IDs for decode callbacks
-
Issue 2252: When are promises in "[[pending resume promises]]" resolved?
-
PR 2266: Prohibit arbitrary termination of AudioWorkletGlobalScopes
11.5. Since Candidate Recommendation of 18 September 2018
-
Issue 2193: Incorrect azimuth comparison in spatialization algorithm
-
Issue 2192: Waveshaper curve interpolation algorithm incorrect
-
Issue 2171: Allow not having get parameterDescriptors in an AudioWorkletProcessor
-
Issue 2184: PannerNode refDistance description unclear
-
Issue 2165: AudioScheduledSourceNode start algorithm incomplete
-
Issue 2155: Restore changes accidentally reverted in bikeshed conversion
-
Issue 2154: Exception for changing channelCountMode on ScriptProcessorNode does not match browsers
-
Issue 2153: Exception for changing channelCount on ScriptProcessorNode does not match browsers
-
Issue 2152: close() steps don’t make sense
-
Issue 2150: AudioBufferOptions requires throwing NotFoundError in cases that can’t happen
-
Issue 2149: MediaStreamAudioSourceNode constructor has weird check for AudioContext
-
Issue 2148: IIRFilterOptions description makes impossible demands
-
Issue 2147: PeriodicWave constructor examines lengths of things that might not be there
-
Issue 2113: BiquadFilter gain lower bound can be lower.
-
Issue 2096: Lifetime of pending processor construction data and exceptions in instantiation of AudioWorkletProcessor
-
Issue 2087: Minor issues with BiquadFilter AudioParams
-
Issue 2083: Missing text in WaveShaperNode?
-
Issue 2082: WaveShaperNode curve interpolation incomplete
-
Issue 2074: Should the AudioWorkletNode constructor invoke the algorithm for initializing an object that inherits from AudioNode?
-
Issue 2073: Inconsistencies in constructor descriptions and factory method initialization
-
Issue 2072: Clarification on
AudioBufferSourceNode
looping, and loop points -
Issue 2071: cancelScheduledValues with setValueCurveAtTime
-
Issue 2060: Would it be helpful to restrict use of
AudioWorkletProcessor.port().postMessage()
in order to facilitate garbage collection? -
Issue 2051: Update to constructor operations
-
Issue 2050: Restore ConvolverNode channel mixing configurability (up to 2 channels)
-
Issue 2045: Should the check on
process()
be removed fromAudioWorkletGlobalScope.registerProcessor()
? -
Issue 2044: Remove
options
parameter fromAudioWorkletProcessor
constructor WebIDL -
Issue 2036: Remove
options
parameter ofAudioWorkletProcessor
constructor -
Issue 2035: De-duplicate initial value setting on AudioWorkletNode AudioParams
-
Issue 2027: Revise "processor construction data" algorithm
-
Issue 2021: AudioWorkletProcessor constructor leads to infinite recursion
-
Issue 2018: There are still issues with the setup of an AudioWorkletNode’s parameters
-
Issue 2016: Clarify
parameters
in AudioWorkletProcessor.process() -
Issue 2011: AudioWorkletNodeOptions.processorOptions should not default to null.
-
Issue 1989: Please update to Web IDL changes to optional dictionary defaulting
-
Issue 1984: Handling of exceptions in audio worklet is not very clear
-
Issue 1976: AudioWorkletProcessor’s [[node reference]] seems to be write-only
-
Issue 1972: parameterDescriptors handling during AudioWorkletNode initialization is probably wrong
-
Issue 1971: AudioWorkletNode options serialization is underdefined
-
Issue 1970: "active source" flag handling is a weird monkeypatch
-
Issue 1969: It would be clearer if the various validation of AudioWorkletNodeOptions were an explicit step or set of steps
-
Issue 1966: parameterDescriptors is not looked up by the AudioWorkletProcessor constructor
-
Issue 1963: NewTarget check for AudioWorkletProcessor isn’t actually possible with a Web IDL constructor
-
Issue 1947: Spec is inconsistent about whether parameterDescriptors is an array or an iterable
-
Issue 1946: Population of "node name to parameter descriptor map" needs to be defined
-
Issue 1945: registerProcessor is doing odd things with threads and JS values
-
Issue 1943: Describe how WaveShaperNode shapes the input with the curve
-
Issue 1935: length of AudioWorkletProcessor.process() parameter sequences with inactive inputs
-
Issue 1932: Make AudioWorkletNode output buffer available for reading
-
Issue 1925: front vs forward
-
Issue 1902: Mixer Gain Structure section not needed
-
Issue 1906: Steps in rendering algorithm
-
Issue 1905: Rendering callbacks are observable
-
Issue 1904: Strange Note in algorithm for swapping a control message queue
-
Issue 1903: Funny sentence about priority and latency
-
Issue 1901: AudioWorkletNode state property?
-
Issue 1900: AudioWorkletProcessor NewTarget undefined
-
Issue 1899: Missing synchronous markers
-
Issue 1897: WaveShaper curve value setter allows multiple sets
-
Issue 1896: WaveShaperNode constructor says curve set is initialized to false
-
Issue #1471: AudioNode Lifetime section seems to attempt to make garbage collection observable
-
Issue #1893: Active processing for Panner/Convolver/ChannelMerger
-
Issue #1894: Funny text in PannerNode.orientationX
-
Issue #1866: References to garbage collection
-
Issue #1851: Parameter values used for BiquadFilterNode::getFrequencyResponse
-
Issue #1905: Rendering callbacks are observable
-
Issue #1879: ABSN playback algorithm offset
-
Issue #1882: Biquad lowpass/highpass Q
-
Issue #1303: MediaElementAudioSourceNode information in a funny place
-
Issue #1896: WaveShaperNode constructor says curve set is initialized to false
-
Issue #1897: WaveShaper curve value setter allows multiple sets.
-
Issue #1880: setOrientation description has confusing paragraph
-
Issue #1855: createScriptProcessor parameter requirements
-
Issue #1857: Fix typos and bad phrasing
-
Issue #1788: Unclear what value is returned by AudioParam.value
-
Issue #1852: Fix error condition of AudioNode.disconnect(destinationNode, output, input)
-
Issue #1841: Recovering from unstable biquad filters?
-
Issue #1777: Picture of the coordinate system for panner node
-
Issue #1802: Clarify interaction between user-invoked suspend and autoplay policy
-
Issue #1822: OfflineAudioContext.suspend can suspend before the given time
-
Issue #1772: Sorting tracks alphabetically is underspecified
-
Issue #1797: Specification is incomplete for AudioNode.connect()
-
Issue #1805: Exception ordering on error
-
Issue #1790: Automation example chart has an error (reversed function arguments
-
Fix rendering algorithm iteration and cycle breaking
-
Issue #1719: channel count changes in filter nodes with tail time
-
Issue #1563: Make decodeAudioData more precise
-
Issue #1481: Tighten spec on ABSN output channels?
-
Issue #1762: Setting convolver buffer more than once?
-
Issue #1758: Explicitly include time-domain processing code for BiquadFilterNode
-
Issue #1770: Link to correct algorithm for StereoPannerNode, mention algorithm is equal-power
-
Issue #1753: Have a single
AudioWorkletGlobalScope
perBaseAudioContext
-
Issue #1746: AnalyserNode: Clarify how much time domain data we’re supposed to keep around
-
Issue #1741: Sample rate of AudioBuffer
-
Issue #1745: Clarify unit of fftSize
-
Issue #1743: Missing normative reference to Fetch
-
Use "get a reference to the bytes" algorithm as needed.
-
Specify rules for determining output chanel count.
-
Clarified rendering algorithm for AudioListener.
11.6. Since Working Draft of 19 June 2018
-
Minor editorial clarifications.
-
Update implementation-report.html.
-
Widen the valid range of detune values so that any value that doesn’t cause 2^(d/1200) to overflow is valid.
-
PannerNode constructor throws errors.
-
Rephrase algorithm for setting buffer and curve.
-
Refine startRendering algorithm.
-
Make "queue a task" link to the HTML spec.
-
Specify more precisely, events overlapping with SetValueCurveAtTime.
-
Add implementation report to gh-pages.
-
Honor the given value in
outputChannelCount
. -
Initialize bufferDuration outside of process() in ABSN algorithm.
-
Rework definition of ABSN output behavior to account for playbackRate’s interaction with the start(…duration) argument.
-
Add mention of video element in ultrasonic attack surface.
11.7. Since Working Draft of 08 December 2015
-
Add AudioWorklet and related interfaces to support custom nodes. This replaces ScriptProcessorNode, which is now deprecated.
-
Explicitly say what the channel count, mode, and interpretation values are for all source nodes.
-
Specify the behavior of Web Audio when a document is unloaded.
-
Merge the proposed SpatialListener interface into AudioListener.
-
Rework and clean up algorithms for panning and spatialization and define "magic functions".
-
Clarify that AudioBufferSourceNode looping is limited by duration argument to start().
-
Add constructors with options dictionaries for all node types.
-
Clarify parameter automation method behavior and equations. Handle cases where automation methods may interact with each other.
-
Support latency hints and arbitrary sample rates in AudioContext constructor.
-
Clear up ambiguities in definitions of start() and stop() for scheduled sources.
-
Remove automatic dezippering from AudioParam value setters which now equate to setValueAtTime().
-
Specify normative behavior of DynamicsCompressorNode.
-
Specify that AudioParam.value returns the most recent computed value.
-
Permit AudioBufferSourceNode to specify sub-sample start, duration, loopStart and loopEnd. Respecify algorithms to say exactly how looping works in all scenarios, including dynamic and negative playback rates.
-
Harmonized behavior of IIRFilterNode with BiquadFilterNode.
-
Add diagram describing mono-input-to-matrixed-stereo case.
-
Prevent connecting an AudioNode to an AudioParam of a different AudioContext.
-
Added Audioparam cancelAndHoldAtTime
-
Clarify behaviour of AudioParam.cancelScheduledValues().
-
Add playing reference to MediaElementAudioSourceNodes and MediaStreamAudioSourceNodes.
-
Refactor BaseAudioContext interface out of AudioContext, OfflineAudioContext.
-
OfflineAudioContext inherits from BaseAudioContext, not AudioContext.
-
"StereoPanner" replaced with the correct "StereoPannerNode".
-
Support chaining on AudioNode.connect() and AudioParam automation methods.
-
Specify behavior of events following SetTarget events.
-
Reinstate channelCount declaration for AnalyserNode.
-
Specify exponential ramp behavior when previous value is 0.
-
Specify behavior of setValueCurveAtTime parameters.
-
Add spatialListener attribute to AudioContext.
-
Remove section titled "Doppler Shift".
-
Added a list of nodes and reason why they can add latency, in an informative section.
-
Speced nominal ranges, nyquist, and behavior when outside the range.
-
Spec the processing model for the Web Audio API.
-
Merge the SpatialPannerNode into the PannerNode, undeprecating the PannerNode.
-
Merge the SpatialListener into the AudioListener, undeprecating the AudioListener.
-
Added latencyHint(s).
-
Move the constructor from BaseAudioContext to AudioContext where it belongs; BaseAudioContext is not constructible.
-
Specified the Behavior of automations and nominal ranges.
-
The playbackRate is widened to +/- infinity.
-
setValueCurveAtTime is modified so that an implicit call to setValueAtTime is made at the end of the curve duration.
-
Make setting the
value
attribute of anAudioParam
strictly equivalent of calling setValueAtTime with AudioContext.currentTime. -
Add new sections for AudioContextOptions and AudioTimestamp.
-
Add constructor for all nodes.
-
Define ConstantSourceNode.
-
Make the WaveShaperNode have a tail time, depending on the oversampling level.
-
Allow collecting MediaStreamAudioSourceNode or MediaElementAudioSourceNode when they won’t play ever again.
-
Add a concept of 'allowed to start' and use it when creating an AudioContext and resuming it from resume() (closes #836).
-
Add AudioScheduledSourceNode base class for source nodes.
-
Mark all AudioParams as being k-rate.
12. Acknowledgements
This specification is the collective work of the W3C Audio Working Group.
Members and former members of the Working Group and contributors to the specification are (at the time of writing, and by
alphabetical order):
Adenot, Paul (Mozilla Foundation) - Specification Co-editor;
Akhgari, Ehsan (Mozilla Foundation);
Becker, Steven (Microsoft Corporation);
Berkovitz, Joe (Invited Expert, affiliated with Noteflight/Hal Leonard) - WG co-chair from September 2013 to December 2017);
Bossart, Pierre (Intel Corporation);
Borins, Myles (Google, Inc);
Buffa, Michel (NSAU);
Caceres, Marcos (Invited Expert);
Cardoso, Gabriel (INRIA);
Carlson, Eric (Apple, Inc);
Chen, Bin (Baidu, Inc);
Choi, Hongchan (Google, Inc) - Specification Co-editor;
Collichio, Lisa (Qualcomm);
Geelnard, Marcus (Opera Software);
Gehring, Todd (Dolby Laboratories);
Goode, Adam (Google, Inc);
Gregan, Matthew (Mozilla Foundation);
Hikawa, Kazuo (AMEI);
Hofmann, Bill (Dolby Laboratories);
Jägenstedt, Philip (Google, Inc);
Jeong, Paul Changjin (HTML5 Converged Technology Forum);
Kalliokoski, Jussi (Invited Expert);
Lee, WonSuk (Electronics and Telecommunications Research Institute);
Kakishita, Masahiro (AMEI);
Kawai, Ryoya (AMEI);
Kostiainen, Anssi (Intel Corporation);
Lilley, Chris (W3C Staff);
Lowis, Chris (Invited Expert) - WG co-chair from December 2012 to September 2013, affiliated with British Broadcasting Corporation;
MacDonald, Alistair (W3C Invited Experts) — WG co-chair from March 2011 to July 2012;
Mandyam, Giridhar (Qualcomm Innovation Center, Inc);
Michel, Thierry (W3C/ERCIM);
Nair, Varun (Facebook);
Needham, Chris (British Broadcasting Corporation);
Noble, Jer (Apple, Inc);
O’Callahan, Robert(Mozilla Foundation);
Onumonu, Anthony (British Broadcasting Corporation);
Paradis, Matthew (British Broadcasting Corporation) - WG co-chair from September 2013 to present;
Pozdnyakov, Mikhail (Intel Corporation);
Raman, T.V. (Google, Inc);
Rogers, Chris (Google, Inc);
Schepers, Doug (W3C/MIT);
Schmitz, Alexander (JS Foundation);
Shires, Glen (Google, Inc);
Smith, Jerry (Microsoft Corporation);
Smith, Michael (W3C/Keio);
Thereaux, Olivier (British Broadcasting Corporation);
Toy, Raymond (Google, Inc.) - WG co-chair from December 2017 - Present;
Toyoshima, Takashi (Google, Inc);
Troncy, Raphael (Institut Telecom);
Verdie, Jean-Charles (MStar Semiconductor, Inc.);
Wei, James (Intel Corporation);
Weitnauer, Michael (IRT);
Wilson, Chris (Google,Inc);
Zergaoui, Mohamed (INNOVIMAX)